Introduction to TypeScript: A Guide for JavaScript Developers
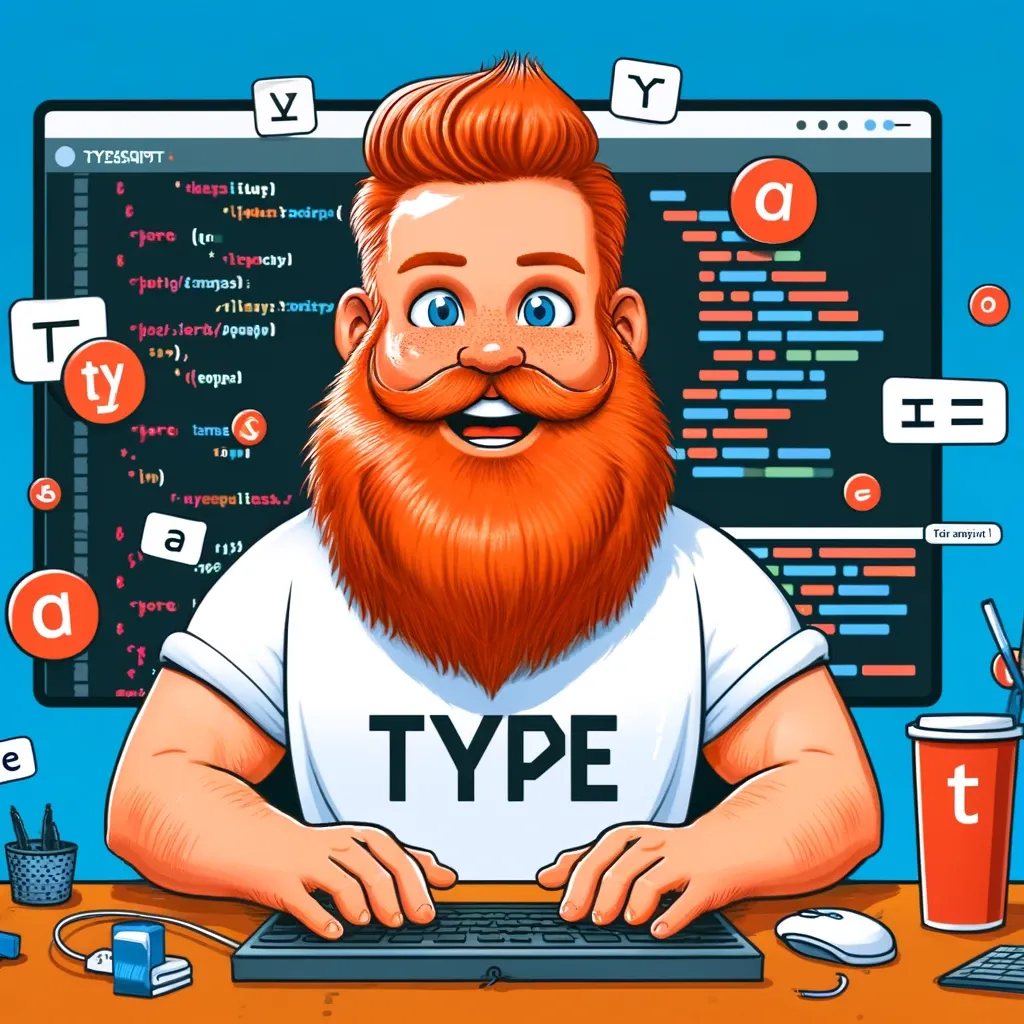
TypeScript has become increasingly popular among developers due to its ability to enhance JavaScript by adding static types. It offers a powerful toolset for building large-scale applications with robust and maintainable code. In this post, we’ll explore what TypeScript is, its benefits, and some basic examples to get you started.
What is TypeScript?
TypeScript is an open-source programming language developed and maintained by Microsoft. It is a strict syntactical superset of JavaScript, which means any valid JavaScript code is also valid TypeScript code. The key feature of TypeScript is its static typing, which allows developers to specify types for variables, function parameters, and return values.
