Introduction to Scala: The Scalable Language for Modern Developers
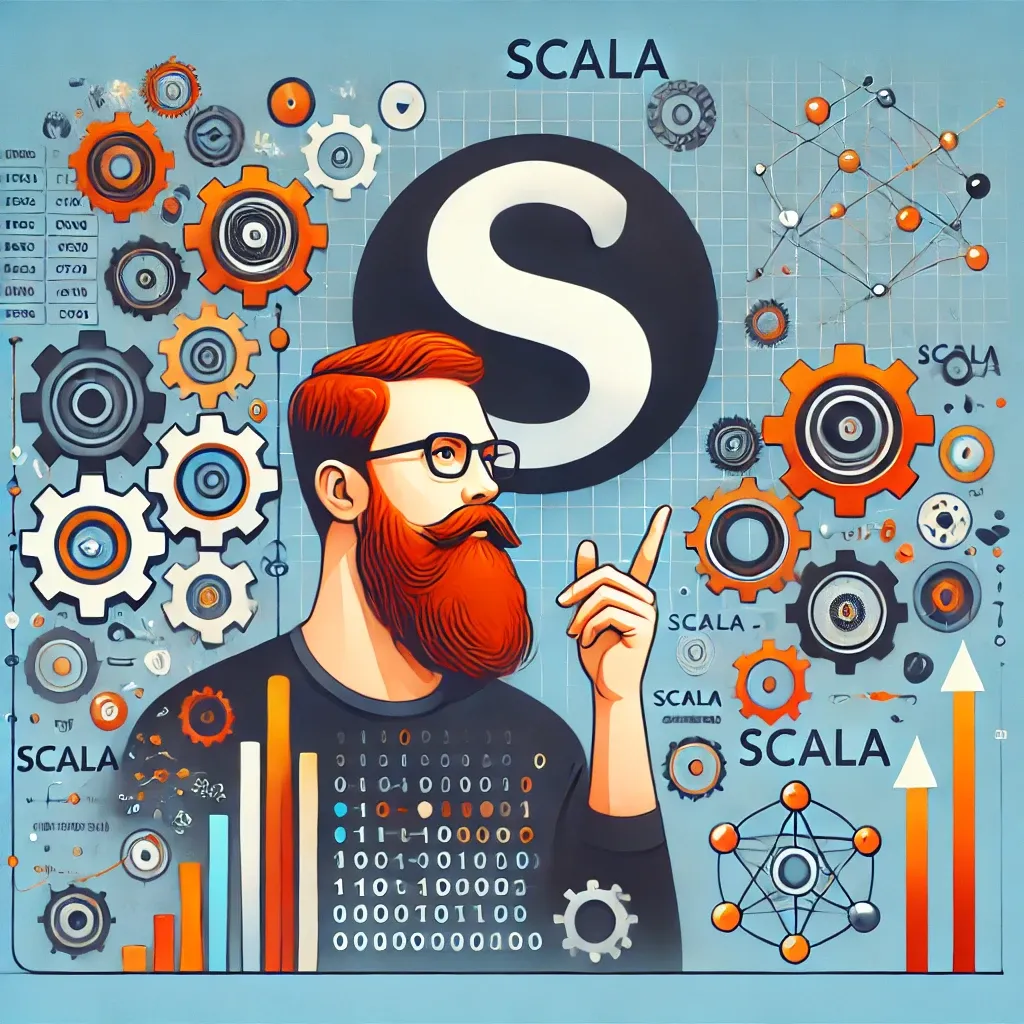
Scala, short for "scalable language," is a powerful and flexible programming language that combines the best features of both object-oriented and functional programming paradigms. Since its inception in 2003 by Martin Odersky, Scala has been steadily gaining popularity, especially in industries that require highly concurrent and scalable applications, such as finance, data engineering, and telecommunications.
In this blog post, we will explore what makes Scala unique, its key features, and why it might be the right choice for your next project.
Why Scala?
1. Combination of Object-Oriented and Functional Programming
Scala seamlessly integrates object-oriented and functional programming into one concise, high-level language. This dual nature allows developers to leverage the strengths of both paradigms:
Object-Oriented
Scala fully supports object-oriented programming, allowing you to create classes, objects, and traits (Scala's version of interfaces).
Here's an example of how you might use these features:
// Define a class with properties and methods
class Animal(val name: String, val sound: String) {
def makeSound(): Unit = {
println(s"The $name goes '$sound'")
}
}
// Define a trait (similar to an interface in Java)
trait Pet {
def ownerName: String
}
// Extend the Animal class and mix in the Pet trait
class Dog(name: String, owner: String) extends Animal(name, "bark") with Pet {
def ownerName: String = owner
}
// Create an instance of Dog and use it
val myDog = new Dog("Buddy", "John")
myDog.makeSound() // Output: The Buddy goes 'bark'
println(s"${myDog.name} is owned by ${myDog.ownerName}") // Output: Buddy is owned by John
Explanation:
- Class Definition:
Animal
is a class with two properties,name
andsound
, and a methodmakeSound
. - Trait:
Pet
is a trait that defines a contract for any class that mixes it in. - Inheritance and Composition:
Dog
extends theAnimal
class and mixes in thePet
trait, demonstrating both inheritance and composition.
