Top Ruby Methods Every Developer Should Know
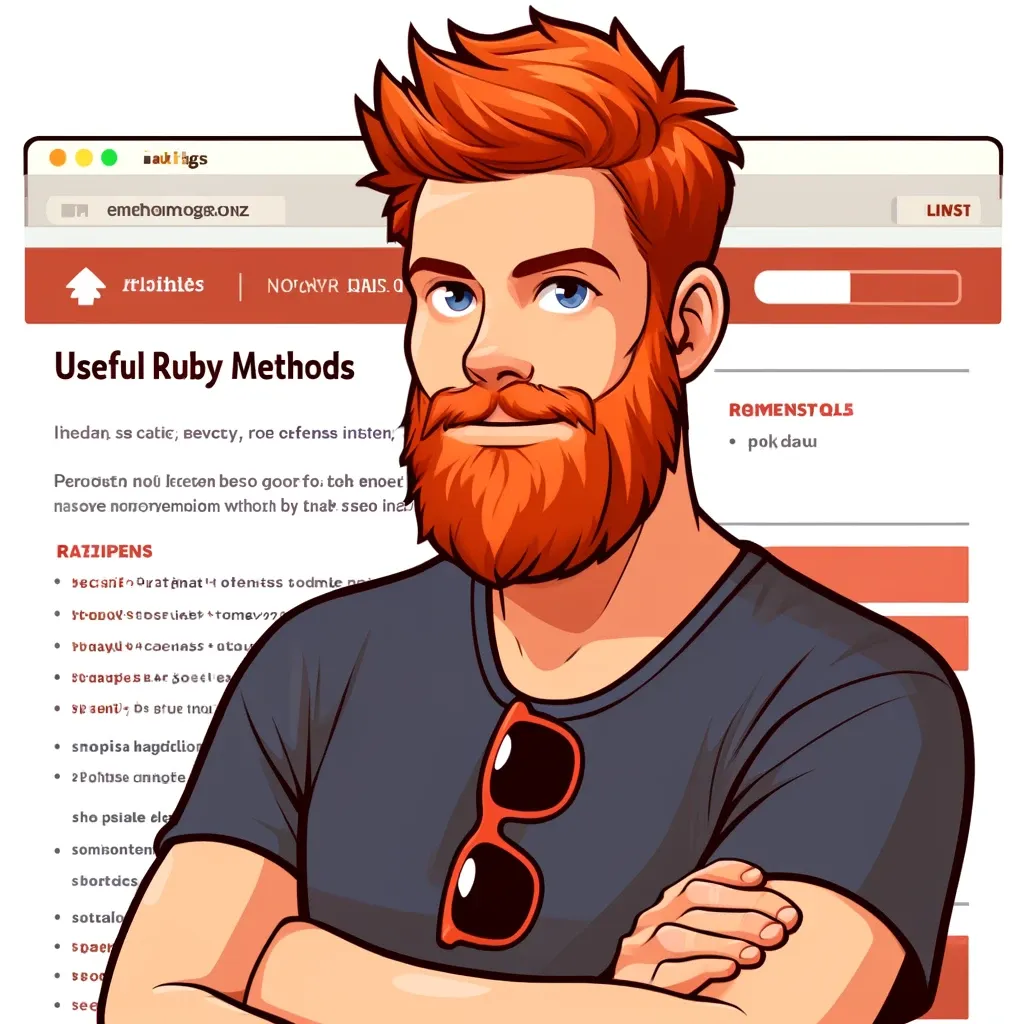
Ruby is a dynamic and expressive programming language known for its simplicity and elegance. It offers a rich set of built-in methods that make coding in Ruby a pleasure. In this blog post, we'll explore some of the most popular and useful Ruby methods that every developer should know.
1. each
The each
method is used to iterate over elements in an array, hash, or range. It is a foundational method for working with collections in Ruby.
numbers = [1, 2, 3, 4, 5]
numbers.each do |number|
puts number
end
####################################
########### Results ################
####################################
1
2
3
4
5
2. map
The map
method transforms each element in a collection based on the block's return value. It returns a new array with the transformed elements.
numbers = [1, 2, 3, 4, 5]
squares = numbers.map { |number| number ** 2 }
puts squares
####################################
########### Results ################
####################################
[1, 4, 9, 16, 25]
3. select
The select
method returns a new array containing only the elements for which the block returns true
. It's a great way to filter collections.
numbers = [1, 2, 3, 4, 5]
even_numbers = numbers.select { |number| number.even? }
puts even_numbers
####################################
########### Results ################
####################################
[2, 4]
4. reduce
The reduce
method combines all elements in a collection into a single value based on the block's logic. It's useful for aggregating data.
numbers = [1, 2, 3, 4, 5]
sum = numbers.reduce(0) { |acc, number| acc + number }
puts sum
####################################
########### Results ################
####################################
15
5. sort
The sort
method returns a new array with elements sorted according to a block or the default comparison.
numbers = [5, 3, 1, 4, 2]
sorted_numbers = numbers.sort
puts sorted_numbers
####################################
########### Results ################
####################################
[1, 2, 3, 4, 5]
6. uniq
The uniq
method removes duplicate elements from an array, returning a new array with unique values.
numbers = [1, 2, 2, 3, 3, 4, 5]
unique_numbers = numbers.uniq
puts unique_numbers
####################################
########### Results ################
####################################
[1, 2, 3, 4, 5]
7. zip
The zip
method combines elements from multiple arrays into a single array of pairs. It's useful for merging related data.
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
name_age_pairs = names.zip(ages)
puts name_age_pairs.inspect
####################################
########### Results ################
####################################
[('Alice', 25), ('Bob', 30), ('Charlie', 35)]
8. flatten
The flatten
method converts nested arrays into a single array, making it easier to work with deeply nested data structures.
nested_array = [[1, 2], [3, 4], [5]]
flattened_array = nested_array.flatten
puts flattened_array
####################################
########### Results ################
####################################
[1, 2, 3, 4, 5]
Conclusion
These methods represent just a fraction of Ruby's rich collection of built-in functionalities. By mastering these methods, you'll become more proficient in writing clean, efficient, and expressive Ruby code. Whether you're building web applications with Ruby on Rails or working on a simple script, these methods will be invaluable in your development toolkit.