Essential SQL Commands for PostgreSQL: A Comprehensive Guide
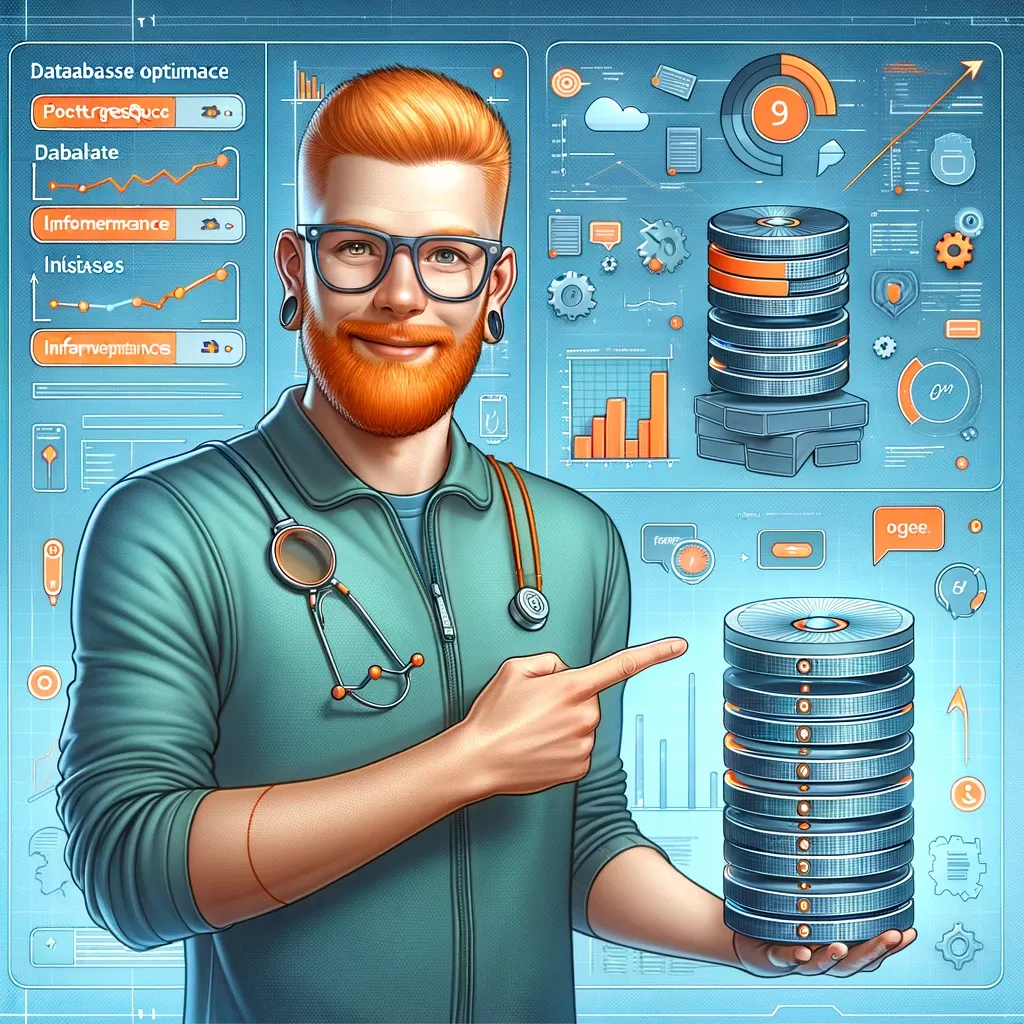
PostgreSQL is a powerful, open-source relational database system known for its robustness and performance. Whether you're a beginner or an experienced developer, understanding and mastering SQL commands is crucial for effectively managing and manipulating your database. In this guide, we will cover some of the most essential SQL commands used in PostgreSQL.
Setting Up PostgreSQL
Before diving into SQL commands, ensure you have PostgreSQL installed on your machine. You can download it from the official PostgreSQL website. Once installed, you can interact with PostgreSQL using the psql
command-line tool.