Benefits of TypeScript
- Static Typing: Helps catch errors at compile time rather than at runtime.
- Improved Readability and Maintainability: Makes the code more understandable and easier to maintain, especially in large projects.
- Enhanced IDE Support: Offers better autocompletion, navigation, and refactoring tools.
- Compatibility: Works seamlessly with existing JavaScript libraries and frameworks.
- Scalability: Makes it easier to manage and scale large codebases.
Static Typing
One of the main features that distinguish TypeScript from JavaScript is its static typing system. Static typing means that the type of a variable is known at compile time. This contrasts with dynamic typing, where types are known only at runtime.
What is Static Typing?
In statically typed languages, variables, function parameters, and return values must be declared with specific types. The TypeScript compiler checks these types at compile time to ensure that the types are used consistently throughout the code. If there are any type mismatches or violations, the compiler will raise errors before the code is executed.
Benefits of Static Typing
- Improved Code Quality:
By enforcing type checks, TypeScript ensures that the code adheres to predefined type constraints. This results in cleaner and more predictable code, which is easier to understand and maintain. - Enhanced IDE Support:
With static types, IDEs (Integrated Development Environments) can provide better autocompletion, navigation, and refactoring tools. They can understand the types of variables and functions, offering more accurate and helpful suggestions to the developer. - Documentation and Readability:
Type annotations serve as a form of documentation. They make it clear what types of values a function expects and returns, which helps other developers (or your future self) understand the code more easily. - Early Error Detection:
Static typing allows developers to catch type-related errors early in the development process, during the compilation phase. This can prevent many common bugs that occur due to type mismatches, such as trying to call a method on an undefined variable or performing operations on incompatible types.
let num: number = 5;
num = "hello"; // Error: Type 'string' is not assignable to type 'number'.
Examples of Static Typing
Example 1: Function Parameters and Return Types
In TypeScript, you can specify the types of function parameters and return values. The compiler will enforce these types and raise errors if they are not adhered to.
function multiply(a: number, b: number): number {
return a * b;
}
let result = multiply(2, 3); // Correct usage
let result2 = multiply(2, "three"); // Error: Argument of type 'string' is not assignable to parameter of type 'number'.
Example 2: Variable Types
You can declare variables with specific types. This ensures that the variable can only hold values of the specified type.
let age: number = 30;
age = 31; // Correct usage
age = "thirty-one"; // Error: Type 'string' is not assignable to type 'number'.
Compile-Time vs. Run-Time Errors
Run-Time Errors:
These errors occur during the execution of the program. They can be due to logic errors, unexpected input, or other issues that cannot be detected until the code runs.
let user = { name: "Alice" };
console.log(user.age.toString()); // Run-Time Error: Cannot read property 'toString' of undefined
Compile-Time Errors:
These errors are detected by the TypeScript compiler during the compilation phase. They are usually related to type mismatches, syntax errors, or other issues that can be checked without executing the code.
let userName: string = "Alice";
userName.toUpperCase(); // Correct usage
userName.push("B"); // Error: Property 'push' does not exist on type 'string'.
Static typing in TypeScript provides a robust mechanism for catching errors early, improving code quality, and enhancing the development experience. By enforcing type constraints at compile time, TypeScript helps developers identify and fix potential issues before they make it to production, leading to more reliable and maintainable code.
Improved Readability and Maintainability in TypeScript
One of the significant advantages of using TypeScript is its ability to improve the readability and maintainability of code, especially in large-scale projects. By enforcing type annotations and providing clear interfaces, TypeScript helps developers understand, manage, and maintain their codebases more effectively.
