Exploring Ruby Hashes: A Basic Guide
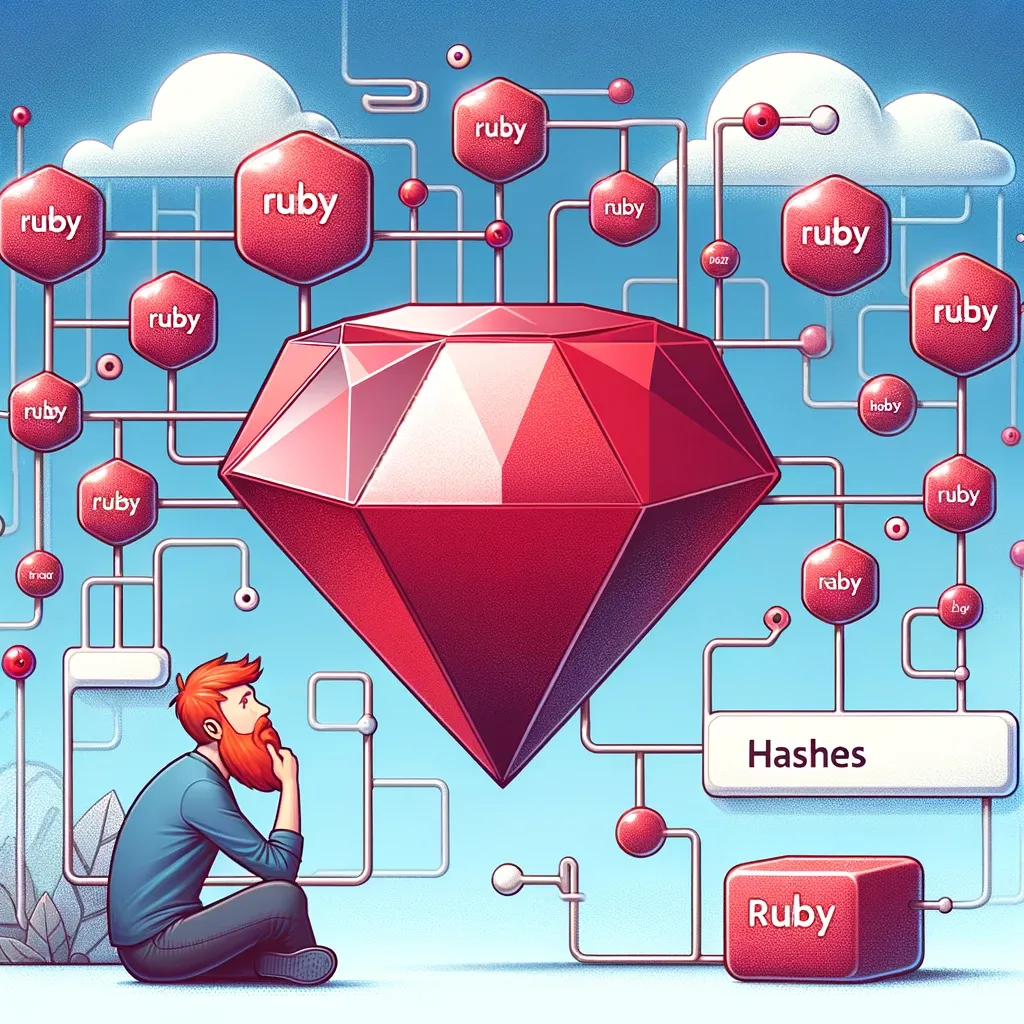
Ruby hashes are a fundamental data structure in the Ruby programming language, allowing you to store key-value pairs. They are similar to dictionaries in other languages and are extremely versatile, used in various programming contexts. This guide explores the basics of Ruby hashes, including their creation, manipulation, and common use cases.
What is a Ruby Hash?
A Ruby hash is a collection of unique keys and their associated values. You can think of a hash as a set of unique keys where each key points to a specific value. The keys can be of any type, but symbols and strings are the most common.
# Example of a simple hash with string keys
person = {
"name" => "John",
"age" => 30,
"profession" => "Developer"
}
# Example of a hash with symbol keys
person_symbol = {
name: "John",
age: 30,
profession: "Developer"
}
# Accessing a hash value by key
puts person["name"] # Output: John
puts person_symbol[:age] # Output: 30
Creating Ruby Hashes
There are multiple ways to create hashes in Ruby. The most straightforward method is to use curly braces {}
. You can also use the Hash.new
constructor to create an empty hash or set a default value for missing keys.
# Create an empty hash
empty_hash = {}
# Create a hash with default value for missing keys
default_hash = Hash.new("default")
# Attempt to access a missing key
puts default_hash[:missing_key] # Output: default
Manipulating Ruby Hashes
Hashes offer a variety of methods for manipulation. You can add, remove, or update key-value pairs, as well as iterate over the hash.
# Adding key-value pairs
person[:country] = "USA"
# Updating a key's value
person[:age] = 31
# Removing a key-value pair
person.delete(:profession)
# Iterating over a hash
person.each do |key, value|
puts "\#{key}: \#{value}"
end
# Output:
# name: John
# age: 31
# country: USA
Common Use Cases for Ruby Hashes
Ruby hashes are used in many contexts, from basic data storage to complex data manipulation. Some common use cases include:
- Configuration Settings: Hashes are often used to store configuration options for programs and libraries.
- Data Mapping: Hashes can map data from one form to another, making them useful for tasks like data transformation.
- Counting Occurrences: You can use a hash to count the occurrences of elements in an array or string.
# Counting character occurrences in a string
str = "hello world"
char_count = {}
str.each_char do |char|
char_count[char] = char_count.fetch(char, 0) + 1
end
puts char_count
# Output:
# {"h"=>1, "e"=>1, "l"=>3, "o"=>2, " "=>1, "w"=>1, "r"=>1, "d"=>1}
Helpful Videos
Conclusion
Ruby hashes are a versatile and powerful data structure, suitable for a wide range of tasks. Understanding how to create, manipulate, and use hashes is crucial for Ruby programming. With this guide, you should have a solid foundation for working with hashes in your Ruby projects.