Flexbox in CSS: A Comprehensive Tutorial
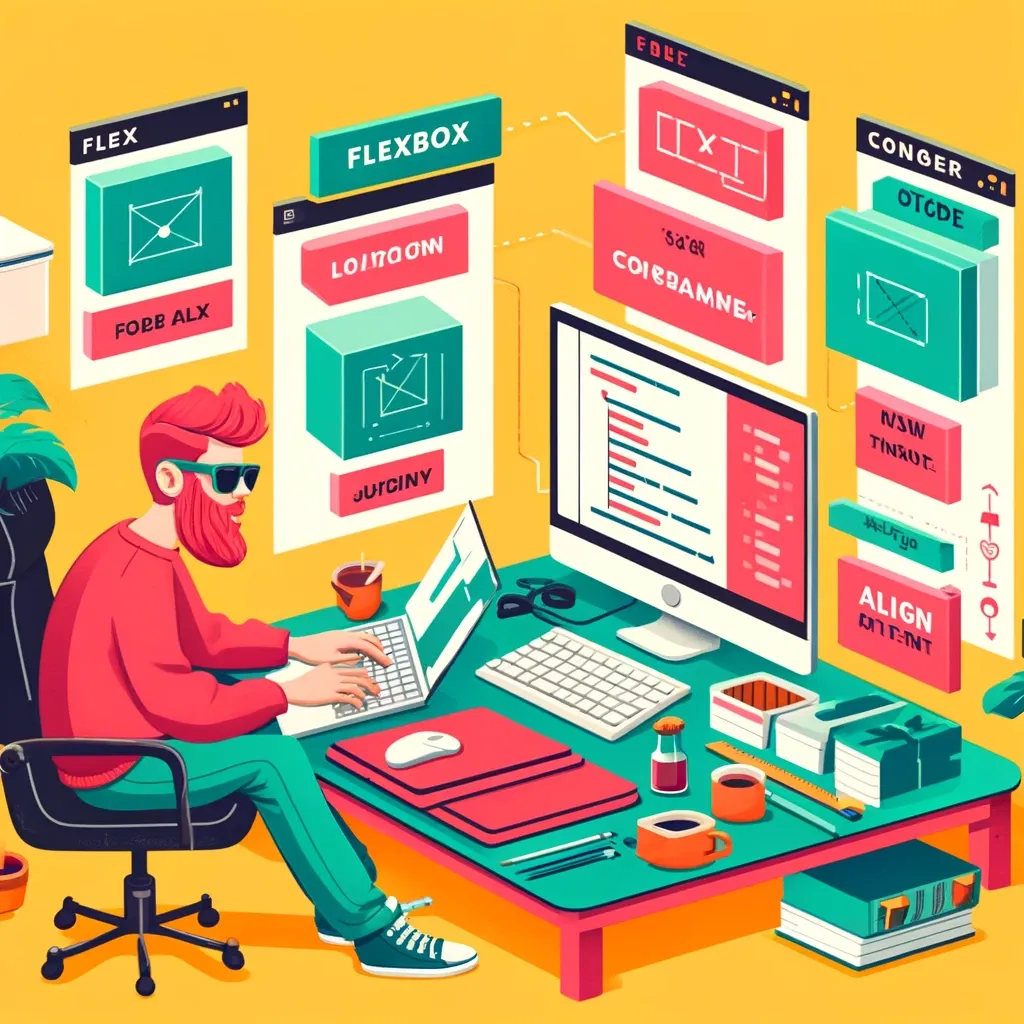
Flexbox (Flexible Box Layout) is a CSS layout model that allows developers to create flexible and responsive designs with ease. It is widely used for creating layouts where elements need to be dynamically adjusted to fit different screen sizes and orientations. This tutorial will delve into Flexbox, covering its key concepts, applications, and examples to help you understand how to leverage Flexbox for various scenarios.
What is Flexbox?
Flexbox is a one-dimensional layout model in CSS designed to distribute space along a single axis, either horizontally or vertically. It simplifies complex layouts by allowing you to align and distribute elements within a container with minimal code. Flexbox is particularly useful for creating responsive designs that adapt to different screen sizes and resolutions.
Flexbox is based on the concept of a "flex container" and "flex items." The flex container defines the layout context, and the flex items are the elements within the container that you want to align and distribute.
Flexbox Terminology and Key Concepts
To understand Flexbox, it's essential to grasp the following terminology and key concepts:
- Main Axis: The primary axis along which the flex items are arranged. It can be horizontal or vertical, depending on the layout direction.
- Cross Axis: The secondary axis perpendicular to the main axis. If the main axis is horizontal, the cross axis is vertical, and vice versa.
- Flex Container: The parent element that defines the Flexbox context.
- Flex Items: The child elements within the flex container.
- Justify Content: Controls the alignment of flex items along the main axis.
- Align Items: Controls the alignment of flex items along the cross axis.
- Flex Direction: Defines the direction of the main axis (row or column).
- Flex Wrap: Determines whether flex items wrap onto multiple lines if they exceed the container's space.
Flexbox Container Properties
Flexbox offers several properties to control the layout of the flex container:
- Display: To create a flex container, set
display: flex
ordisplay: inline-flex
.
