SCSS Mixins: Reusable Blocks for Efficient Stylesheets
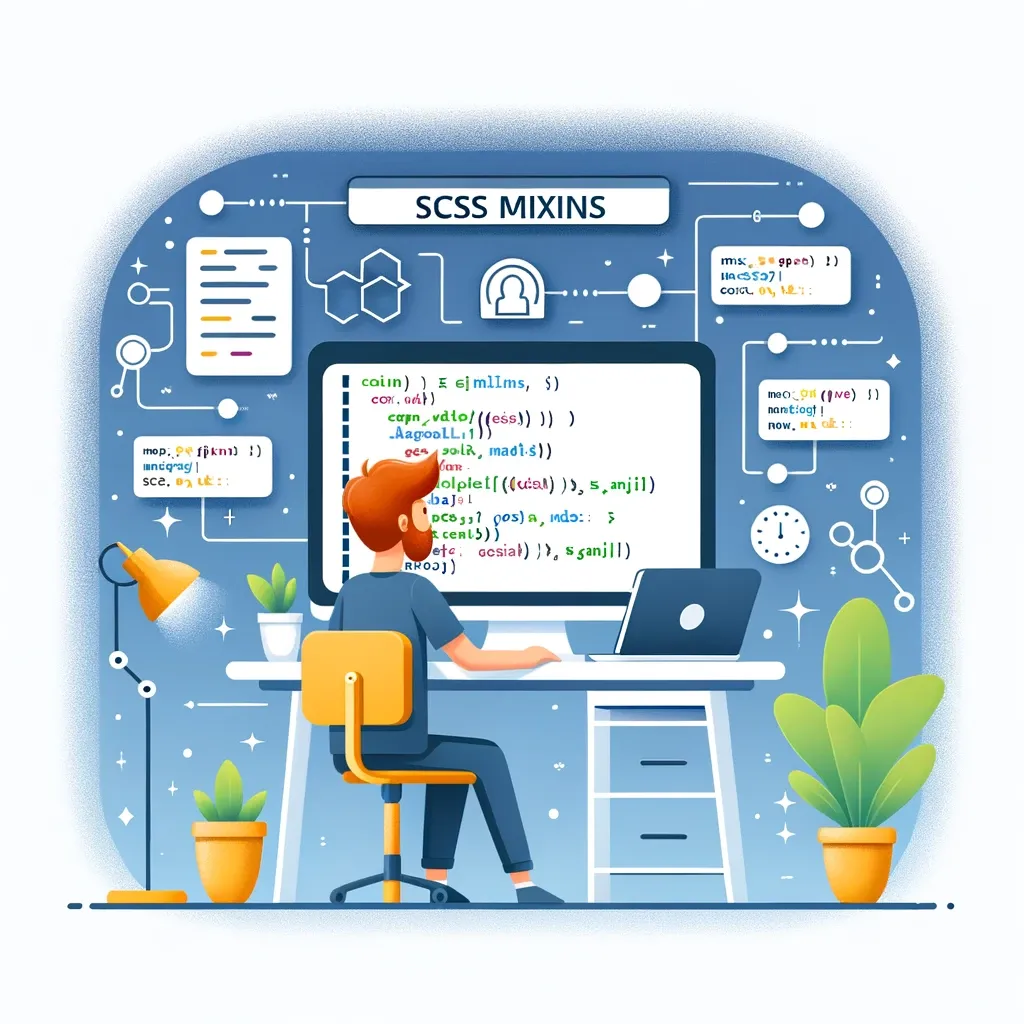
Introduction to SCSS Mixins
SCSS (Sassy CSS) is renowned for its powerful features, one of which is mixins. Mixins are reusable blocks of code that can be included in multiple places, allowing developers to maintain a clean and modular structure in their stylesheets. This article explores SCSS mixins, explaining their importance, use cases, and practical examples to demonstrate how they can improve the efficiency and maintainability of your CSS.
What are SCSS Mixins?
In simple terms, mixins in SCSS are chunks of code that can be reused throughout your stylesheet. They are particularly useful for reducing redundancy and keeping your code DRY (Don't Repeat Yourself). With mixins, you can define complex styles once and then apply them wherever needed, streamlining your development process.
Mixins can also accept parameters, allowing you to create dynamic and flexible code snippets. This feature is handy when you need to apply variations of a style without writing repetitive code.
Creating SCSS Mixins
Creating a mixin in SCSS is straightforward. You use the @mixin
keyword followed by the mixin name and any optional parameters. Here's an example of a simple mixin that centers content using flexbox:
@mixin flex-center {
display: flex;
justify-content: center;
align-items: center;
}
.container {
@include flex-center;
height: 100vh;
}
In this example, the flex-center
mixin defines the necessary flexbox properties to center content. We then use the @include
keyword to apply the mixin to the .container
class, centering its content vertically and horizontally.
SCSS Mixins with Parameters
Mixins become even more powerful when you add parameters, allowing you to pass different values and create flexible styles. Here's an example of a mixin with a parameter to set the border radius:
@mixin border-radius($radius) {
border-radius: $radius;
}
.button {
@include border-radius(10px);
}
.card {
@include border-radius(5px);
}
In this example, the border-radius
mixin accepts a parameter $radius
to define the border radius. We use this mixin to apply different border radii to a .button
and a .card
. This approach reduces redundancy and allows for easy updates if needed.
Combining Mixins in SCSS
Another advantage of SCSS mixins is that you can combine multiple mixins to create complex styles. This feature enables you to build reusable style blocks that can be used across different components. Here's an example of combining mixins to create a styled button:
@mixin flex-center {
display: flex;
justify-content: center;
align-items: center;
}
@mixin button-style($bg-color) {
padding: 10px 20px;
background-color: $bg-color;
color: white;
border: none;
cursor: pointer;
text-transform: uppercase;
@include flex-center;
}
.primary-button {
@include button-style(#3498db);
}
.secondary-button {
@include button-style(#2ecc71);
}
In this example, we combine the flex-center
and button-style
mixins to create a styled button. The button-style
mixin accepts a parameter for the background color, allowing you to create different button styles without repeating code.
Benefits of SCSS Mixins
SCSS mixins offer several key benefits:
- Code Reuse: Mixins reduce redundancy by allowing you to define styles once and use them across different components.
- Flexibility: With parameters, mixins can be customized to create variations of a style, providing flexibility in your stylesheets.
- Maintainability: Using mixins, you can keep your stylesheets clean and modular, making them easier to maintain and update.
- Consistency: Mixins ensure consistency across your project, reducing the risk of design discrepancies.
- Reduced Errors: Reusing mixins can help minimize errors, as changes to a mixin are automatically reflected in all places where it's used.
Conclusion
SCSS mixins are a powerful tool for web developers, promoting code reuse, flexibility, and maintainability in stylesheets. By incorporating mixins into your workflow, you can streamline your CSS development process and create consistent, scalable designs. Whether you're building complex web applications or simple websites, mixins can significantly enhance your productivity and code quality.
Experiment with mixins, explore different use cases, and start implementing them in your projects to see the benefits they bring to your development workflow.