Improved Readability
Readability refers to how easily a developer can understand the code. TypeScript enhances readability through:
- Consistent Coding Practices:
TypeScript encourages consistent coding practices. By adhering to type definitions, the codebase becomes uniform, reducing the cognitive load on developers when switching between different parts of the code. - Self-Documenting Code:
With TypeScript, code becomes self-documenting. The types serve as inline documentation, making it easier for developers to understand the data flow and structure without extensive comments.
interface Product {
id: number;
name: string;
price: number;
}
function printProductDetails(product: Product): void {
console.log(`Product ID: ${product.id}`);
console.log(`Product Name: ${product.name}`);
console.log(`Product Price: ${product.price}`);
}
- Explicit Type Annotations:
Type annotations make it clear what types of values are expected and returned by functions. This reduces ambiguity and helps developers understand the code at a glance.
function getFullName(firstName: string, lastName: string): string {
return `${firstName} ${lastName}`;
}
Improved Maintainability
Maintainability refers to how easily the code can be modified or extended. TypeScript improves maintainability through:
Modular Code Structure:
TypeScript supports modules and namespaces, which promote a modular code structure. This makes it easier to organize and manage large codebases, as related functionality can be grouped together logically.
// math.ts
export function add(a: number, b: number): number {
return a + b;
}
export function subtract(a: number, b: number): number {
return a - b;
}
// main.ts
import { add, subtract } from './math';
console.log(add(10, 5)); // Output: 15
console.log(subtract(10, 5)); // Output: 5
Refactoring Support:
TypeScript's type system makes refactoring safer and more straightforward. When changing a type or refactoring a function, TypeScript will ensure that all usages of the type or function are updated accordingly, reducing the risk of introducing errors.
// Original function
function calculateArea(width: number, height: number): number {
return width * height;
}
// Refactored function
function calculateRectangleArea(rectangle: { width: number, height: number }): number {
return rectangle.width * rectangle.height;
}
let area = calculateRectangleArea({ width: 5, height: 10 });
Error Detection and Prevention:
TypeScript's static typing system catches type-related errors at compile time, preventing many common bugs from making it to production. This leads to more stable and reliable code.
interface User {
id: number;
name: string;
}
function getUser(id: number): User {
// Simulate fetching user data
return { id, name: "Alice" };
}
let user = getUser(1);
console.log(user.age); // Error: Property 'age' does not exist on type 'User'.
Real-World Examples
- Large-Scale Projects:
In large-scale projects, understanding the structure and flow of data is crucial. TypeScript's type system ensures that developers can quickly grasp how data is passed around and manipulated, making it easier to onboard new team members and collaborate effectively. - Library and API Development:
When developing libraries or APIs, providing clear and precise type definitions helps users understand how to interact with the library or API. TypeScript's type declarations act as an accurate and reliable form of documentation.
interface ApiResponse {
status: number;
data: any;
}
function fetchUserData(userId: number): Promise<ApiResponse> {
// Simulate an API call
return new Promise((resolve) => {
resolve({ status: 200, data: { id: userId, name: "Alice" } });
});
}
fetchUserData(1).then(response => {
console.log(response.data.name); // Output: Alice
});
TypeScript significantly enhances the readability and maintainability of code by providing explicit type annotations, supporting consistent coding practices, and offering robust error detection and refactoring support. These features are especially beneficial in large-scale projects, where managing complexity and ensuring code quality are paramount.
By adopting TypeScript, development teams can create more understandable, maintainable, and reliable codebases, leading to more efficient and successful projects.
Enhanced IDE Support in TypeScript
One of the standout features of TypeScript is its ability to significantly enhance the development experience through improved IDE (Integrated Development Environment) support. TypeScript's static typing and type annotations enable IDEs to provide powerful features such as autocompletion, navigation, and refactoring tools, making development faster and more efficient.
Autocompletion
Autocompletion is a feature that suggests possible completions for partially typed code. This helps developers write code more quickly and with fewer errors. TypeScript's type system allows IDEs to provide accurate autocompletion suggestions based on the types defined in the code.
Example
interface User {
id: number;
name: string;
email: string;
}
let user: User = {
id: 1,
name: "Alice",
email: "alice@example.com"
};
// When you type 'user.' the IDE will suggest 'id', 'name', and 'email'.
console.log(user.name);
In this example, when you type user.
the IDE will automatically suggest the properties id
, name
, and email
, reducing the chance of typos and speeding up the coding process.