.container {
display: flex;
}
- Flex Direction: Sets the direction of the main axis. It can be
row
,row-reverse
,column
, orcolumn-reverse
.
.container {
flex-direction: row; /* Default: horizontal layout */
}
- Justify Content: Controls the alignment along the main axis. Options include
flex-start
,flex-end
,center
,space-between
,space-around
, andspace-evenly
.
.container {
justify-content: center; /* Align items to the center */
}
- Align Items: Controls the alignment along the cross axis. Options include
flex-start
,flex-end
,center
,baseline
, andstretch
.
.container {
align-items: center; /* Align items to the center of the cross axis */
}
- Flex Wrap: Determines whether flex items wrap onto multiple lines. Set it to
nowrap
,wrap
, orwrap-reverse
.
.container {
flex-wrap: wrap; /* Allow flex items to wrap onto multiple lines */
}
Flexbox Item Properties
Flexbox also provides properties to control the behavior of flex items within the container:
- Flex Grow: Determines how much a flex item should grow relative to others when there's extra space in the container.
.item {
flex-grow: 1; /* Allow this item to grow */
}
- Flex Shrink: Determines how much a flex item should shrink relative to others when there's less space in the container.
.item {
flex-shrink: 1; /* Allow this item to shrink */
}
- Flex Basis: Sets the default size of a flex item before it is distributed along the main axis.
.item {
flex-basis: 100px; /* Set the default size to 100px */
}
- Align Self: Controls the alignment of an individual flex item along the cross axis. It can override
align-items
.
.item {
align-self: center; /* Center this item along the cross axis */
}
Aligning Items with Flexbox
Flexbox allows for various alignment techniques along the main and cross axes:
- Justify Content: Aligns items along the main axis. Here's an example demonstrating different options:
.container {
display: flex;
justify-content: space-between;
}
.item {
width: 100px;
height: 100px;
background-color: lightblue;
}
This code creates a row of three boxes, evenly spaced along the main axis.
- Align Items: Aligns items along the cross axis. Here's an example demonstrating different options:
.container {
display: flex;
align-items: flex-end;
}
.item {
width: 100px;
height: 100px;
background-color: lightcoral;
}
This code aligns the boxes along the bottom of the container (cross axis).
Building Layouts with Flexbox
Flexbox is versatile for building various layouts, from simple rows and columns to complex multi-line arrangements. Here's an example demonstrating a simple Flexbox layout:
.container {
display: flex;
flex-direction: row;
justify-content: space-around;
}
.item {
width: 100px;
height: 100px;
background-color: lightgreen;
}
This code creates a row with three evenly spaced boxes.
Flexbox also allows for nested layouts, where a flex item can contain its own flex layout. This technique is useful for creating complex designs. Here's an example of a nested layout:
/* Outer container */
.outer {
display: flex;
justify-content: space-between;
}
/* Inner container */
.inner {
display: flex;
flex-direction: column;
}
.item {
width: 100px;
height: 100px;
background-color: lightblue;
}
In this example, the outer container aligns items along the main axis, while the inner container aligns items vertically.
Examples of Flexbox in Practice
Flexbox has numerous applications in modern web design, from simple navigation bars to complex dashboards. Here are some examples of Flexbox in practice:
Navigation Bar
Flexbox can be used to create a responsive navigation bar. Here's an example:
.navbar {
display: flex;
justify-content: space-between;
padding: 10px;
}
.nav-item {
padding: 5px;
}
This code creates a navigation bar with items evenly spaced.
Photo Gallery
Flexbox can also create a responsive photo gallery. Here's an example:
.gallery {
display: flex;
flex-wrap: wrap;
justify-content: space-around;
}
.photo {
width: 200px;
height: 150px;
margin: 10px;
}
This code creates a photo gallery with evenly spaced photos that wrap onto multiple lines if needed.
Footer Layout
Flexbox is great for creating a footer with various elements. Here's an example:
.footer {
display: flex;
flex-direction: row;
justify-content: space-between;
}
.footer-item {
padding: 10px;
}
This code creates a footer with three evenly spaced sections.
Common Flexbox Patterns
Flexbox can be used to create common layout patterns used in web design:
Two-Column Layout
Flexbox is ideal for creating a simple two-column layout:
.container {
display: flex;
flex-direction: row;
justify-content: space-between;
}
.left {
width: 70%;
}
.right {
width: 30%;
}
This code creates a two-column layout with a 70-30 split.
Three-Column Layout
Flexbox can also create a three-column layout:
.container {
display: flex;
flex-direction: row;
justify-content: space-between;
}
.left {
width: 25%;
}
.center {
width: 50%;
}
.right {
width: 25%;
}
This code creates a three-column layout with a 25-50-25 split.
Helpful Videos
Conclusion
Flexbox is a powerful and flexible layout model in CSS, allowing developers to create responsive and dynamic layouts with ease. By understanding the key concepts and properties discussed in this tutorial, you'll be able to leverage Flexbox for a wide range of applications.
Experiment with different Flexbox techniques and explore various layout patterns to enhance your web designs. Flexbox provides the tools to create efficient, adaptable, and visually appealing layouts, making it an invaluable resource for modern web development.