Understanding GraphQL: The Basics and Its Usefulness
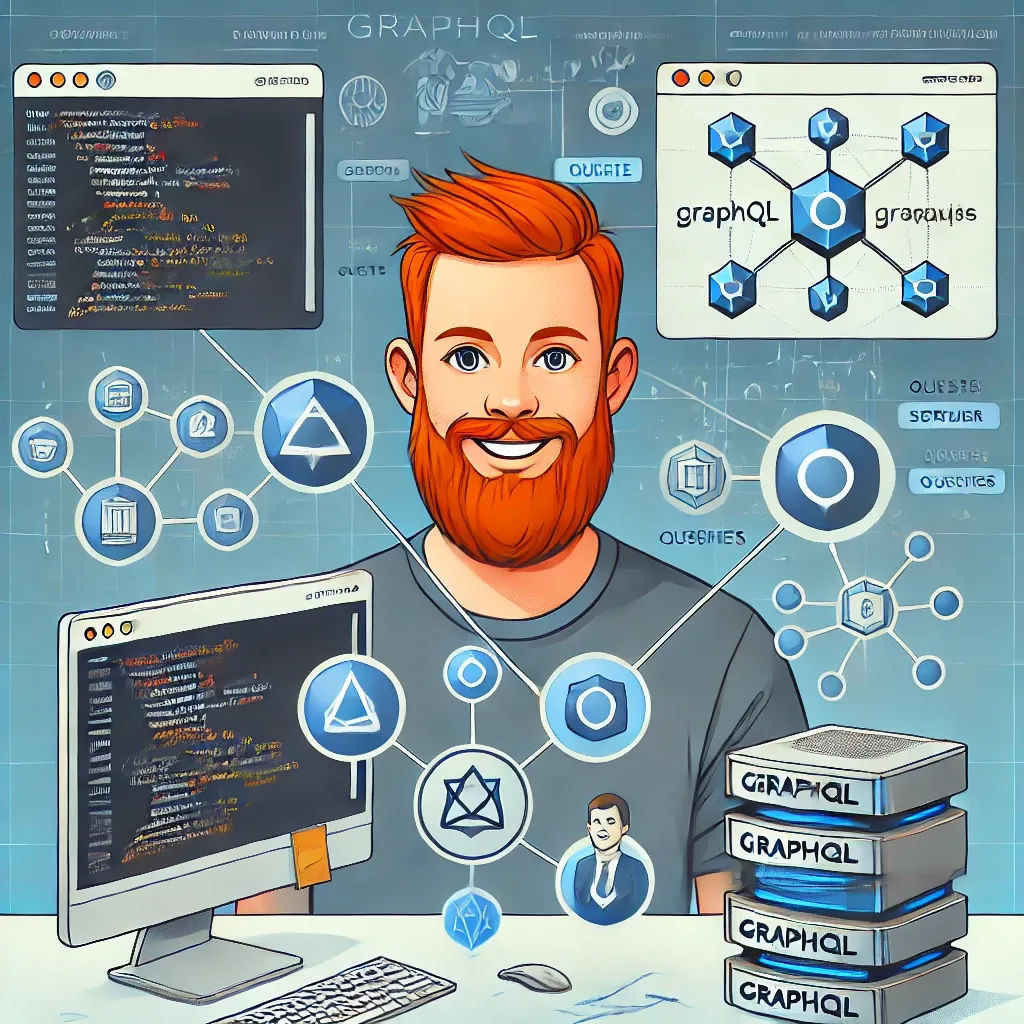
Introduction
GraphQL is a query language for APIs and a runtime for executing those queries by using a type system you define for your data. Developed by Facebook in 2012 and released as an open-source project in 2015, GraphQL provides a more efficient, powerful, and flexible alternative to REST.
What is GraphQL?
GraphQL stands for Graph Query Language. It's a syntax that describes how to ask for data and is generally used to load data from a server to a client. It allows clients to request exactly what they need and nothing more, making it possible to get all required data in a single request.
Key Features of GraphQL
- Hierarchical: A GraphQL query is hierarchical. The shape of the query matches the shape of the result. This makes it easy to predict what the response will look like.
- Strongly Typed: Every GraphQL server defines an application-specific schema. Clients can only query with what’s allowed by the schema.
- Client-Specified Queries: Clients have the power to specify not only the structure but also the granularity of the response.
- Introspection: GraphQL APIs are self-documenting. The type system can be queried to understand the schema, making it easier to work with APIs.