Functional
Scala treats functions as first-class citizens, meaning you can pass them as arguments, return them from other functions, and assign them to variables.
Here's an example that highlights the functional programming approach:
// Define a function that applies a given function to a list of numbers
def applyOperation(numbers: List[Int], operation: Int => Int): List[Int] = {
numbers.map(operation)
}
// Define a function that doubles a number
val double: Int => Int = _ * 2
// Use the applyOperation function with the double function
val result = applyOperation(List(1, 2, 3, 4, 5), double)
println(result) // Output: List(2, 4, 6, 8, 10)
Explanation:
- Higher-Order Functions:
applyOperation
is a higher-order function that takes another function (operation
) as a parameter and applies it to each element in the numbers list. - Anonymous Functions: The function
double
is defined using a concise lambda expression_ * 2
. - Immutability: The list
result
is immutable, which is a common practice in functional programming to avoid side effects.
2. Statically Typed Yet Concise
Scala's type system is powerful, but the language also provides mechanisms to keep your code concise.
Here’s an example demonstrating concise statically-typed code in Scala.
// Define a list of integers with type inference
val numbers = List(1, 2, 3, 4, 5)
// Define a function with type inference
val sum = numbers.reduce(_ + _)
println(sum) // Output: 15
// Explicit types
val explicitNumbers: List[Int] = List(1, 2, 3, 4, 5)
val explicitSum: Int = explicitNumbers.reduce((a: Int, b: Int) => a + b)
println(explicitSum) // Output: 15
Explanation:
- Type Inference: In Scala, you don't need to specify types explicitly; the compiler infers them. This makes the code more concise.
- Concise Syntax: The function
_ + _
is a shorthand for a lambda function that takes two arguments and returns their sum.
Java Example (Verbose Alternative):
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Verbose list definition
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Verbose sum calculation
int sum = 0;
for (int number : numbers) {
sum += number;
}
System.out.println(sum); // Output: 15
}
}
Explanation:
- Explicit Typing: Java requires explicit types, which can make the code more verbose.
- Imperative Loop: The sum is calculated using a for-loop, which is more verbose compared to Scala’s functional approach.
Contrasting Example of Non-Concise, Verbose, and Dynamic Typing (in Python)
Here’s a similar example in Python to show how dynamic typing can lead to different practices:
# Dynamic typing allows for implicit type assignment
numbers = [1, 2, 3, 4, 5]
# Using a loop for summation (less concise than Scala's reduce)
sum = 0
for number in numbers:
sum += number
print(sum) # Output: 15
Explanation:
- Dynamic Typing: Python doesn’t require explicit type declarations, which can make the code concise but potentially error-prone if types are not what you expect.
- Less Functional: The imperative style with a loop is less concise compared to Scala's functional approach using reduce.
These examples highlight how Scala allows you to write powerful, concise, and maintainable code using both object-oriented and functional paradigms while maintaining the safety and performance benefits of static typing.
3. Interoperability with Java
One of Scala's most significant advantages is its seamless interoperability with Java. Scala runs on the Java Virtual Machine (JVM), which means you can use all existing Java libraries and frameworks. This feature makes Scala an attractive option for teams transitioning from Java to a more modern language.
4. Concurrency Support
In modern computing, efficiently handling multiple tasks at once (concurrency) is crucial, especially in applications like web servers, real-time data processing, and complex simulations. Scala excels in this area by providing high-level abstractions for concurrency, allowing developers to write code that is both expressive and safe from common concurrency pitfalls like race conditions.
Futures
A Future
in Scala represents a computation that may complete at some point in the future, either successfully or with an error. It allows you to perform asynchronous, non-blocking operations and easily manage the result once it's available.
Basic Example of a Future:
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
// Define a Future that performs an asynchronous computation
val future = Future {
Thread.sleep(1000) // Simulate a long-running task
42 // Return the result
}
// Handle the result once the future is completed
future.onComplete {
case scala.util.Success(value) => println(s"Success: $value")
case scala.util.Failure(exception) => println(s"Failed with exception: $exception")
}
// Output (after 1 second): Success: 42
Explanation:
- Asynchronous Computation: The
Future
wraps a block of code that will be executed asynchronously. - Non-blocking: The main program continues to execute without waiting for the
Future
to complete. - Completion Handling: The
onComplete
method is used to handle the result of theFuture
once it's done, allowing you to react to both success and failure.
Composing Futures
Futures can be easily composed, allowing you to chain multiple asynchronous operations together.
