Organizing SCSS with Partials and Import Statements
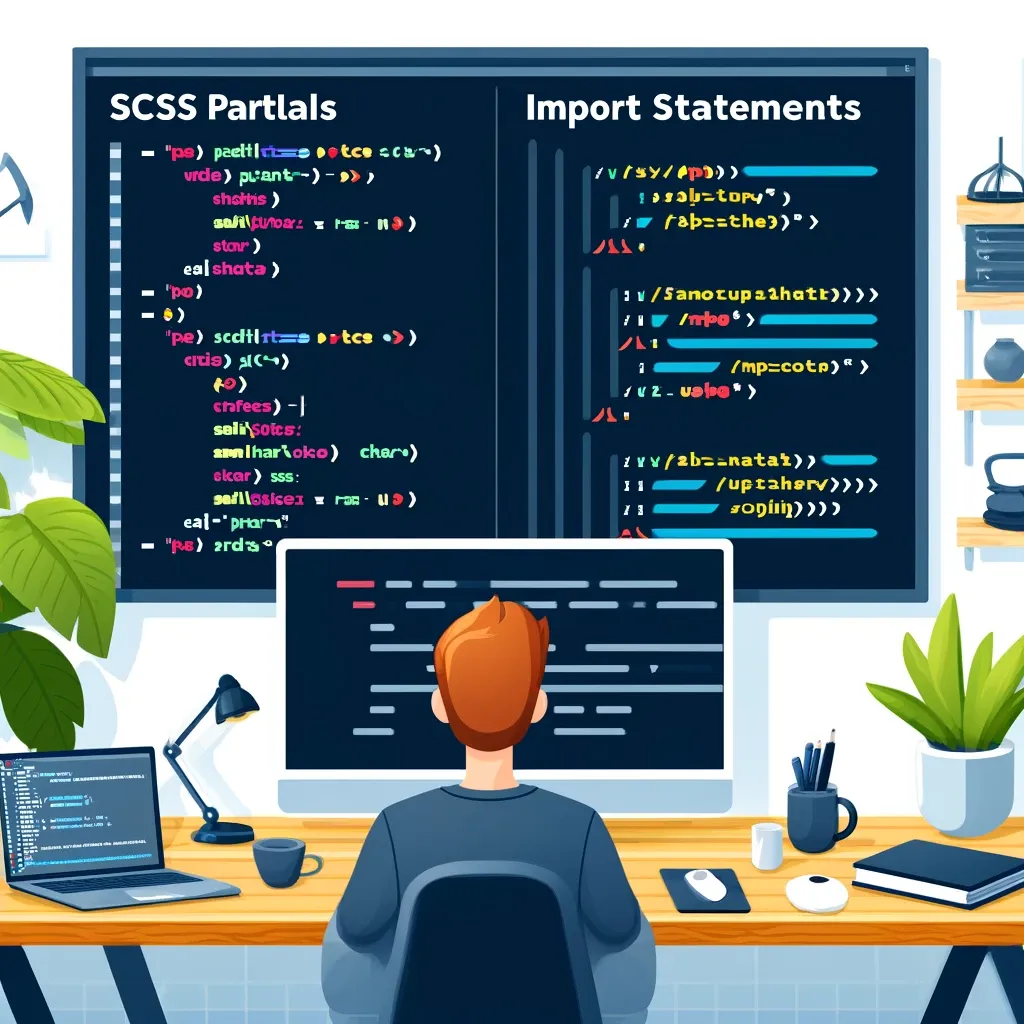
Introduction to SCSS Partials and Import Statements
SCSS (Sassy CSS) offers a range of powerful features, one of which is the ability to create partials and import them into a main stylesheet. This approach allows developers to organize their CSS into smaller, manageable sections, promoting a more modular and scalable structure. In this article, we'll explore the concept of SCSS partials and import statements, their benefits, and how to use them effectively in your projects.
What Are SCSS Partials?
Partials in SCSS are smaller chunks of stylesheets that can be imported into a main file. They are typically used to organize related styles or create reusable code snippets that can be included across different stylesheets. Partials are usually prefixed with an underscore _
, indicating that they are not intended to be compiled into standalone CSS files.
By dividing your stylesheets into partials, you can maintain a cleaner structure and improve collaboration among team members. This approach also makes it easier to manage large projects by allowing you to work on individual components separately.
Creating SCSS Partials and Importing Them
Creating a partial in SCSS is straightforward. You create a new SCSS file and add the underscore prefix to its name. This indicates that it's a partial and will not be compiled into a separate CSS file. Here's an example of creating partials for variables and mixins:
// _variables.scss
$primary-color: #3498db;
$secondary-color: #2ecc71;
// _mixins.scss
@mixin box-shadow {
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
In this example, we've created two partials: _variables.scss
and _mixins.scss
. The _variables.scss
file contains color variables, while the _mixins.scss
file defines a simple mixin for box-shadow.
To import these partials into a main SCSS file, you use the @import
statement. Here's an example of how to import the partials into a main SCSS file:
// main.scss
@import 'variables';
@import 'mixins';
.container {
background-color: $primary-color;
@include box-shadow;
}
In this example, we import the _variables.scss
and _mixins.scss
partials into the main.scss
file. The container
class then uses the imported variables and mixins to apply styles.
Benefits of SCSS Partials and Import Statements
Using partials and import statements in SCSS offers several benefits:
- Modularity: Partials allow you to organize your stylesheets into smaller sections, promoting a modular approach. This makes it easier to manage large projects and collaborate with others.
- Reusability: With partials, you can create reusable code snippets that can be imported into multiple stylesheets. This reduces redundancy and promotes code reuse.
- Maintainability: Dividing stylesheets into partials makes them easier to maintain and update. Changes to a partial are reflected across all stylesheets that import it.
- Flexibility: Partials and import statements give you the flexibility to structure your stylesheets in a way that suits your project. You can create partials for different components, themes, or other logical groupings.
Best Practices for SCSS Partials and Import Statements
To get the most out of SCSS partials and import statements, consider the following best practices:
- Use Descriptive Names: Give your partials descriptive names that reflect their purpose. This makes it easier to understand the structure of your stylesheets.
- Group Related Styles: Organize your partials by grouping related styles. For example, you can create partials for variables, mixins, components, and layout.
- Avoid Deep Nesting: While nesting can be useful, avoid deep nesting of import statements to keep your stylesheets clean and easy to follow.
- Use a Main SCSS File: Create a main SCSS file that imports all your partials. This file should represent the complete structure of your stylesheets.
Conclusion
SCSS partials and import statements are powerful features that help you organize and manage your stylesheets effectively. By breaking down your CSS into smaller, reusable sections, you can promote a modular approach, reduce redundancy, and improve maintainability. Partials are especially useful for large projects where multiple developers are collaborating.
Experiment with partials in your SCSS workflow and explore different ways to structure your stylesheets. With the flexibility that partials and import statements provide, you can create scalable and maintainable CSS for any project.