Basics of GraphQL
Schema Definition
A GraphQL schema is a model of the data that can be queried through your API. It defines what queries are allowed and what types of objects can be returned.
type Query {
user(id: ID!): User
}
type User {
id: ID!
name: String
age: Int
friends: [User]
}
Query Example
A basic GraphQL query to fetch a user's name and age might look like this:
{
user(id: "1") {
name
age
}
}
The server’s response will match the shape of the query:
{
"data": {
"user": {
"name": "John Doe",
"age": 28
}
}
}
Mutations
Besides querying for data, GraphQL also supports writing data with mutations. Here's an example mutation to update a user's name:
mutation {
updateUser(id: "1", name: "Jane Doe") {
id
name
}
}
Why GraphQL is Useful
- Efficiency: Avoids over-fetching or under-fetching data, allowing clients to request exactly what they need.
- Flexibility: Clients can specify precisely what they require and in what format, reducing the need for multiple endpoints.
- Strongly Typed Schema: Helps in validating queries against the schema before executing them, reducing runtime errors.
- Versionless API: With GraphQL, there is no need for versioning. The schema can evolve by deprecating old fields and adding new ones.
- Better Developer Experience: Built-in tools like GraphiQL make it easier to explore and interact with the API.
Step-by-Step Guide to Setting Up GraphQL
Setting up GraphQL involves several steps, including setting up the server, defining the schema, and creating resolvers. This will guide you through setting up a basic GraphQL server using Node.js and Express with the graphql
and express-graphql
libraries.
1. Install Node.js and npm
First, make sure you have Node.js and npm installed. You can download them from the official Node.js website.
2. Set Up a New Node.js Project
Create a new directory for your project and navigate into it:
mkdir graphql-server
cd graphql-server
npm init -y
3. Install Required Dependencies
Install the necessary packages:
npm install express express-graphql graphql
4. Create the Server
Create a new file server.js
and set up a basic Express server with GraphQL.
const express = require('express');
const { graphqlHTTP } = require('express-graphql');
const { buildSchema } = require('graphql');
// Define the schema
const schema = buildSchema(`
type Query {
hello: String
}
`);
// Define the root resolver
const root = {
hello: () => {
return 'Hello, world!';
},
};
const app = express();
app.use('/graphql', graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true,
}));
app.listen(4000, () => {
console.log('Running a GraphQL API server at http://localhost:4000/graphql');
});
5. Run the Server
Run the server with the following command:
node server.js
You should see a message indicating that the server is running:
Running a GraphQL API server at http://localhost:4000/graphql
6. Test Your GraphQL Server
Open your browser and navigate to http://localhost:4000/graphql
. You should see the GraphiQL interface, which you can use to interact with your GraphQL API.
In the GraphiQL interface, enter the following query and click the "Execute" button:
{
hello
}
You should see the following response:
{
"data": {
"hello": "Hello, world!"
}
}
Adding More Complexity
To demonstrate a more complex example, let's add a user type with fields and queries.
Update Schema and Resolvers
Modify your server.js
to include the new schema and resolvers:
const express = require('express');
const { graphqlHTTP } = require('express-graphql');
const { buildSchema } = require('graphql');
// Define the schema
const schema = buildSchema(`
type User {
id: ID!
name: String
age: Int
}
type Query {
user(id: ID!): User
users: [User]
}
`);
// Sample data
const users = [
{ id: 1, name: 'John Doe', age: 28 },
{ id: 2, name: 'Jane Doe', age: 25 },
];
// Define the root resolver
const root = {
user: ({ id }) => users.find(user => user.id == id),
users: () => users,
};
const app = express();
app.use('/graphql', graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true,
}));
app.listen(4000, () => {
console.log('Running a GraphQL API server at http://localhost:4000/graphql');
});
Test the New Queries
In the GraphiQL interface, enter the following query to fetch a specific user and all users:
{
user(id: 1) {
name
age
}
users {
id
name
age
}
}
You should see a response with the user data:
{
"data": {
"user": {
"name": "John Doe",
"age": 28
},
"users": [
{
"id": "1",
"name": "John Doe",
"age": 28
},
{
"id": "2",
"name": "Jane Doe",
"age": 25
}
]
}
}
Conclusion
GraphQL offers a modern way to work with APIs by providing a flexible and efficient alternative to REST. Its ability to allow clients to request specific data, its strongly typed schema, and its introspective nature make it a powerful tool for API development. Whether you're building a new API or working with an existing one, GraphQL can help streamline data fetching and improve overall performance.
This guide has walked you through the basics of setting up a GraphQL server using Node.js and Express. By following these steps, you can start building more complex GraphQL APIs tailored to your specific needs. GraphQL's flexibility and efficiency can significantly enhance your API development experience, providing powerful tools for managing data and building robust applications.