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
val future1 = Future { Thread.sleep(500); 10 }
val future2 = Future { Thread.sleep(500); 32 }
// Combine the results of two futures
val combinedFuture = for {
result1 <- future1
result2 <- future2
} yield result1 + result2
combinedFuture.onComplete {
case scala.util.Success(value) => println(s"Combined Result: $value")
case scala.util.Failure(exception) => println(s"Failed with exception: $exception")
}
// Output (after 1 second): Combined Result: 42
Explanation:
- Future Composition: The
for-comprehension
is used to compose multiple futures, allowing you to wait for both to complete and then combine their results. - Non-blocking: Even though the computation waits for both futures, it remains non-blocking.
The Actor Model (with Akka)
The Actor model is another powerful abstraction for managing concurrency in Scala. It’s a design pattern that helps you manage state and behavior in concurrent systems without worrying about low-level thread management.
Introduction to Akka Actors:
Akka is a popular toolkit in Scala for building concurrent, distributed, and resilient message-driven applications using the Actor model. An Actor in Akka is an independent entity that encapsulates state and behavior. Actors communicate by exchanging messages asynchronously, ensuring that no two actors share state directly, thus avoiding race conditions.
import akka.actor.{Actor, ActorSystem, Props}
// Define an Actor class
class Counter extends Actor {
var count = 0
def receive: Receive = {
case "increment" => count += 1
case "get" => sender() ! count
}
}
// Create an ActorSystem and an instance of the Counter actor
val system = ActorSystem("CounterSystem")
val counter = system.actorOf(Props[Counter], "counter")
// Send messages to the counter actor
counter ! "increment"
counter ! "increment"
counter ! "get"
// Shutdown the actor system
system.terminate()
Explanation:
- Actor Definition: The
Counter
actor maintains an internal state (count
). It can receive messages and modify its state or reply to the sender. - Message Passing: Actors communicate exclusively through messages, which helps in achieving concurrency without the risks associated with shared mutable state.
- Actor System:
ActorSystem
is the environment in which actors live. It manages the lifecycle and execution of actors.
Scaling with Akka Actors:
Actors can easily be distributed across multiple nodes, making it possible to build highly scalable systems. Akka takes care of the complexities of networking, ensuring that messages are reliably delivered even in distributed environments.
import akka.actor.{Actor, ActorSystem, Props}
// Define a message protocol
case class Increment(value: Int)
case object GetCount
// Define an Actor with more complex behavior
class AdvancedCounter extends Actor {
var count = 0
def receive: Receive = {
case Increment(value) => count += value
case GetCount => sender() ! count
}
}
// Create an ActorSystem and the AdvancedCounter actor
val system = ActorSystem("AdvancedCounterSystem")
val counter = system.actorOf(Props[AdvancedCounter], "advancedCounter")
// Interact with the AdvancedCounter actor
counter ! Increment(10)
counter ! Increment(5)
counter ! GetCount
// Shutdown the actor system
system.terminate()
Explanation:
- Custom Messages: The
AdvancedCounter
actor handles custom messages (Increment
andGetCount
), allowing more flexible interaction. - Concurrency Management: Akka’s message-passing model ensures that all operations are handled sequentially within an actor, avoiding race conditions.
When to Use Futures vs. Actors
- Futures: Ideal for simple, stateless asynchronous computations where you need to perform a task in the background and eventually retrieve a result.
- Actors: Best suited for scenarios where you need to manage state across multiple concurrent tasks or when building complex, distributed systems that require fault tolerance and resilience.
5. Advanced Type System
Scala’s type system goes beyond the basics of static typing. It includes features like:
- Case Classes: For immutable data structures.
- Pattern Matching: For concise and readable code.
- Traits: To create modular and reusable code components.
These features empower developers to write robust, maintainable, and error-free code.
Use Cases for Scala
Scala shines in several areas, making it a go-to language for various types of projects:
- Big Data Processing: Scala is the language of choice for Apache Spark, a leading framework for big data processing.
- Web Development: Frameworks like Play provide a solid foundation for building scalable web applications.
- Data Science: With libraries like Breeze and Spire, Scala is becoming increasingly popular in data science and machine learning circles.
- Reactive Systems: The Akka framework, built in Scala, is ideal for creating highly concurrent, distributed, and fault-tolerant systems.
Getting Started with Scala
If you're interested in diving into Scala, here are a few steps to get started:
- Install Scala: You can install Scala through the official website or use a build tool like sbt (Scala Build Tool).
- Set Up Your Environment: Consider using an IDE like IntelliJ IDEA, which has excellent support for Scala.
- Learn the Basics: There are plenty of free resources, including the official Scala documentation, to help you get started.
- Build Projects: Start with simple projects and gradually move on to more complex ones, leveraging both object-oriented and functional programming.
Conclusion
Scala is a powerful, versatile language that caters to modern development needs, especially in areas requiring scalability and concurrency. Whether you're a Java developer looking to explore functional programming or a data engineer working with big data, Scala offers the tools and flexibility you need to succeed.