Python in Web Development: Powerful Frameworks and Versatile Tools
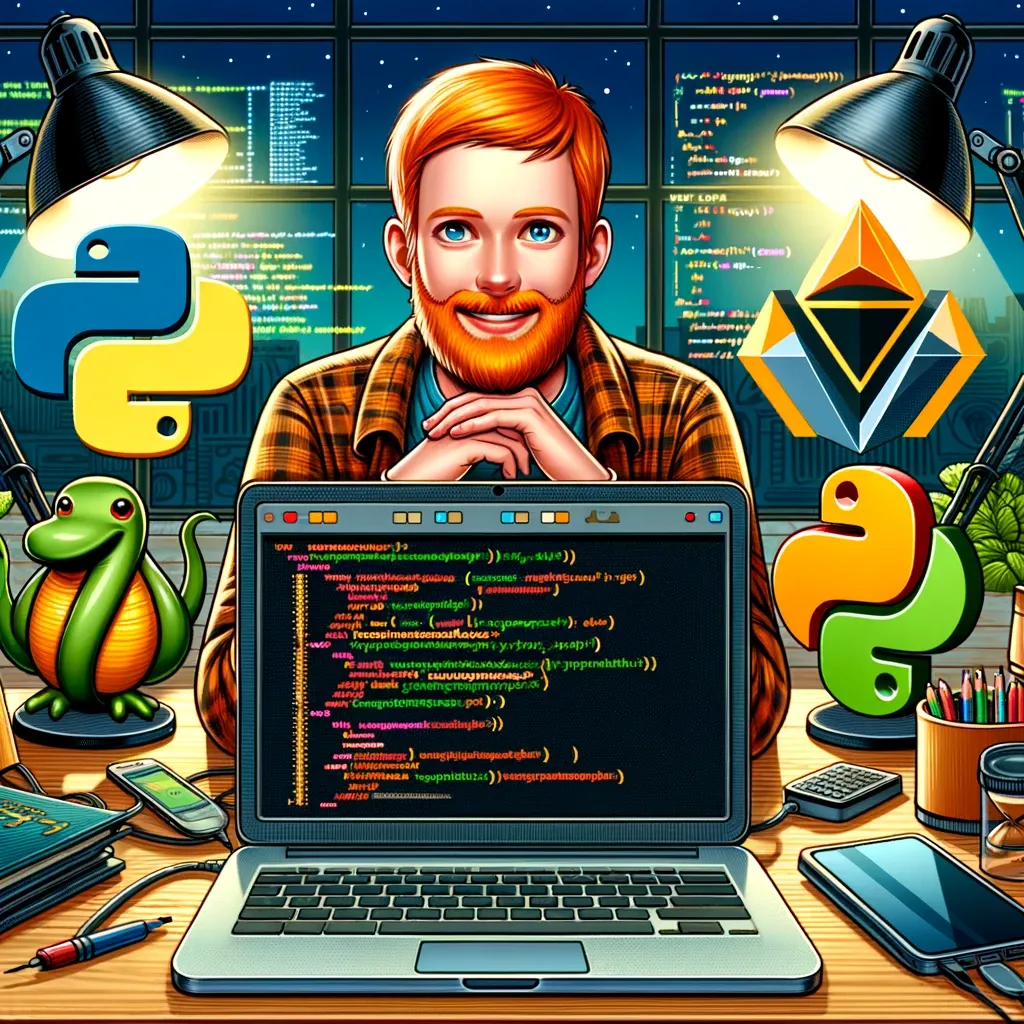
Python has become a popular choice for web development due to its simplicity, readability, and powerful frameworks. This blog post will explore how Python is used in web development, the frameworks that make it stand out, and why it is a preferred language for many web developers.
Why Use Python for Web Development?
Python offers several advantages that make it ideal for web development:
- Readability and Maintainability: Python's clean and readable syntax ensures that developers can easily understand and maintain the code.
- Rapid Development: Python's simplicity allows for rapid development and iteration, reducing the time to market.
- Large Standard Library: Python's extensive standard library supports many web development tasks out of the box.
