Exploring Ruby's reduce Method: Power and Versatility
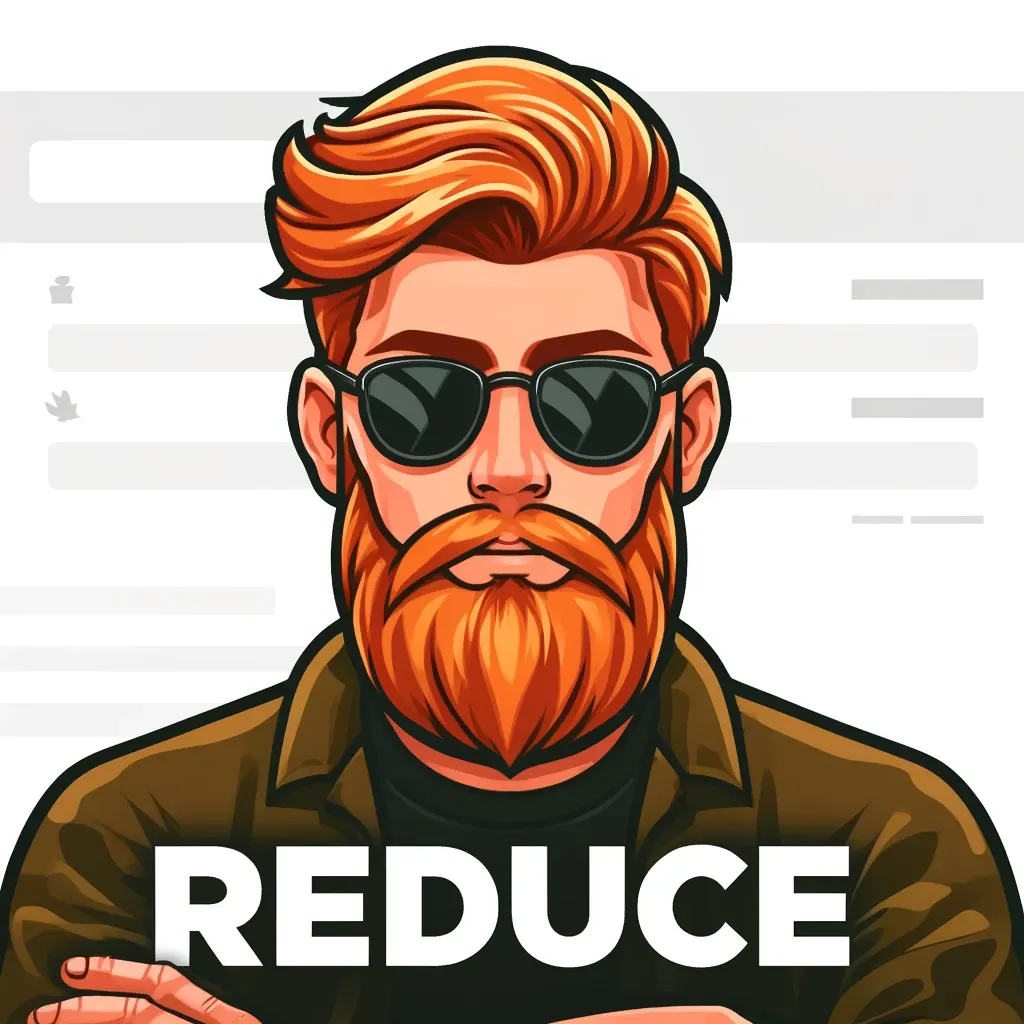
The reduce
method in Ruby, also known as inject
, is a powerful tool that allows you to transform an array or an enumerable into a single value. It's a versatile method with a variety of use cases, from simple aggregation to complex data processing. In this blog post, we'll explore the reduce
method in detail, along with examples to illustrate its capabilities.
The Basics of reduce
The reduce
method combines all elements of an enumerable by applying a binary operation, specified by a block, to each element. The operation is cumulative, meaning the result from one iteration is used in the next. It takes an initial value as an optional argument. Here's a simple example to get started:
numbers = [1, 2, 3, 4, 5]
sum = numbers.reduce(0) { |accumulator, num| accumulator + num }
puts sum
####################################
########### Results ################
####################################
15
This code snippet uses reduce
to calculate the sum of all numbers in the array, starting with an initial value of 0. The accumulator
variable holds the ongoing result, which is then updated by adding each element in the array.
Reducing Without an Initial Value
If no initial value is given, the first element of the enumerable becomes the initial value. This can be useful when the operation being applied doesn't require an external starting point:
numbers = [1, 2, 3, 4, 5]
product = numbers.reduce { |accumulator, num| accumulator * num }
puts product
####################################
########### Results ################
####################################
120
In this example, reduce
calculates the product of all numbers in the array, using the first element as the starting point.
Custom Operations with reduce
reduce
is not limited to arithmetic operations. You can use it to perform any transformation or computation that requires combining elements. For example, you can concatenate strings:
words = ["Ruby", "is", "fun"]
sentence = words.reduce { |accumulator, word| "#{accumulator} #{word}" }
puts sentence
####################################
########### Results ################
####################################
Ruby is fun
Here, reduce
is used to create a sentence by concatenating words with spaces in between.
Using reduce
to Find Minimum and Maximum Values
reduce
can also be used to find the minimum and maximum values in an array. This can be helpful in scenarios where you need to find the smallest or largest element:
numbers = [4, 2, 7, 1, 8, 3]
min_value = numbers.reduce { |min, num| num < min ? num : min }
max_value = numbers.reduce { |max, num| num > max ? num : max }
puts "Min: \#{min_value}, Max: \#{max_value}"
####################################
########### Results ################
####################################
Min: 1, Max: 8
This example demonstrates how reduce
can be used to find both minimum and maximum values by comparing each element with the current minimum and maximum.
Practical Use Cases for reduce
Beyond basic examples, reduce
can be applied to real-world scenarios. Here are a few practical use cases:
Summing Objects' Attributes:reduce
can be used to sum attributes of objects within an array, which is common in financial calculations or inventory management.
items = [
{ name: "Item 1", price: 10 },
{ name: "Item 2", price: 20 },
{ name: "Item 3", price: 30 }
]
total_price = items.reduce(0) { |total, item| total + item[:price] }
puts total_price
####################################
########### Results ################
####################################
60
This code snippet uses reduce
to calculate the total price of a list of items.
Flattening Nested Arrays:reduce
can be used to flatten nested arrays, which is helpful in data restructuring or cleaning.
nested_array = [[1, 2], [3, 4], [5, 6]]
flat_array = nested_array.reduce([]) do |flat, inner_array|
flat.concat(inner_array)
end
puts flat_array
####################################
########### Results ################
####################################
[1, 2, 3, 4, 5, 6]
This example demonstrates how reduce
can be used to flatten a nested array into a single flat array.
Counting Occurrences:reduce
can be used to count the occurrences of elements in an array, useful in data analysis or text processing.
words = ["apple", "banana", "orange", "apple", "orange", "orange"]
word_count = words.reduce(Hash.new(0)) do |counts, word|
counts[word] += 1
counts
end
puts word_count
####################################
########### Results ################
####################################
{"apple": 2, "banana": 1, "orange": 3}
This code snippet creates a hash that counts the occurrences of each word in the array.
Conclusion
The reduce
method in Ruby is an incredibly versatile tool that allows you to perform a wide range of operations on enumerables. From simple arithmetic to complex data transformations, reduce
offers a concise and powerful way to manipulate collections. Whether you're summing numbers, concatenating strings, or processing complex data structures, reduce
is a valuable addition to your Ruby toolkit.