Basic SQL Commands
1. Creating a Database
To create a new database in PostgreSQL, use the following command:
CREATE DATABASE mydatabase;
2. Connecting to a Database
To connect to a specific database, use the \c
command followed by the database name:
\c mydatabase
3. Creating a Table
To create a new table, use the CREATE TABLE
command. Here’s an example:
CREATE TABLE employees (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
position VARCHAR(50),
salary NUMERIC
);
4. Inserting Data
To insert data into a table, use the INSERT INTO
command:
INSERT INTO employees (name, position, salary) VALUES ('John Doe', 'Manager', 60000);
5. Querying Data
To retrieve data from a table, use the SELECT
command:
SELECT * FROM employees;
6. Updating Data
To update existing data in a table, use the UPDATE
command:
UPDATE employees SET salary = 65000 WHERE name = 'John Doe';
7. Deleting Data
To delete data from a table, use the DELETE
command:
DELETE FROM employees WHERE name = 'John Doe';
Advanced SQL Commands
SQL Joins
SQL joins are used to combine rows from two or more tables based on a related column. Here are the most common types of joins:
Joining Tables
To combine rows from two or more tables based on a related column, use the JOIN
command:
SELECT employees.name, departments.department_name
FROM employees
JOIN departments ON employees.department_id = departments.id;
1. INNER JOIN
An INNER JOIN
returns only the rows that have matching values in both tables.
Syntax:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.column = table2.column;
Example:
SELECT employees.name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.id;
2. LEFT JOIN (or LEFT OUTER JOIN)
A LEFT JOIN
returns all the rows from the left table and the matched rows from the right table. If no match is found, NULL values are returned for columns from the right table.
Syntax:
SELECT columns
FROM table1
LEFT JOIN table2
ON table1.column = table2.column;
Example:
SELECT employees.name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.id;
3. RIGHT JOIN (or RIGHT OUTER JOIN)
A RIGHT JOIN
returns all the rows from the right table and the matched rows from the left table. If no match is found, NULL values are returned for columns from the left table.
Syntax:
SELECT columns
FROM table1
RIGHT JOIN table2
ON table1.column = table2.column;
Example:
SELECT employees.name, departments.department_name
FROM employees
RIGHT JOIN departments ON employees.department_id = departments.id;
4. FULL JOIN (or FULL OUTER JOIN)
A FULL JOIN
returns all rows when there is a match in either left or right table. If there is no match, NULL values are returned for columns from the table without matches.
Syntax:
SELECT columns
FROM table1
FULL JOIN table2
ON table1.column = table2.column;
Example:
SELECT employees.name, departments.department_name
FROM employees
FULL JOIN departments ON employees.department_id = departments.id;
5. CROSS JOIN
A CROSS JOIN
returns the Cartesian product of the two tables, meaning it returns all possible combinations of rows from both tables.
Syntax:
SELECT columns
FROM table1
CROSS JOIN table2;
Example:
SELECT employees.name, departments.department_name
FROM employees
CROSS JOIN departments;
6. SELF JOIN
A SELF JOIN
is a regular join but the table is joined with itself. It is useful for hierarchical data or comparing rows within the same table.
Syntax:
SELECT a.column1, b.column2
FROM table a, table b
WHERE condition;
Example:
SELECT e1.name AS Employee, e2.name AS Manager
FROM employees e1
INNER JOIN employees e2 ON e1.manager_id = e2.id;
Detailed Examples
INNER JOIN Example
Suppose we have two tables, orders
and customers
. We want to find all orders along with customer details.
SELECT orders.order_id, customers.customer_name
FROM orders
INNER JOIN customers ON orders.customer_id = customers.id;
LEFT JOIN Example
We want to find all customers and their orders, including customers who have not placed any orders.
SELECT customers.customer_name, orders.order_id
FROM customers
LEFT JOIN orders ON customers.id = orders.customer_id;
RIGHT JOIN Example
We want to find all orders and the customers who placed them, including orders that are not associated with any customers.