- Strong Community: The active Python community provides extensive resources, libraries, and frameworks to support web development.
Popular Python Frameworks for Web Development
Python offers several frameworks that simplify and streamline the web development process. Here are some of the most popular ones:
1. Django
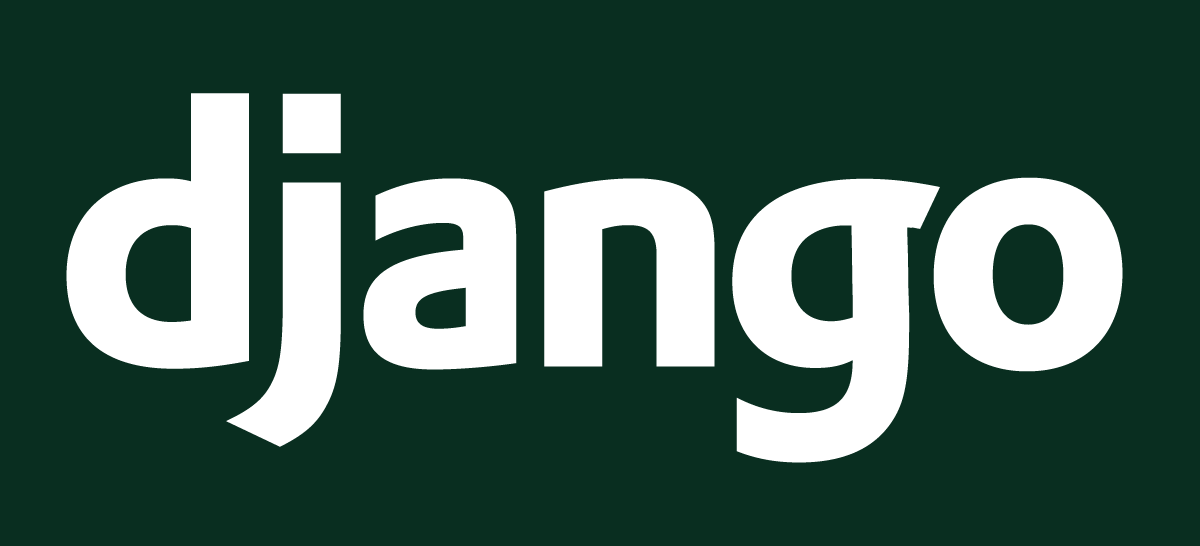
Django is a high-level web framework that encourages rapid development and clean, pragmatic design. It includes many built-in features that make it a powerful tool for building complex web applications.
What Makes Django Stand Out?
Django comes with several features that make it a powerful tool for web development:
- ORM (Object-Relational Mapping): Allows developers to interact with the database using Python objects.
- Admin Interface: Automatically generates a web-based admin interface for managing application data.
- Security: Includes built-in protection against common security threats like SQL injection, cross-site scripting, and cross-site request forgery.
- Scalability: Designed to handle high-traffic websites and applications.
- Batteries-Included Philosophy: Comes with many built-in features, reducing the need to install and configure third-party libraries.
Setting Up Django
Before we dive into the examples, let's set up a Django project. First, ensure you have Python and pip installed. Then, install Django using pip:
pip install django
Next, create a new Django project:
django-admin startproject myproject
cd myproject
Start the development server to ensure everything is set up correctly:
python manage.py runserver
Visit http://127.0.0.1:8000/
in your browser, and you should see the Django welcome page.
Creating a Simple Django Application
Let's create a simple application to demonstrate some of Django's features. We'll build a blog application.
