Understanding Ruby Classes and Objects
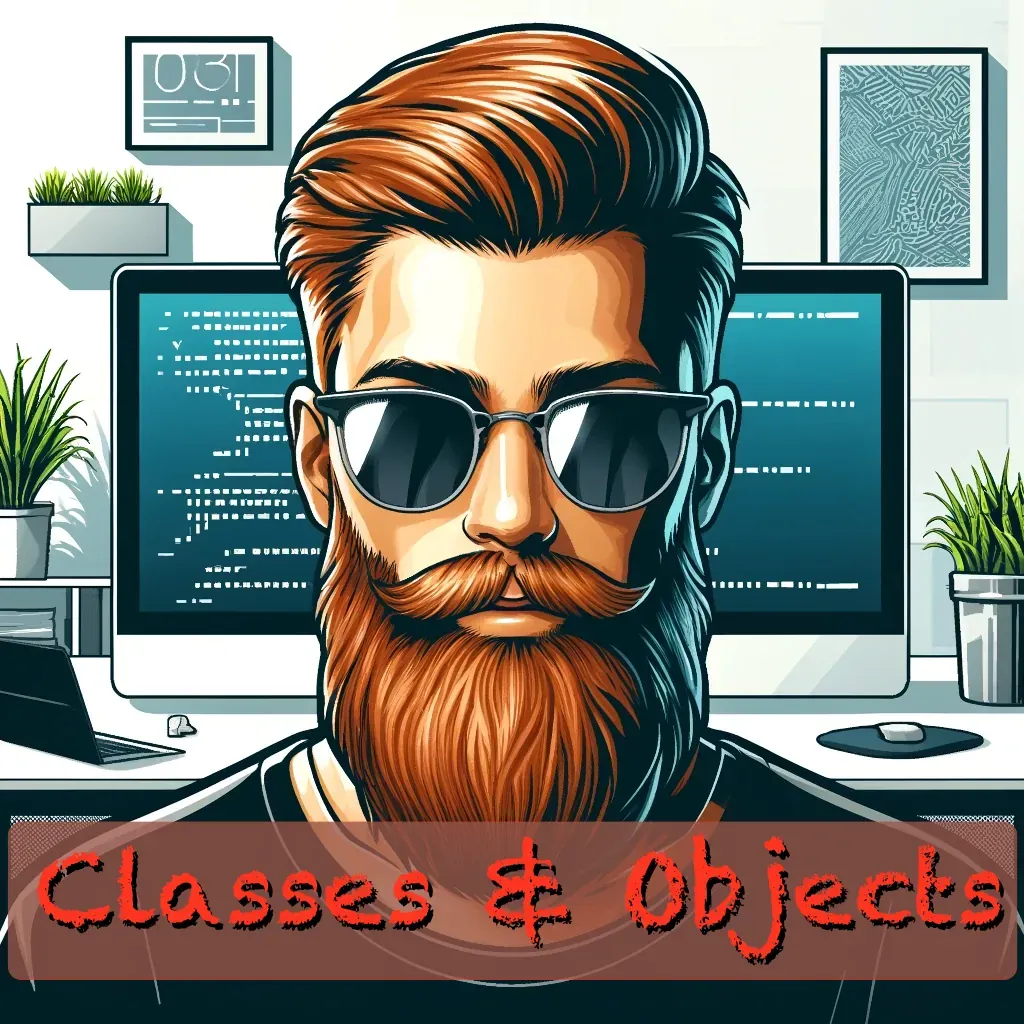
In Ruby, everything is an object. To understand how Ruby works, it's crucial to grasp the concepts of classes and objects. This post will explore these fundamental concepts, explaining how to define classes and create objects.
What is a Class?
A class in Ruby serves as a blueprint for creating objects. It defines a set of properties and methods that describe the behavior of objects created from the class. Here's a basic example of a Ruby class:
class Car
attr_accessor :make, :model, :year
def initialize(make, model, year)
@make = make
@model = model
@year = year
end
def start_engine
puts "Engine started for #{@make} #{@model}"
end
end
In this example, the Car
class has three attributes (make
, model
, and year
) and a method (start_engine
). The initialize
method is a special method that gets called when an object is created from the class.
Creating Objects from Classes
An object is an instance of a class. To create an object, you use the new
keyword, followed by any initialization parameters. Here's how to create a new Car
object:
my_car = Car.new('Toyota', 'Corolla', 2020)
my_car.start_engine
Output:
Engine started for Toyota Corolla
This code snippet creates a new Car
object with the specified attributes and calls the start_engine
method, resulting in the output "Engine started for Toyota Corolla."
Adding Methods to Classes
Classes can have multiple methods to define their behavior. Let's add a method to describe the car:
class Car
attr_accessor :make, :model, :year
def initialize(make, model, year)
@make = make
@model = model
@year = year
end
def start_engine
puts "Engine started for #{@make} #{@model}"
end
def describe
puts "This is a #{@year} #{@make} #{@model}."
end
end
Now you can create an object and call the new describe
method:
my_car = Car.new('Toyota', 'Corolla', 2020)
my_car.describe
Output:
This is a 2020 Toyota Corolla.
The describe
method outputs a brief description of the car, showing the year
, make
, and model
attributes.
Helpful Videos
Conclusion
In Ruby, classes define the blueprint for objects, while objects are instances of those classes. This basic understanding of classes and objects is crucial for Ruby programming. You can create complex programs by defining custom classes and creating objects to represent real-world entities.
With this knowledge, you're well on your way to mastering Ruby's object-oriented programming concepts.