Navigation
Navigation features in IDEs allow developers to quickly jump to the definitions of types, functions, and variables. This is particularly useful in large codebases where finding the definition of a particular item can be time-consuming.
Example
interface Product {
id: number;
name: string;
price: number;
}
function getProduct(): Product {
return { id: 1, name: "Laptop", price: 1000 };
}
let product = getProduct();
// Ctrl+Click or Cmd+Click on 'getProduct' to navigate to its definition.
In this example, you can use navigation features (e.g., Ctrl+Click or Cmd+Click) to jump directly to the definition of the getProduct
function, making it easier to understand and trace the code flow.
Refactoring Tools
Refactoring tools help developers restructure their code without changing its external behavior. TypeScript's type system enables IDEs to provide powerful refactoring capabilities, such as renaming variables, extracting methods, and more.
Example
class Person {
firstName: string;
lastName: string;
constructor(firstName: string, lastName: string) {
this.firstName = firstName;
this.lastName = lastName;
}
getFullName(): string {
return `${this.firstName} ${this.lastName}`;
}
}
let person = new Person("John", "Doe");
// Rename 'firstName' to 'givenName' using IDE refactoring tools.
In this example, if you want to rename the firstName
property to givenName
, you can use the IDE's refactoring tool. The IDE will automatically update all occurrences of firstName
to givenName
throughout the codebase, ensuring consistency and saving time.
Real-World Benefits
- Increased Productivity:
Enhanced IDE support makes developers more productive by reducing the time spent on repetitive tasks. Features like autocompletion and navigation allow developers to write code more quickly and accurately. - Error Reduction:
Accurate autocompletion and type checking help reduce errors. Developers are less likely to make typos or use incorrect types, resulting in more reliable code. - Simplified Code Maintenance:
Refactoring tools make it easier to update and maintain code. Developers can confidently rename variables, extract methods, and perform other refactoring operations knowing that the IDE will handle all necessary updates. - Better Code Understanding:
Navigation features help developers understand codebases more quickly. By easily jumping to definitions and references, developers can trace the flow of data and logic, improving their understanding of the code.
Popular IDEs with TypeScript Support
Several popular IDEs offer excellent TypeScript support, including:
- Visual Studio Code (VSCode):
VSCode is a free, open-source IDE developed by Microsoft. It has robust TypeScript support, including features like IntelliSense, autocompletion, navigation, and refactoring tools. - WebStorm:
WebStorm is a commercial IDE developed by JetBrains. It provides advanced TypeScript support with powerful refactoring tools, code navigation, and debugging capabilities.
TypeScript's enhanced IDE support offers significant benefits to developers by providing powerful autocompletion, navigation, and refactoring tools. These features increase productivity, reduce errors, simplify code maintenance, and help developers better understand their codebases. By leveraging these capabilities, developers can create more robust and maintainable applications with greater efficiency.
Compatibility in TypeScript
One of the key strengths of TypeScript is its compatibility with existing JavaScript libraries and frameworks. This makes it easy to integrate TypeScript into existing projects and leverage the vast ecosystem of JavaScript tools and libraries.
How TypeScript Ensures Compatibility
- Superset of JavaScript: TypeScript is a strict syntactical superset of JavaScript, which means any valid JavaScript code is also valid TypeScript code. This allows developers to gradually adopt TypeScript without having to rewrite their entire codebase.
// Valid JavaScript code
function greet(name) {
return `Hello, ${name}!`;
}
// Also valid TypeScript code
function greet(name: string): string {
return `Hello, ${name}!`;
}
- Type Definitions:
TypeScript uses declaration files (with a.d.ts
extension) to provide type information about existing JavaScript libraries. These files describe the shape of the library's API and can be used by the TypeScript compiler to check types at compile time.
- DefinitelyTyped: The DefinitelyTyped project is a community-driven repository of TypeScript type definitions for popular JavaScript libraries. Developers can easily install these type definitions using npm.
npm install @types/jquery
Example usage with jQuery:
import * as $ from 'jquery';
$(document).ready(() => {
$('body').append('<p>Hello, TypeScript!</p>');
});
- Interop with JavaScript:
TypeScript provides several features to interoperate with JavaScript, such asany
type, type assertions, anddeclare
keyword. This makes it easy to call JavaScript functions and use JavaScript libraries in TypeScript code.