Create a New App:
python manage.py startapp blog
Define Models:
In blog/models.py
, define the Post
model:
from django.db import models
from django.utils import timezone
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
created_at = models.DateTimeField(default=timezone.now)
def __str__(self):
return self.title
Create and Apply Migrations:
Generate and apply the database migrations:
python manage.py makemigrations blog
python manage.py migrate
Register the Model in the Admin Interface:
In blog/admin.py
, register the Post
model:
from django.contrib import admin
from .models import Post
admin.site.register(Post)
Create Views:
In blog/views.py
, create a view to display blog posts:
from django.shortcuts import render
from .models import Post
def index(request):
posts = Post.objects.all()
return render(request, 'blog/index.html', {'posts': posts})
Configure URLs:
In blog/urls.py
, define the URL pattern for the index view:
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
]
In myproject/urls.py
, include the blog
URLs:
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('admin/', admin.site.urls),
path('blog/', include('blog.urls')),
]
Create Templates:
Create the index.html
template in blog/templates/blog/
:
<!DOCTYPE html>
<html>
<head>
<title>Blog</title>
</head>
<body>
<h1>Blog Posts</h1>
<ul>
{% for post in posts %}
<li>{{ post.title }} - {{ post.created_at }}</li>
{% endfor %}
</ul>
</body>
</html>
Now, start the development server and visit http://127.0.0.1:8000/blog/
to see the list of blog posts.
Useful Features of Django
1. Django Admin Interface
Django's admin interface is one of its standout features. It allows you to manage your application's data without writing a single line of code. You can customize the admin interface by creating custom admin classes.
from django.contrib import admin
from .models import Post
class PostAdmin(admin.ModelAdmin):
list_display = ('title', 'created_at')
search_fields = ('title',)
admin.site.register(Post, PostAdmin)
2. User Authentication
Django comes with a built-in authentication system that handles user registration, login, logout, password reset, and more. It provides decorators and mixins to protect views and manage user permissions.
from django.contrib.auth.decorators import login_required
@login_required
def secret_view(request):
return render(request, 'secret.html')
3. Form Handling
Django simplifies form handling with its form classes. You can define forms using Python classes and render them in templates. Django handles form validation and error messages automatically.
from django import forms
from .models import Post
class PostForm(forms.ModelForm):
class Meta:
model = Post
fields = ['title', 'content']
4. Middleware
Django's middleware framework allows you to process requests and responses globally. Middleware components can be used for a variety of tasks, such as modifying the request or response, handling sessions, and more.
class MyCustomMiddleware:
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
# Code to execute before the view
response = self.get_response(request)
# Code to execute after the view
return response
Django is a powerful and versatile framework that simplifies the web development process. Its extensive features, such as the admin interface, ORM, and built-in security, make it a top choice for developers. Whether you're building a small website or a large-scale web application, Django provides the tools and flexibility you need to succeed.
2. Flask
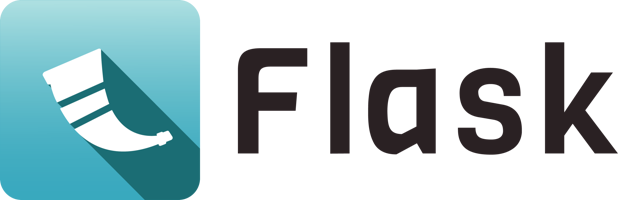
Flask is a lightweight web framework for Python that is designed to make getting started quick and easy, with the ability to scale up to complex applications. Flask is known for its simplicity and flexibility, making it a popular choice for web development. In this post, we'll explore Flask's features and provide some practical examples to showcase its capabilities.
What Makes Flask Stand Out?
Flask offers several features that make it a powerful tool for web development:
- Lightweight and Modular: Flask provides the essential components to build web applications while allowing developers to choose additional libraries and tools as needed.
- Flexibility: Flask's minimalistic core allows you to use the tools and libraries that best suit your project's needs.
- Easy to Learn: Flask's simplicity and straightforward design make it easy for developers to learn and use.
- Extensive Documentation: Flask has extensive and well-organized documentation, which makes it easy to find information and get started.
Setting Up Flask
Before we dive into the examples, let's set up a Flask project. First, ensure you have Python and pip installed. Then, install Flask using pip:
pip install Flask
Creating a Simple Flask Application
Let's create a simple Flask application to demonstrate some of its features. We'll build a basic web application that displays a welcome message.
Create a New Flask Application:
Create a new file called app.py
and add the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
Run the Application:
Start the Flask development server by running the following command in your terminal:
python app.py
Visit http://127.0.0.1:5000/
in your browser, and you should see the message "Hello, World!".
