Getting Started with Express.js: A Simple and Efficient Node.js Framework
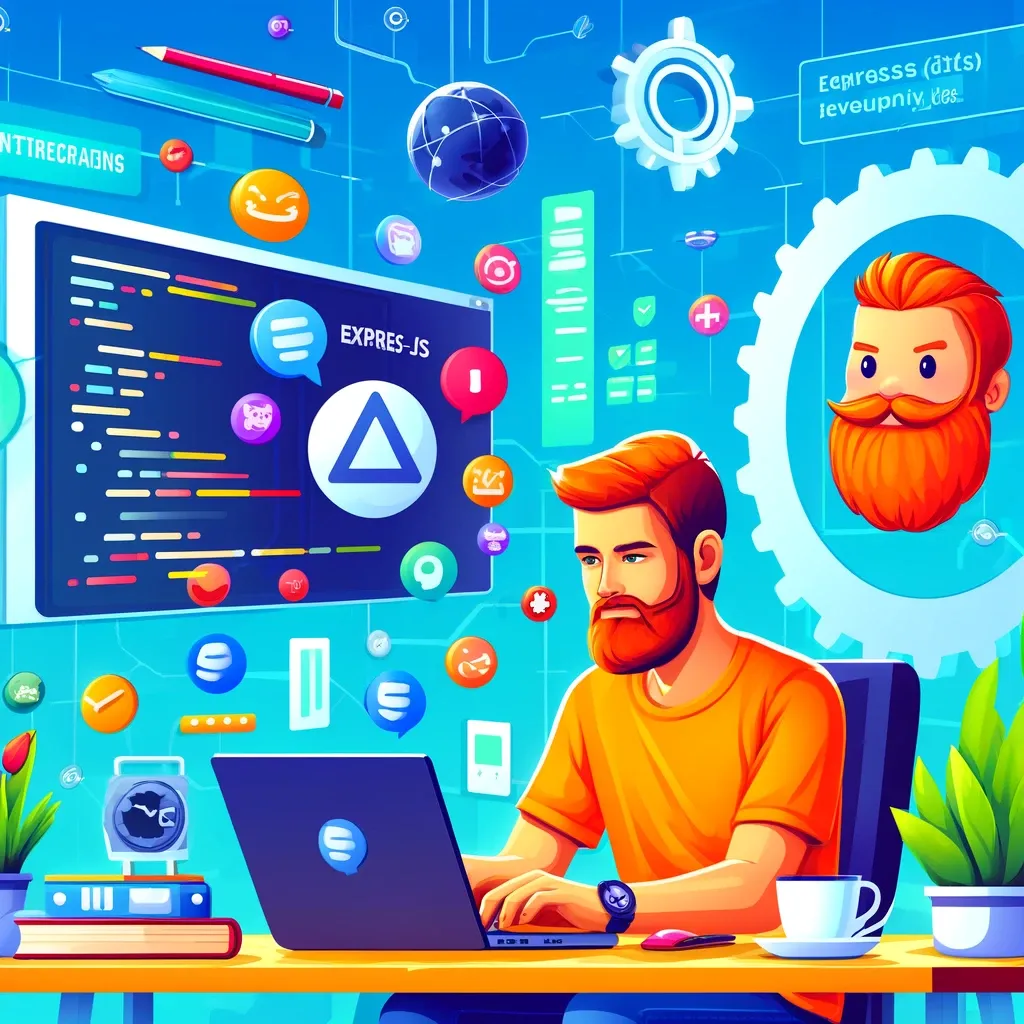
Express.js is a fast, unopinionated, minimalist web framework for Node.js, designed to build web applications and APIs with ease. In this blog post, we'll cover the basics of setting up an Express.js project, creating a simple API, and explore some real-world use cases and examples.
What is Express.js?
Express.js is a lightweight web framework that provides robust features for building web and mobile applications. It simplifies the development process by providing a thin layer of fundamental web application features without obscuring Node.js features.
Why Use Express.js?
- Minimal and Flexible: It provides a thin layer of fundamental web application features, leaving the core Node.js features intact.
- Robust Routing: Includes a powerful routing API that makes handling different HTTP requests easy.
- Middleware Support: Allows the use of middleware to handle various tasks like parsing request bodies, managing sessions, etc.
