PHP Fundamentals: An Introduction to PHP
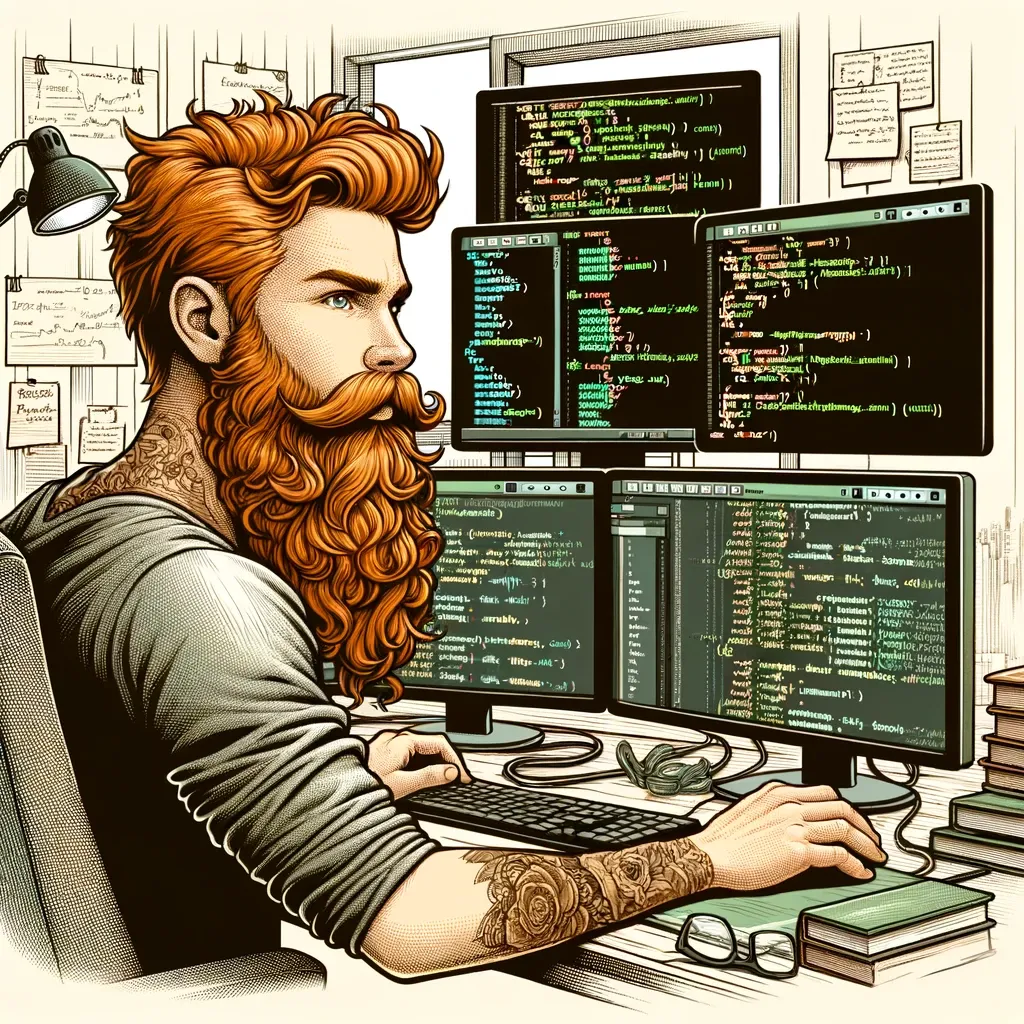
PHP (PHP: Hypertext Preprocessor) is a server-side scripting language designed for web development but also used as a general-purpose programming language. It enables developers to create dynamic content that interacts with databases. PHP is notable for its ease of use, extensive feature set, and strong community support.
PHP Fundamentals and Examples
Syntax
Basic syntax involves PHP tags, comments, and standard HTML integration.
<?php
// This is a single-line comment
echo "Hello, World!"; // Outputs: Hello, World!
?>
Variables
Variables in PHP are declared with a $ sign followed by the variable name.
<?php
$name = "John";
$age = 25;
?>
Data Types
PHP supports several data types including strings, integers, floats, booleans, arrays, and objects.
<?php
$string = "Hello";
$integer = 10;
$float = 10.5;
$boolean = true;
?>
Strings
Operations on strings, such as concatenation and various string functions.
<?php
$greeting = "Hello";
$name = "Alice";
echo $greeting . " " . $name; // Outputs: Hello Alice
?>
Arrays
PHP allows indexed, associative, and multi-dimensional arrays.
<?php
$colors = array("red", "green", "blue");
echo $colors[0]; // Outputs: red
$age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43");
echo $age['Ben']; // Outputs: 37
?>
Control Structures
Includes if-else statements, switch cases, and loops (for, while, do-while, foreach).
<?php
for ($x = 0; $x <= 10; $x++) {
echo "The number is: $x <br>";
}
?>
Functions
Defining and calling functions.
<?php
function familyName($fname) {
echo "$fname Refsnes.<br>";
}
familyName("Jani");
?>
Superglobals
PHP superglobals are built-in variables that are always accessible.
<?php
echo $_SERVER['HTTP_USER_AGENT'];
?>
Form Handling
Handling data from forms using POST and GET methods.
<?php
// "welcome.php"
echo "Welcome " . $_POST['name'];
?>
File Inclusion
Including and requiring files in scripts.
<?php
include 'header.php';
require 'functions.php';
?>
File Handling
Reading and writing files.
<?php
$file = fopen("testfile.txt", "w");
$txt = "Hello World";
fwrite($file, $txt);
fclose($file);
?>
Sessions and Cookies
Managing sessions and cookies for maintaining state.
<?php
session_start();
$_SESSION['favcolor'] = 'green';
?>
Error Handling
Custom error handling and exceptions.
<?php
function customError($errno, $errstr) {
echo "<b>Error:</b> [$errno] $errstr";
}
set_error_handler("customError");
?>
Database Access
Connecting to databases like MySQL.
<?php
$conn = new mysqli($servername, $username, $password, $dbname);
?>
Regular Expressions
Utilizing regular expressions for pattern matching.
<?php
$str = "Visit W3Schools";
$pattern = "/w3schools/i";
echo preg_match($pattern, $str); // Outputs: 1
?>
Object-Oriented Programming
PHP supports OOP concepts such as classes, objects, inheritance, etc.
<?php
class Car {
function Car() {
$this->model = "VW";
}
}
$herbie = new Car();
echo $herbie->model;
?>
Conclusion
Mastering these fundamentals will significantly improve your ability to develop robust PHP applications and enhance your overall understanding of server-side programming.