SELECT customers.customer_name, orders.order_id
FROM customers
RIGHT JOIN orders ON customers.id = orders.customer_id;
FULL JOIN Example
We want to find all customers and all orders, including those that do not have matches in the other table.
SELECT customers.customer_name, orders.order_id
FROM customers
FULL JOIN orders ON customers.id = orders.customer_id;
CROSS JOIN Example
We want to create a list of all possible combinations of products and customers.
SELECT products.product_name, customers.customer_name
FROM products
CROSS JOIN customers;
SELF JOIN Example
We want to find pairs of employees where one is the manager of the other.
SELECT e1.name AS Employee, e2.name AS Manager
FROM employees e1
INNER JOIN employees e2 ON e1.manager_id = e2.id;
Understanding these different types of joins and their use cases will help you to effectively query and manipulate your PostgreSQL databases, ensuring you can retrieve and analyze your data as needed.
Aggregating Data
Data aggregation is a crucial aspect of data analysis, allowing you to summarize and perform calculations on your data to extract meaningful insights. PostgreSQL provides powerful aggregate functions that make it easy to perform these operations. In this guide, we will delve into the details of data aggregation using SQL.
What is Data Aggregation?
Data aggregation involves performing calculations on multiple rows of data to produce a single result. This can include operations such as counting the number of rows, calculating averages, finding sums, and determining minimum and maximum values.
Common Aggregate Functions
COUNT
The COUNT
function returns the number of rows that match a specified condition.
Example:
SELECT COUNT(*) FROM employees;
This query counts the total number of employees.
SUM
The SUM
function returns the total sum of a numeric column.
Example:
SELECT SUM(salary) FROM employees;
This query calculates the total salary of all employees.
AVG
The AVG
function returns the average value of a numeric column.
Example:
SELECT AVG(salary) FROM employees;
This query calculates the average salary of all employees.
MIN and MAX
The MIN
and MAX
functions return the minimum and maximum values of a column, respectively.
Example:
SELECT MIN(salary) FROM employees;
SELECT MAX(salary) FROM employees;
These queries find the lowest and highest salaries among employees.
Grouping Data with GROUP BY
The GROUP BY
clause groups rows that have the same values in specified columns into summary rows. It is often used with aggregate functions to perform calculations on each group of data.
Syntax:
SELECT column1, aggregate_function(column2)
FROM table
GROUP BY column1;
Example: Calculating Average Salary by Position
To calculate the average salary for each position in the employees
table, you can use the GROUP BY
clause with the AVG
function:
SELECT position, AVG(salary) AS average_salary
FROM employees
GROUP BY position;
Explanation:
SELECT position, AVG(salary) AS average_salary
: Selects the position and calculates the average salary for each position.FROM employees
: Specifies theemployees
table.GROUP BY position
: Groups the results by position, so the average salary is calculated for each group of positions.
Example: Counting Employees by Department
To count the number of employees in each department, you can use the COUNT
function with the GROUP BY
clause:
SELECT department, COUNT(*) AS num_employees
FROM employees
GROUP BY department;
Explanation:
SELECT department, COUNT(*) AS num_employees
: Selects the department and counts the number of employees in each department.FROM employees
: Specifies theemployees
table.GROUP BY department
: Groups the results by department, so the count is calculated for each group of departments.
Filtering Grouped Data with HAVING
The HAVING
clause is used to filter groups based on a condition, similar to the WHERE
clause but for groups.
Example: Finding Departments with More Than 10 Employees
To find departments with more than 10 employees, you can use the HAVING
clause:
SELECT department, COUNT(*) AS num_employees
FROM employees
GROUP BY department
HAVING COUNT(*) > 10;
Explanation:
SELECT department, COUNT(*) AS num_employees
: Selects the department and counts the number of employees in each department.FROM employees
: Specifies theemployees
table.GROUP BY department
: Groups the results by department.HAVING COUNT(*) > 10
: Filters the groups to include only departments with more than 10 employees.