- Performance: Being a lightweight framework, it offers high performance for web applications and APIs.
Setting Up Express.js
Let's walk through setting up a basic Express.js application.
Step 1: Install Node.js and npm
Before getting started, ensure you have Node.js and npm (Node Package Manager) installed. You can download them from Node.js official website.
Step 2: Initialize a New Node.js Project
Create a new directory for your project and navigate into it using the terminal. Then, initialize a new Node.js project with the following command:
npm init -y
This command creates a package.json
file with default settings.
Step 3: Install Express.js
Next, install Express.js using npm:
npm install express
Step 4: Create Your First Express Application
Create a new file named app.js
and add the following code:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Example app listening at http://localhost:${port}`);
});
This code sets up a basic Express server that responds with "Hello World!" when accessed at the root URL.
Step 5: Run Your Application
Start your Express application by running the following command in your terminal:
node app.js
You should see the message Example app listening at http://localhost:3000
in your terminal. Open your web browser and navigate to http://localhost:3000
to see the "Hello World!" message.
Adding Routes
Express makes it easy to define routes for your application. Let's add a few more routes to demonstrate this:
app.get('/about', (req, res) => {
res.send('About Page');
});
app.get('/contact', (req, res) => {
res.send('Contact Page');
});
With these routes added, you can visit http://localhost:3000/about
and http://localhost:3000/contact
to see the respective messages.
Middleware in Express.js
Middleware functions are functions that have access to the request object (req
), the response object (res
), and the next middleware function in the application’s request-response cycle. Middleware can execute code, modify the request and response objects, end the request-response cycle, and call the next middleware function.
Example of Middleware
Here's a simple example of middleware that logs the current timestamp for each request:
app.use((req, res, next) => {
console.log('Time:', Date.now());
next();
});
Real-World Use Cases and Examples
1. Building a RESTful API
Express.js is commonly used to build RESTful APIs. Let's create a simple API for managing a list of books.
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
let books = [
{ id: 1, title: '1984', author: 'George Orwell' },
{ id: 2, title: 'To Kill a Mockingbird', author: 'Harper Lee' }
];
// Get all books
app.get('/books', (req, res) => {
res.json(books);
});
// Get a single book by ID
app.get('/books/:id', (req, res) => {
const book = books.find(b => b.id === parseInt(req.params.id));
if (!book) return res.status(404).send('Book not found');
res.json(book);
});
// Add a new book
app.post('/books', (req, res) => {
const book = {
id: books.length + 1,
title: req.body.title,
author: req.body.author
};
books.push(book);
res.status(201).json(book);
});
// Update a book
app.put('/books/:id', (req, res) => {
const book = books.find(b => b.id === parseInt(req.params.id));
if (!book) return res.status(404).send('Book not found');
book.title = req.body.title;
book.author = req.body.author;
res.json(book);
});
// Delete a book
app.delete('/books/:id', (req, res) => {
const bookIndex = books.findIndex(b => b.id === parseInt(req.params.id));
if (bookIndex === -1) return res.status(404).send('Book not found');
const deletedBook = books.splice(bookIndex, 1);
res.json(deletedBook);
});
app.listen(port, () => {
console.log(`Book API listening at http://localhost:${port}`);
});
2. Creating a Static File Server
Express.js can serve static files such as HTML, CSS, and JavaScript files.
const express = require('express');
const app = express();
const port = 3000;
app.use(express.static('public'));
app.listen(port, () => {
console.log(`Static file server running at http://localhost:${port}`);
});
Place your static files (e.g., index.html
, style.css
, script.js
) in the public
directory, and they will be accessible at http://localhost:3000
.
3. Building a Simple Authentication System
Express.js can be used to build a simple authentication system.
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
const users = [
{ id: 1, username: 'user1', password: 'password1' },
{ id: 2, username: 'user2', password: 'password2' }
];
// Login route
app.post('/login', (req, res) => {
const user = users.find(u => u.username === req.body.username && u.password === req.body.password);
if (!user) return res.status(401).send('Invalid credentials');
res.send('Login successful');
});
app.listen(port, () => {
console.log(`Authentication system running at http://localhost:${port}`);
});
Conclusion
Express.js is a powerful yet simple framework that makes building web applications and APIs with Node.js straightforward. With its minimalistic approach and robust features, you can quickly set up and expand your applications.