Flask Features and Examples
1. Routing
Flask uses a simple and intuitive routing system to map URLs to functions. You can define routes using the @app.route
decorator.
@app.route('/hello/<name>')
def hello(name):
return f'Hello, {name}!'
2. Templates
Flask uses Jinja2 as its templating engine, allowing you to generate HTML dynamically. Create a folder called templates
and add an index.html
file:
<!DOCTYPE html>
<html>
<head>
<title>Flask App</title>
</head>
<body>
<h1>{{ message }}</h1>
</body>
</html>
In app.py
, render the template:
from flask import render_template
@app.route('/welcome')
def welcome():
return render_template('index.html', message='Welcome to Flask!')
3. Handling Forms
Flask makes it easy to handle forms and validate input. Install Flask-WTF
for form handling:
pip install Flask-WTF
Create a form in forms.py
:
from flask_wtf import FlaskForm
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired
class NameForm(FlaskForm):
name = StringField('What is your name?', validators=[DataRequired()])
submit = SubmitField('Submit')
In app.py
, handle the form submission:
from flask import Flask, render_template, flash, redirect, url_for
from forms import NameForm
app.config['SECRET_KEY'] = 'your_secret_key'
@app.route('/form', methods=['GET', 'POST'])
def form():
form = NameForm()
if form.validate_on_submit():
name = form.name.data
flash(f'Hello, {name}!')
return redirect(url_for('form'))
return render_template('form.html', form=form)
Create a form.html
template:
<!DOCTYPE html>
<html>
<head>
<title>Flask Form</title>
</head>
<body>
<form method="POST">
{{ form.hidden_tag() }}
<p>
{{ form.name.label }}<br>
{{ form.name(size=32) }}<br>
{% for error in form.name.errors %}
<span style="color: red;">[{{ error }}]</span>
{% endfor %}
</p>
<p>{{ form.submit() }}</p>
</form>
{% with messages = get_flashed_messages() %}
{% if messages %}
<ul>
{% for message in messages %}
<li>{{ message }}</li>
{% endfor %}
</ul>
{% endif %}
{% endwith %}
</body>
</html>
4. RESTful APIs
Flask is often used to build RESTful APIs. You can use the flask-restful
extension to simplify this process:
pip install flask-restful
Create a simple API in app.py
:
from flask import Flask
from flask_restful import Resource, Api
app = Flask(__name__)
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'hello': 'world'}
api.add_resource(HelloWorld, '/api')
if __name__ == '__main__':
app.run(debug=True)
Flask is a flexible and lightweight framework that simplifies web development. Its minimalistic core and modular design allow developers to build web applications efficiently. Whether you're creating a small website or a RESTful API, Flask provides the tools and flexibility you need to succeed.
3. Pyramid
Pyramid is a lightweight and flexible web framework for Python, designed to scale with your application. Pyramid is known for its simplicity and powerful features, making it a popular choice for both small and large applications. In this post, we'll explore Pyramid's features and provide some practical examples to showcase its capabilities.

What Makes Pyramid Stand Out?
Pyramid offers several features that make it a powerful tool for web development:
- Flexibility: Pyramid provides a minimalistic core, allowing developers to choose the tools and libraries that best suit their project's needs.
- Scalability: Pyramid is designed to scale with your application, from small websites to large, complex applications.
- Extensibility: Pyramid's modular design makes it easy to extend and customize.
- Security: Pyramid includes built-in security features and allows for custom security policies.
- Traversal and URL Dispatch: Two powerful methods for mapping URLs to code.
Setting Up Pyramid
Before we dive into the examples, let's set up a Pyramid project. First, ensure you have Python and pip installed. Then, install Pyramid using pip:
pip install pyramid
pip install pyramid-cookiecutter-starter
Create a new Pyramid project using the cookiecutter template:
cookiecutter gh:Pylons/pyramid-cookiecutter-starter
cd your_project_name
Create a virtual environment and activate it:
python -m venv env
source env/bin/activate
Install the project dependencies:
pip install -e .
Start the development server:
pserve development.ini
Visit http://127.0.0.1:6543/
in your browser, and you should see the Pyramid welcome page.
