Introduction to Ruby on Rails Action Cable
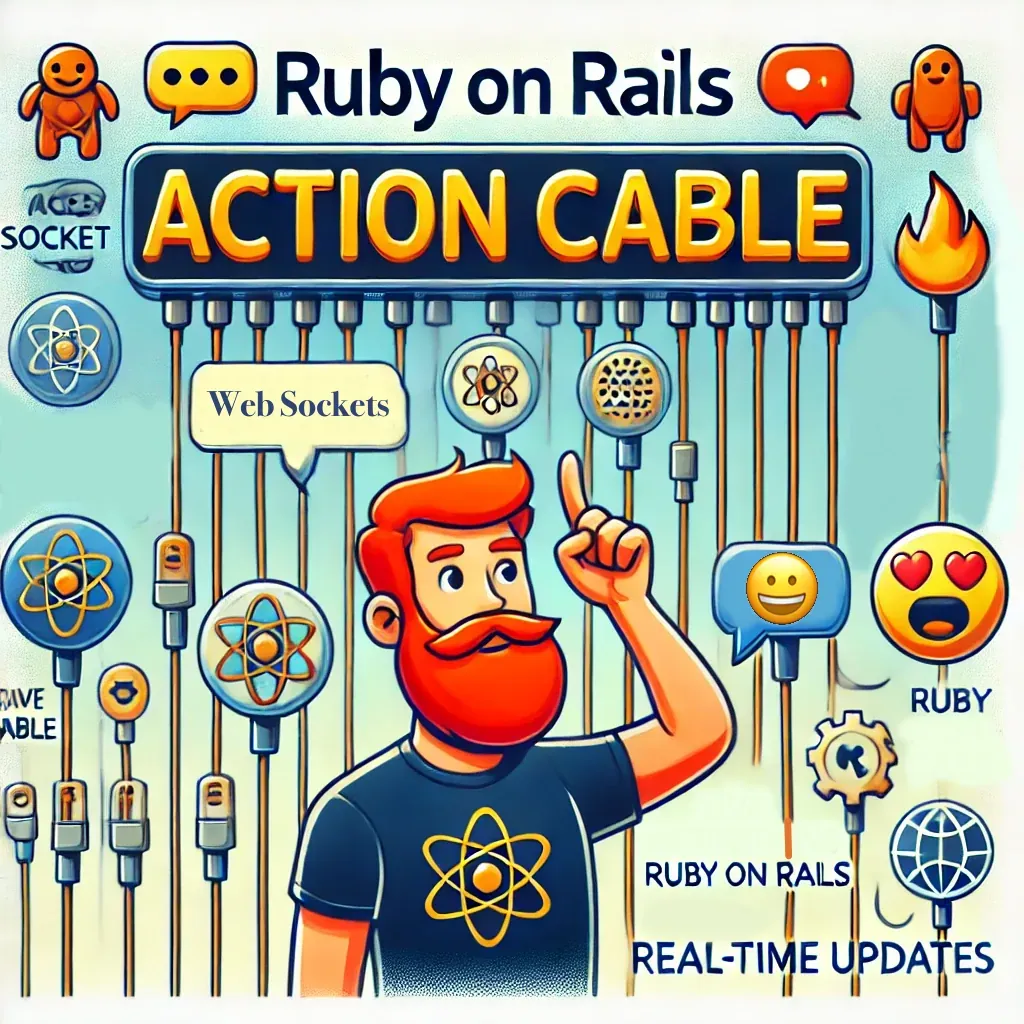
Ruby on Rails has long been a popular framework for web development due to its simplicity and robustness. One of the features that enhance its capability is Action Cable, a framework that integrates WebSockets with Rails. This allows developers to create real-time applications seamlessly. In this blog post, we'll dive into what Action Cable is, how it works, and how you can start using it in your Rails applications.
What is Action Cable?
Action Cable is a WebSocket framework that comes with Ruby on Rails. It allows you to handle real-time features in your Rails applications, such as live notifications, chat applications, and live updates. By integrating WebSockets with Rails, Action Cable enables full-duplex communication channels over a single TCP connection, providing a more interactive experience for users.
Key Features of Action Cable:
- Seamless Integration: Works directly with Rails, making it easy to add real-time features without extensive configuration.
- Scalability: Can handle multiple connections efficiently and integrates with background job processing for offloading tasks.
- Security: Comes with built-in authentication mechanisms to ensure secure connections.
Setting Up Action Cable
To get started with Action Cable, you need to follow these steps:
1. Create a New Rails Application
If you don’t already have a Rails application, you can create one by running:
rails new myapp
cd myapp
2. Generate a Channel
Channels are the cornerstone of Action Cable. They act like controllers in your typical Rails MVC structure. Generate a new channel using the Rails generator:
rails generate channel Chat
This command will create a new channel file in app/channels/chat_channel.rb
and a corresponding CoffeeScript file in app/assets/javascripts/channels/chat.coffee
.
3. Define the Channel Logic
Edit the ChatChannel
to define what happens when a user subscribes or performs actions. For example:
# app/channels/chat_channel.rb
class ChatChannel < ApplicationCable::Channel
def subscribed
stream_from "chat_#{params[:room]}"
end
def unsubscribed
# Any cleanup needed when channel is unsubscribed
end
def speak(data)
ActionCable.server.broadcast "chat_#{params[:room]}", message: data['message']
end
end
4. Client-Side Subscription
Edit the chat.coffee
file to handle the client-side subscription:
# app/assets/javascripts/channels/chat.coffee
App.chat = App.cable.subscriptions.create { channel: "ChatChannel", room: "BestRoom" },
connected: ->
# Called when the subscription is ready for use on the server
disconnected: ->
# Called when the subscription has been terminated by the server
received: (data) ->
# Called when there's incoming data on the websocket for this channel
$('#messages').append "<p>#{data.message}</p>"
speak: (message) ->
@perform 'speak', message: message
5. Broadcasting Messages
To broadcast messages to the channel, you can use the following command in a Rails controller:
# app/controllers/messages_controller.rb
class MessagesController < ApplicationController
def create
@message = Message.create!(message_params)
ActionCable.server.broadcast "chat_BestRoom", message: @message.content
end
private
def message_params
params.require(:message).permit(:content)
end
end
6. Add a Front-End Form
Create a form in your view to send messages:
<!-- app/views/messages/index.html.erb -->
<h1>Chat Room</h1>
<div id="messages">
<!-- Messages will appear here -->
</div>
<form id="new_message" action="/messages" method="post">
<input type="text" id="message_content" name="message[content]">
<input type="submit" value="Send">
</form>
<%= javascript_include_tag "application" %>
7. Enabling Redis
For production environments, it’s recommended to use Redis as the Action Cable adapter. You can set it up by adding redis
gem to your Gemfile:
gem 'redis', '~> 4.0'
And configure it in cable.yml
:
# config/cable.yml
production:
adapter: redis
url: redis://localhost:6379/1
channel_prefix: myapp_production
Conclusion
Action Cable is a powerful feature of Ruby on Rails that allows developers to add real-time capabilities to their applications with ease. By following the steps outlined in this post, you can start integrating WebSockets into your Rails projects and create dynamic, interactive web applications.