- Using
any
Type: Theany
type allows developers to opt out of type checking for specific variables, enabling compatibility with dynamic JavaScript code
let someLibrary: any = require('some-library');
someLibrary.doSomething();
- Type Assertions:
Type assertions allow developers to tell the TypeScript compiler to treat a value as a specific type. This is useful when integrating with JavaScript code that may not have type definitions.
let someValue: any = "Hello, TypeScript!";
let strLength: number = (someValue as string).length;
- Declare Keyword: The
declare
keyword is used to tell TypeScript about the existence of global variables or functions defined in JavaScript libraries.
declare var GLOBAL_VAR: string;
console.log(GLOBAL_VAR);
Real-World Examples
Interoperating with Legacy JavaScript:
TypeScript can be incrementally adopted in legacy JavaScript projects. Developers can start by renaming .js
files to .ts
and adding type annotations gradually.
// Old JavaScript code
function add(a, b) {
return a + b;
}
// Updated TypeScript code
function add(a: number, b: number): number {
return a + b;
}
Using Node.js Libraries:
TypeScript is fully compatible with Node.js, allowing developers to write type-safe server-side code. TypeScript can use existing Node.js libraries with the help of type definitions.
import * as express from 'express';
const app = express();
app.get('/', (req, res) => {
res.send('Hello, TypeScript with Express!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Integrating with React:
TypeScript works seamlessly with React, a popular JavaScript library for building user interfaces. With TypeScript, developers can write type-safe React components and catch errors at compile time.
import * as React from 'react';
interface Props {
name: string;
}
const HelloWorld: React.FC<Props> = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default HelloWorld;
TypeScript's compatibility with existing JavaScript libraries and frameworks makes it an excellent choice for both new and existing projects. By being a superset of JavaScript and offering robust type definition support, TypeScript allows developers to seamlessly integrate with the vast JavaScript ecosystem. This ensures that you can enjoy the benefits of type safety and enhanced development experience without sacrificing compatibility with your favorite libraries and tools.
Scalability in TypeScript
Scalability is a crucial factor in software development, especially as projects grow in size and complexity. TypeScript provides several features that make it easier to manage and scale large codebases, ensuring that applications remain maintainable and performant as they evolve.
How TypeScript Enhances Scalability
Code Navigation and Documentation:
TypeScript enhances code navigation and documentation through type annotations and interfaces. Developers can easily navigate to the definitions of types, functions, and variables, improving their understanding of the codebase.
interface Product {
id: number;
name: string;
price: number;
}
function getProduct(id: number): Product {
return { id, name: "Laptop", price: 1000 };
}
let product = getProduct(1);
console.log(product.name);
// Ctrl+Click or Cmd+Click on 'Product' to navigate to its definition.
Refactoring Support:
TypeScript's type system makes refactoring easier and safer. IDEs can leverage TypeScript's type information to provide advanced refactoring tools, such as renaming variables, extracting methods, and more.
class User {
constructor(public id: number, public name: string) {}
}
function createUser(id: number, name: string): User {
return new User(id, name);
}
let user = createUser(1, "Alice");
console.log(user.name);
// Rename 'name' to 'fullName' using IDE refactoring tools.
Interfaces and Type Aliases:
Interfaces and type aliases in TypeScript provide a way to define the structure of objects and complex types. This ensures that different parts of the codebase adhere to the same contract, making the code more consistent and easier to refactor.
interface Address {
street: string;
city: string;
zipcode: string;
}
interface User {
id: number;
name: string;
address: Address;
}
function getUserAddress(user: User): Address {
return user.address;
}
let user: User = {
id: 1,
name: "Alice",
address: { street: "123 Main St", city: "Wonderland", zipcode: "12345" }
};
console.log(getUserAddress(user));
Modular Code Structure:
TypeScript supports modules, which promote a modular code structure. This allows developers to break down the codebase into smaller, reusable components, making it easier to manage and understand.