Creating a Simple Pyramid Application
Let's create a simple application to demonstrate some of Pyramid's features. We'll build a basic web application that displays a welcome message.
Create a View:
In your_project_name/views.py
, create a view function:
from pyramid.view import view_config
@view_config(route_name='home', renderer='templates/mytemplate.jinja2')
def my_view(request):
return {'project': 'your_project_name'}
Configure Routes:
In your_project_name/__init__.py
, configure the routes:
def main(global_config, **settings):
config = Configurator(settings=settings)
config.include('pyramid_jinja2')
config.add_static_view('static', 'static', cache_max_age=3600)
config.add_route('home', '/')
config.scan()
return config.make_wsgi_app()
Create Templates:
Create the mytemplate.jinja2
template in your_project_name/templates/
:
<!DOCTYPE html>
<html>
<head>
<title>Pyramid App</title>
</head>
<body>
<h1>Welcome to Pyramid!</h1>
<p>Project: {{ project }}</p>
</body>
</html>
Now, start the development server and visit http://127.0.0.1:6543/
to see the welcome message.
Pyramid Features and Examples
1. Traversal
Pyramid's traversal system maps URL paths to resources in a tree-like structure. This can be useful for applications with hierarchical data.
from pyramid.view import view_config
class MyResource(dict):
def __init__(self, request):
super().__init__()
self['child'] = ChildResource()
class ChildResource:
@view_config(renderer='json')
def my_view(self):
return {'message': 'Hello from child resource'}
config.add_route('traversal', '/traversal/*traverse', factory=MyResource)
2. Security
Pyramid's security framework allows you to define custom security policies and protect your views with decorators.
from pyramid.security import Allow, Everyone
from pyramid.view import view_config
from pyramid.response import Response
class RootFactory:
__acl__ = [(Allow, Everyone, 'view')]
def __init__(self, request):
pass
@view_config(permission='view')
def secure_view(request):
return Response('This is a protected view.')
config = Configurator()
config.set_root_factory(RootFactory)
config.add_view(secure_view, route_name='secure')
3. Form Handling
Pyramid integrates well with form libraries like Deform for form handling and validation.
from pyramid.view import view_config
import colander
import deform.widget
class ContactSchema(colander.MappingSchema):
name = colander.SchemaNode(colander.String())
email = colander.SchemaNode(colander.String(), validator=colander.Email())
message = colander.SchemaNode(colander.String(), widget=deform.widget.TextAreaWidget())
@view_config(route_name='contact', renderer='templates/contact.jinja2')
def contact_view(request):
schema = ContactSchema()
form = deform.Form(schema, buttons=('submit',))
if request.method == 'POST':
controls = request.POST.items()
try:
appstruct = form.validate(controls)
# Handle valid form submission
except deform.ValidationFailure as e:
return {'form': e.render()}
return {'form': form.render()}
config.add_route('contact', '/contact')
4. RESTful APIs
Pyramid can be used to build RESTful APIs with the help of extensions like Cornice.
from cornice import Service
hello = Service(name='hello', path='/api/hello', description='Hello service')
@hello.get()
def get_hello(request):
return {'hello': 'world'}
config.scan()
Pyramid is a flexible and powerful framework that simplifies web development. Its modular design, scalability, and extensive features make it a top choice for developers. Whether you're creating a small website or a large-scale web application, Pyramid provides the tools and flexibility you need to succeed.
Python Libraries for Web Development
In addition to frameworks, Python offers various libraries that aid in web development:
- Requests: Simplifies HTTP requests, making it easy to interact with web services.
- BeautifulSoup: Facilitates web scraping by parsing HTML and XML documents.
- SQLAlchemy: Provides a full-featured SQL toolkit and ORM for database access.
Conclusion
Python's simplicity, readability, and extensive library support make it a powerful language for web development. Whether you choose Django for a full-fledged web application, Flask for a lightweight project, or Pyramid for flexibility, Python has the tools and frameworks to help you succeed. Its active community and rich ecosystem ensure that you have access to the resources and support you need to build robust and scalable web applications.