Understanding Ruby on Rails Active Job: A Comprehensive Guide
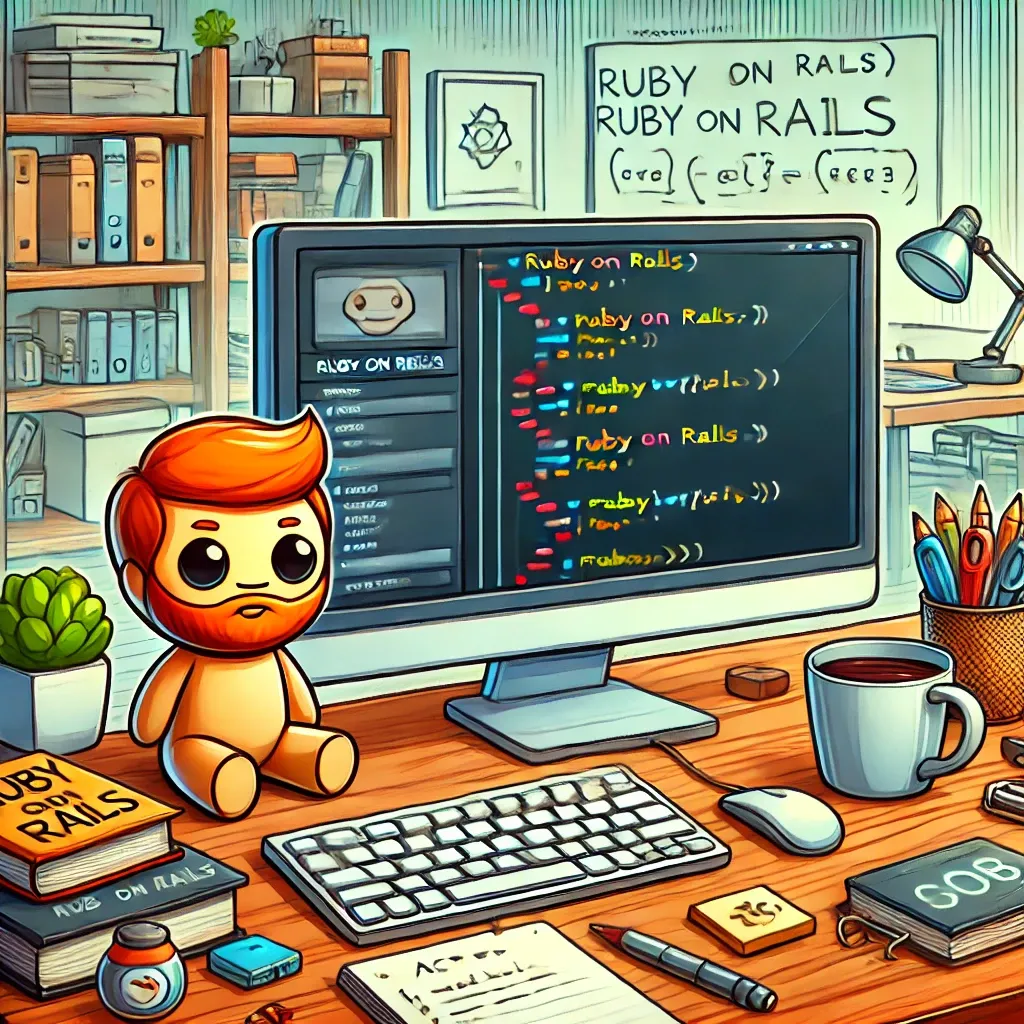
Ruby on Rails (RoR) is a powerful framework for building web applications, and Active Job is a part of the Rails ecosystem that provides a framework for declaring jobs and making them run on a variety of queueing backends. In this blog post, we'll explore what Active Job is, why it's useful, and how you can implement it in your Rails applications to handle background jobs efficiently.
What is Active Job?
Active Job is a framework for declaring jobs in Ruby on Rails, making it easier to run background processes like sending emails, updating data, or generating reports asynchronously. It provides a standardized interface for job processing, which means you can switch between different backend processing libraries (like Sidekiq, Resque, Delayed Job, etc.) without changing the job's code.
Why Use Active Job?
1. Simplified Background Processing
Active Job abstracts the complexity of managing background jobs across different systems. This abstraction allows developers to write jobs that are backend agnostic, meaning you can start with a simple backend like the built-in Async
adapter and scale up to more complex systems like Sidekiq or Resque as your application grows.
2. Unified Interface
With Active Job, you can define jobs in a single way regardless of the backend you choose. This unified interface helps maintain cleaner, more maintainable code.
3. Easy Error Handling and Retry Logic
Active Job allows you to easily handle job errors and implement retry logic, ensuring robust job processing in your Rails application.
How to Set Up Active Job
Let's walk through a basic setup of Active Job in a Ruby on Rails application.
Step 1: Generate a Job
To create a new job, use the Rails generator:
rails generate job Example
This command creates a new job file in the app/jobs
directory:
# app/jobs/example_job.rb
class ExampleJob < ApplicationJob
queue_as :default
def perform(*args)
# Do something later
end
end
Step 2: Enqueue the Job
To enqueue the job, use the perform_later
method. This method will push the job into the queue to be processed asynchronously:
ExampleJob.perform_later('Hello, World!')
You can enqueue jobs from controllers, models, or any part of your Rails application.
Step 3: Configure the Job Queue Adapter
Rails comes with a built-in asynchronous adapter, but for production environments, you'll likely want to use a more robust solution like Sidekiq or Resque. To configure the queue adapter, open the config/application.rb
file and set the desired adapter:
# config/application.rb
config.active_job.queue_adapter = :sidekiq
Make sure you have the appropriate gem installed for your chosen adapter. For example, if you're using Sidekiq, add it to your Gemfile
:
gem 'sidekiq'
Then, run bundle install
to install the gem.
Step 4: Handling Job Errors and Retries
Active Job makes it easy to handle errors and implement retries. You can specify the number of retry attempts and the delay between retries by using the retry_on
method:
class ExampleJob < ApplicationJob
queue_as :default
retry_on StandardError, wait: 5.seconds, attempts: 3
def perform(*args)
# Potentially dangerous operation
end
end
Step 5: Scheduling Jobs
Active Job also supports scheduling jobs to run in the future. You can use the set
method to specify a delay:
ExampleJob.set(wait: 1.minute).perform_later('Hello, delayed World!')
This will schedule the job to run one minute from the time it is enqueued.
Real-World Use Cases for Active Job
Here are some common scenarios where Active Job shines:
- Sending Emails: Use Active Job to send emails in the background without blocking the main thread, improving your application's response time.
- Data Processing: Perform heavy data processing tasks asynchronously, such as generating reports or processing user uploads.
- Integrations with Third-Party Services: Interact with third-party services without making the user wait for the operation to complete.
Conclusion
Active Job in Ruby on Rails provides a powerful yet simple interface for handling background jobs. By abstracting away the complexities of different backend systems, it allows developers to focus on writing business logic rather than managing job queues. Whether you're sending emails, processing data, or interacting with external services, Active Job has you covered.
By following the steps in this guide, you can easily implement background job processing in your Rails applications, improving performance and enhancing the user experience.
For more detailed information, refer to the official Ruby on Rails Active Job documentation.