Data aggregation is a powerful tool for summarizing and analyzing your data in PostgreSQL. By mastering aggregate functions and the GROUP BY
and HAVING
clauses, you can perform complex calculations and extract valuable insights from your data. Whether you are calculating averages, counting rows, or summarizing data in other ways, these techniques are essential for effective data analysis.
To perform calculations on data, such as counting rows or calculating averages, use aggregate functions:
SELECT position, AVG(salary) as average_salary
FROM employees
GROUP BY position;
Creating Indexes
Indexes are a crucial aspect of database optimization, enabling faster retrieval of records and enhancing overall query performance. In PostgreSQL, creating indexes on columns that are frequently searched can significantly improve the efficiency of your database operations. This guide will provide a detailed overview of creating and using indexes in PostgreSQL.
What is an Index?
An index is a database object that provides a quick lookup of data in a column or set of columns. Think of it as a book's index, which helps you quickly find the page containing the information you are looking for.
Benefits of Using Indexes
- Improved Query Performance: Indexes speed up the retrieval of rows by reducing the amount of data that needs to be scanned.
- Efficient Sorting and Filtering: Indexes enhance the performance of sorting and filtering operations.
- Enforcement of Uniqueness: Unique indexes ensure that no duplicate values are inserted into the indexed columns.
Creating an Index
Syntax
The basic syntax for creating an index in PostgreSQL is:
CREATE INDEX index_name ON table_name (column_name);
Example
To create an index on the name
column in the employees
table, use the following command:
CREATE INDEX idx_employees_name ON employees (name);
Explanation:
CREATE INDEX idx_employees_name
: Creates an index namedidx_employees_name
.ON employees (name)
: Specifies theemployees
table and thename
column to be indexed.
Types of Indexes
1. B-tree Index
B-tree indexes are the default and most commonly used type of index in PostgreSQL. They are suitable for a wide range of queries.
Example:
CREATE INDEX idx_employees_name ON employees USING btree (name);
2. Hash Index
Hash indexes are used for equality comparisons. They are faster for these operations but are not as versatile as B-tree indexes.
Example:
CREATE INDEX idx_employees_id ON employees USING hash (id);
3. GiST Index
GiST (Generalized Search Tree) indexes are used for complex data types like geometric data and full-text search.
Example:
CREATE INDEX idx_employees_geo ON employees USING gist (location);
4. GIN Index
GIN (Generalized Inverted Index) indexes are used for full-text search and arrays.
Example:
CREATE INDEX idx_employees_tags ON employees USING gin (tags);
Managing Indexes
Viewing Indexes
To view the indexes on a table, you can use the \d
command in psql
:
\d employees
Dropping an Index
To remove an index, use the DROP INDEX
command:
DROP INDEX idx_employees_name;
Explanation:
DROP INDEX idx_employees_name
: Deletes the index namedidx_employees_name
.
Performance Considerations
While indexes can significantly improve query performance, they also introduce some overhead:
- Disk Space: Indexes require additional storage space.
- Maintenance Costs: Indexes need to be updated whenever the indexed columns are modified, which can affect write performance.
- Choosing the Right Index: Not all queries benefit from indexes, and creating too many indexes can degrade performance.
Example: Using Indexes for Performance Optimization
Scenario
Consider a table orders
with a large number of records. You frequently query this table to find orders by customer_id
.
Without Index
SELECT * FROM orders WHERE customer_id = 123;
This query requires a full table scan, which is inefficient for large tables.
With Index
CREATE INDEX idx_orders_customer_id ON orders (customer_id);
SELECT * FROM orders WHERE customer_id = 123;
By creating an index on customer_id
, the query can quickly locate the matching rows, significantly improving performance.
Indexes are a powerful tool for optimizing database performance in PostgreSQL. By carefully choosing which columns to index and understanding the different types of indexes available, you can enhance the efficiency of your database queries and ensure that your applications run smoothly. Remember to balance the benefits of indexes with their maintenance costs and storage requirements.
Conclusion
Mastering these SQL commands will significantly enhance your ability to manage and manipulate data in PostgreSQL. Whether you are creating databases, inserting data, or performing complex queries, these commands form the foundation of effective database management. Happy coding!