Understanding PHP Classes: A Beginner's Guide
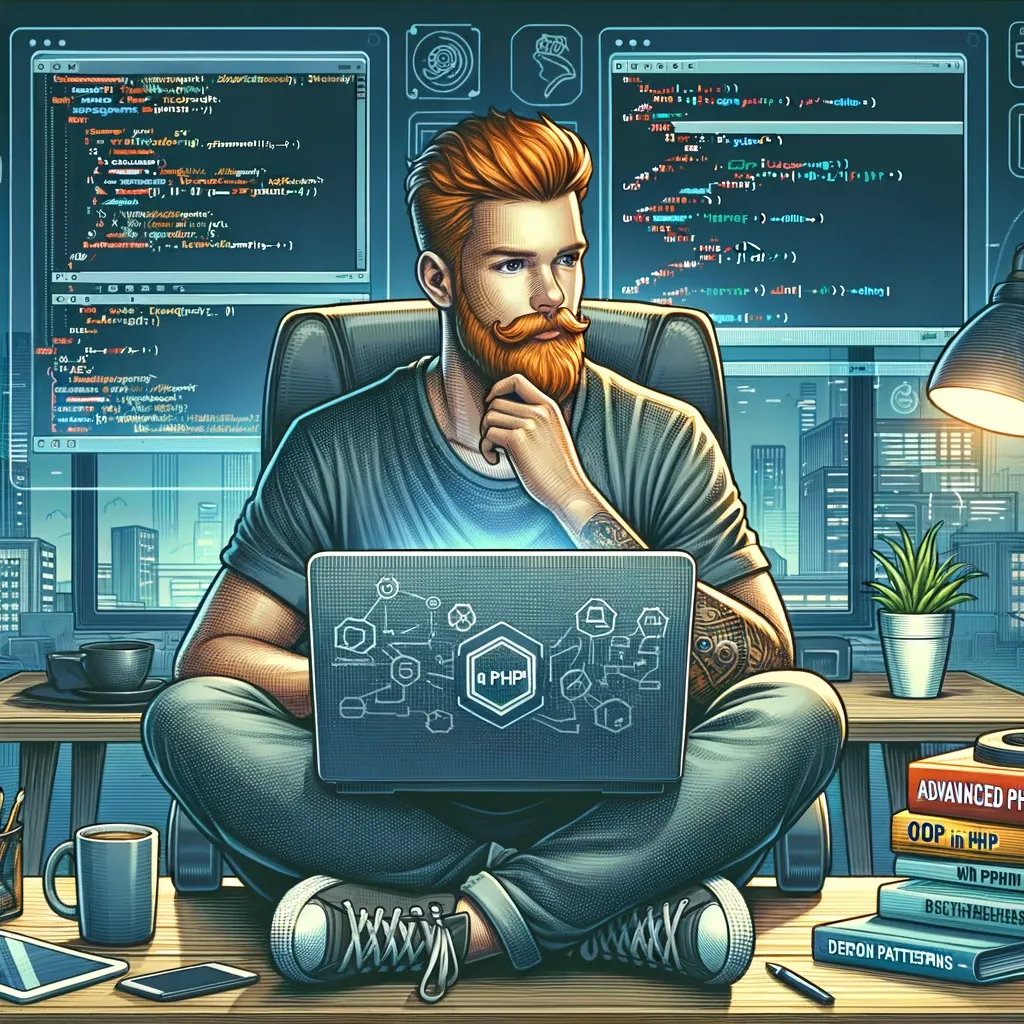
Object-oriented programming (OOP) is a programming paradigm that uses "objects" to design applications and computer programs. PHP, a server-side scripting language, supports OOP by allowing you to define your own classes. In this tutorial, we will explore the basics of creating and using classes in PHP.
What is a Class?
A class in PHP is a blueprint for creating objects. A class encapsulates data for the object, using properties, and methods to manipulate that data.
Defining a Class
To define a class in PHP, you use the class
keyword followed by the name of the class. Here's a simple example:
class Car {
// Properties
public $color;
public $model;
// Method
public function __construct($color, $model) {
$this->color = $color;
$this->model = $model;
}
public function getMessage() {
return "My car is a " + $this->model + " and it's " + $this->color + ".";
}
}
In this example, Car
class has two properties: color
and model
. It also has a constructor method __construct
which initializes the properties when a new object is instantiated, and a method getMessage
that returns a string about the car.
Creating an Object
To create an instance of a class, use the new
keyword:
$myCar = new Car("black", "Volvo");
echo $myCar->getMessage();
Output:
My car is a Volvo and it's black.
This code snippet creates an object $myCar
of class Car
and initializes it with "black" as the color and "Volvo" as the model. It then calls the getMessage
method to print information about the car.
Advanced Uses of PHP Classes
PHP classes can significantly simplify the development process by providing structured, modular, and reusable code. Below, we explore three advanced examples: a database handler, a session manager, and the use of a design pattern.
1. Database Handler Class
A common use of classes in PHP is to handle database operations. Below is a simplified example of a database handler class using PDO (PHP Data Objects) for database interaction.
class DatabaseHandler {
private $host = 'localhost';
private $dbname = 'test_db';
private $user = 'root';
private $pass = 'password';
private $conn;
public function __construct() {
try {
$this->conn = new PDO("mysql:host=$this->host;dbname=$this->dbname", $this->user, $this->pass);
$this->conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
} catch(PDOException $e) {
echo "Connection failed: " + $e->getMessage();
}
}
public function fetchData($sql) {
$stmt = $this->conn->prepare($sql);
$stmt->execute();
return $stmt->fetchAll(PDO::FETCH_ASSOC);
}
public function __destruct() {
$this->conn = null;
}
}
This class handles the connection to a MySQL database, executes queries, and closes the connection automatically when the object is destroyed.
2. Session Management Class
Classes can also be used to encapsulate session management logic, providing a more organized way to handle user sessions.
class SessionManager {
public function startSession() {
session_start();
echo "Session started";
}
public function setSessionData($key, $value) {
$_SESSION[$key] = $value;
}
public function getSessionData($key) {
return $_SESSION[$key] ?? null;
}
public function destroySession() {
session_unset();
session_destroy();
echo "Session destroyed";
}
}
This class provides methods to start a session, set and get session data, and destroy the session, encapsulating all session-related functionality.
3. Singleton Design Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. Here's how you can implement a Singleton pattern in PHP:
class Singleton {
private static $instance = null;
private function __construct() {
// private constructor to prevent multiple instances
}
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new Singleton();
}
return self::$instance;
}
}
This Singleton class restricts the instantiation of a class to one object and is useful for managing connections or settings globally.
Conclusion
Classes are a powerful feature of PHP that allow you to encapsulate and organize your code into reusable components. This brief tutorial introduced you to the syntax of classes in PHP, how to define methods and properties, and how to instantiate an object.
Object-oriented programming in PHP can enhance your code's modularity, reusability, and scalability. As you become more familiar with OOP principles, you will find it easier to manage complex applications and develop robust PHP-based solutions.