// user.ts
export interface User {
id: number;
name: string;
email: string;
}
// userService.ts
import { User } from './user';
export function getUser(id: number): User {
return { id, name: "Alice", email: "alice@example.com" };
}
// main.ts
import { getUser } from './userService';
let user = getUser(1);
console.log(user.name);
Static Typing:
Static typing helps catch errors early in the development process, reducing the likelihood of bugs in production. By ensuring that variables, functions, and objects are used consistently, TypeScript makes the codebase more predictable and easier to understand.
interface User {
id: number;
name: string;
email: string;
}
function getUser(id: number): User {
return { id, name: "Alice", email: "alice@example.com" };
}
let user = getUser(1);
console.log(user.name);
Real-World Examples
- Enterprise Applications:
In large enterprise applications, maintaining consistency and managing complexity are critical. TypeScript's static typing and modular architecture help enforce consistent patterns and practices across the codebase. - Team Collaboration:
TypeScript facilitates better collaboration among team members by providing clear type definitions and interfaces. This reduces misunderstandings and makes it easier for new developers to onboard and contribute to the project.
Library and API Development:
When developing libraries or APIs, TypeScript ensures that the public API is well-defined and consistent. Consumers of the library can rely on the provided types, making integration smoother and reducing the likelihood of misuse.
interface ApiResponse<T> {
status: number;
data: T;
}
function fetchData<T>(url: string): Promise<ApiResponse<T>> {
return fetch(url).then(response => response.json());
}
fetchData<User>("/api/user/1").then(response => {
console.log(response.data.name);
});
TypeScript significantly enhances the scalability of codebases by providing static typing, modular code structure, robust refactoring tools, and improved code navigation. These features make it easier to manage and maintain large applications, ensuring that they remain performant and reliable as they grow. By adopting TypeScript, development teams can build scalable and maintainable software that can adapt to changing requirements and evolving complexity.
Getting Started with TypeScript
Installation
You can install TypeScript via npm:
npm install -g typescript
Basic Example
Let's start with a basic example. Here is a simple TypeScript program that adds two numbers:
function add(a: number, b: number): number {
return a + b;
}
let sum = add(5, 3);
console.log(`Sum: ${sum}`);
Explanation
- Function Definition: The
add
function takes two parameters,a
andb
, both of typenumber
, and returns anumber
. - Type Annotations: The
: number
syntax is used to annotate the types of parameters and the return type. - Variable Declaration: The
let sum
line declares a variablesum
and assigns it the result of theadd
function.
Advanced Features
Interfaces
Interfaces in TypeScript are used to define the shape of an object. Here’s an example:
interface Person {
firstName: string;
lastName: string;
age: number;
}
function greet(person: Person): string {
return `Hello, ${person.firstName} ${person.lastName}! You are ${person.age} years old.`;
}
let user = { firstName: "John", lastName: "Doe", age: 30 };
console.log(greet(user));
Classes
TypeScript also supports object-oriented programming with classes. Here’s an example:
class Animal {
name: string;
constructor(name: string) {
this.name = name;
}
move(distance: number = 0) {
console.log(`${this.name} moved ${distance} meters.`);
}
}
class Dog extends Animal {
bark() {
console.log('Woof! Woof!');
}
}
let myDog = new Dog('Rex');
myDog.bark();
myDog.move(10);
Generics
Generics provide a way to create reusable components. Here’s a simple example:
function identity<T>(arg: T): T {
return arg;
}
let output1 = identity<string>("Hello");
let output2 = identity<number>(42);
console.log(output1); // Output: Hello
console.log(output2); // Output: 42
Conclusion
TypeScript is a powerful tool that can greatly improve your JavaScript development experience. By adding static types, you can catch errors early, improve code readability, and maintainability, and leverage advanced IDE features. Whether you are building small applications or large-scale projects, TypeScript provides a solid foundation for writing robust and scalable code.