The Power of SCSS: A Basic Guide
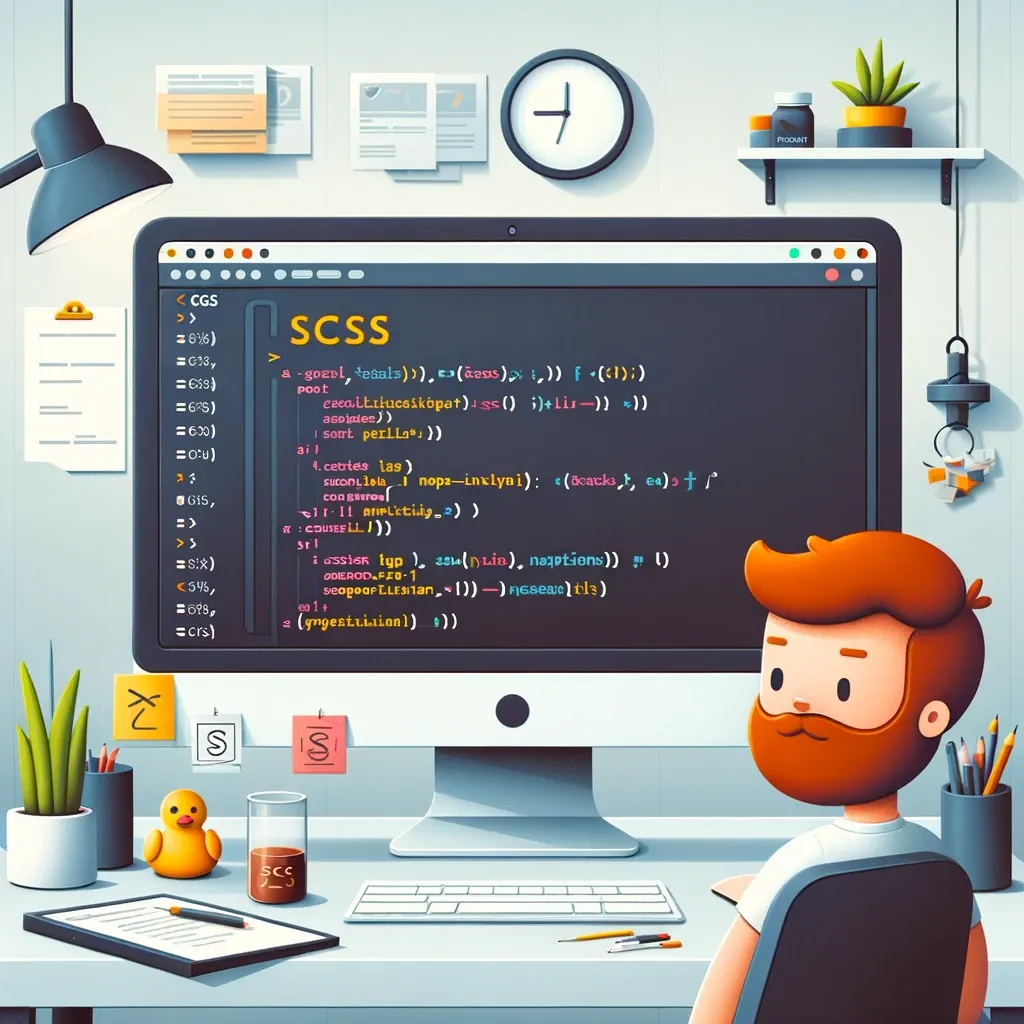
Introduction to SCSS
In the ever-evolving world of web development, CSS plays a pivotal role in determining the visual appeal of a website. However, traditional CSS has limitations, especially when projects scale. This is where SCSS (Sassy CSS) comes into play, offering a more robust and flexible approach to styling.
SCSS is a preprocessor for CSS, allowing developers to write more maintainable and modular stylesheets. It is a superset of CSS, meaning that any valid CSS code is also valid SCSS code. This article delves into the core features of SCSS, its advantages, and practical examples to help you leverage its power in your projects.
SCSS Basics
SCSS, part of the Sass (Syntactically Awesome Style Sheets) family, introduces additional features to CSS, making it more developer-friendly. Some of the key features include:
- Variables: SCSS allows you to define variables for colors, fonts, dimensions, etc. This feature is incredibly useful for maintaining consistency across a project.
- Nesting: Instead of writing repetitive selectors, SCSS allows you to nest styles, reflecting the HTML structure. This makes the code more readable and easier to maintain.
- Mixins: These are reusable blocks of code that can be included in multiple places, reducing redundancy.
- Inheritance (Extend/Placeholder Selectors): With SCSS, you can extend existing styles, promoting code reuse and reducing repetition.
- Partials and Import Statements: SCSS lets you split your stylesheets into smaller, manageable files, which can be imported into a main file.
- Operators and Functions: SCSS provides a variety of operators and functions for arithmetic operations, color manipulation, and more.
Let's dive into each of these features in more detail.
Variables in SCSS
Variables are a core feature of SCSS, allowing you to store values and reuse them throughout your stylesheet. This is especially useful when you have consistent elements like colors, font sizes, and spacing. Here's an example of how variables work in SCSS:
$primary-color: #3498db;
$secondary-color: #2ecc71;
$font-size: 16px;
body {
color: $primary-color;
font-size: $font-size;
}
h1 {
color: $secondary-color;
}
In this example, we've defined a primary color, a secondary color, and a font size. These variables are then used to style the body and heading elements. If you need to change the primary color, you can do so in one place, and it will update across the entire stylesheet.
Nesting in SCSS
Nesting allows you to structure your stylesheets in a way that reflects the HTML structure. This makes the code easier to read and reduces redundancy. Here's an example of nesting in SCSS:
.navbar {
background-color: $primary-color;
.nav-item {
color: white;
&:hover {
color: $secondary-color;
}
}
}
In this example, we have a navbar
class containing nested styles for .nav-item
. The &
symbol is used to reference the parent selector, allowing you to create hover effects and other pseudo-classes easily.
Mixins in SCSS
Mixins are reusable blocks of code that can be included in multiple places. This reduces redundancy and makes your code more maintainable. Here's an example of a simple mixin:
@mixin flex-center {
display: flex;
justify-content: center;
align-items: center;
}
.container {
@include flex-center;
height: 100vh;
}
In this example, the flex-center
mixin is defined to center content using flexbox. The container
class includes this mixin to center its content. Mixins can also accept parameters, allowing for more flexibility.
Inheritance in SCSS
Inheritance allows you to extend existing styles, reducing code duplication. This is done using the @extend
keyword or placeholder selectors. Here's an example:
%base-button {
padding: 10px 20px;
border: none;
cursor: pointer;
}
.primary-button {
@extend %base-button;
background-color: $primary-color;
}
.secondary-button {
@extend %base-button;
background-color: $secondary-color;
}
In this example, we've defined a placeholder selector %base-button
with common button styles. The .primary-button
and .secondary-button
classes extend this base style, allowing you to create consistent button designs.
Partials and Import Statements in SCSS
Partials and import statements allow you to split your stylesheets into smaller files for better organization. Partials are prefixed with an underscore, indicating that they are not compiled into separate CSS files. Here's an example of how you might use partials and import statements:
// _variables.scss
$primary-color: #3498db;
// _mixins.scss
@mixin box-shadow {
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
// main.scss
@import 'variables';
@import 'mixins';
.container {
background-color: $primary-color;
@include box-shadow;
}
In this example, we've created two partials: _variables.scss
and _mixins.scss
. The main.scss
file imports these partials and uses the defined variables and mixins.
Operators and Functions in SCSS
SCSS provides various operators and functions for arithmetic operations and color manipulation. This feature allows you to create dynamic styles. Here's an example:
$base-spacing: 8px;
.container {
padding: $base-spacing * 2;
}
$base-color: #3498db;
.button {
background-color: lighten($base-color, 10%);
&:hover {
background-color: darken($base-color, 10%);
}
}
In this example, we use operators to double the base spacing for padding. The lighten
and darken
functions are used to manipulate colors for hover effects.
Advanced SCSS Techniques
SCSS offers additional advanced techniques that can further enhance your workflow and code quality. Let's explore some of these techniques:
Control Directives
SCSS provides control directives that allow you to write conditional statements and loops within your stylesheets. This feature adds a level of programmability to your CSS. Here's an example of a control directive using the @if
statement:
$theme: 'dark';
body {
@if $theme == 'dark' {
background-color: #333;
color: #f1f1f1;
} @else {
background-color: #f1f1f1;
color: #333;
}
}
In this example, we use the @if
statement to apply different styles based on a condition. This allows for more dynamic stylesheets, adaptable to different scenarios.
Loops in SCSS
Loops in SCSS allow you to generate repetitive code with ease. This feature is especially useful for creating grid systems and other complex layouts. Here's an example of a loop using the @for
directive:
$columns: 12;
@for $i from 1 through $columns {
.col-#{$i} {
width: percentage($i / $columns);
}
}
In this example, we create a basic grid system with 12 columns. The loop generates the column classes dynamically based on the specified range.
Functions in SCSS
SCSS functions are custom functions that can be used to create reusable logic. This is different from mixins, as functions return a value rather than generating CSS code. Here's an example of a custom function:
@function px-to-rem($px) {
$base-font-size: 16px;
@return $px / $base-font-size * 1rem;
}
.container {
padding: px-to-rem(16px);
}
In this example, we create a custom function px-to-rem
to convert pixel values to rem. This allows for more flexible and responsive design, especially when dealing with different screen sizes.
Benefits of SCSS
SCSS offers several benefits for web developers:
- Improved Maintainability: The use of variables, mixins, and inheritance makes stylesheets more maintainable. Changes can be made in one place, reducing the risk of errors.
- Enhanced Readability: Nesting and partials improve the readability of stylesheets, making it easier to understand the structure and relationships between elements.
- Code Reuse: Mixins and inheritance promote code reuse, reducing redundancy and keeping stylesheets DRY (Don't Repeat Yourself).
- Consistency: Variables ensure consistency across a project, reducing the likelihood of design discrepancies.
- Powerful Tools: The addition of operators, functions, and advanced features makes SCSS a powerful tool for creating dynamic and responsive designs.
Conclusion
SCSS is a valuable addition to any web developer's toolkit. Its features enhance the flexibility and maintainability of CSS, allowing for more complex and scalable projects. By adopting SCSS in your workflow, you can streamline your development process and create more robust stylesheets.
Whether you're a beginner or an experienced developer, SCSS has something to offer. Experiment with its features, explore its documentation, and start incorporating it into your projects for a more efficient and enjoyable web development experience.