Comprehensive Analysis of Data Structure Operations and Algorithm Performance
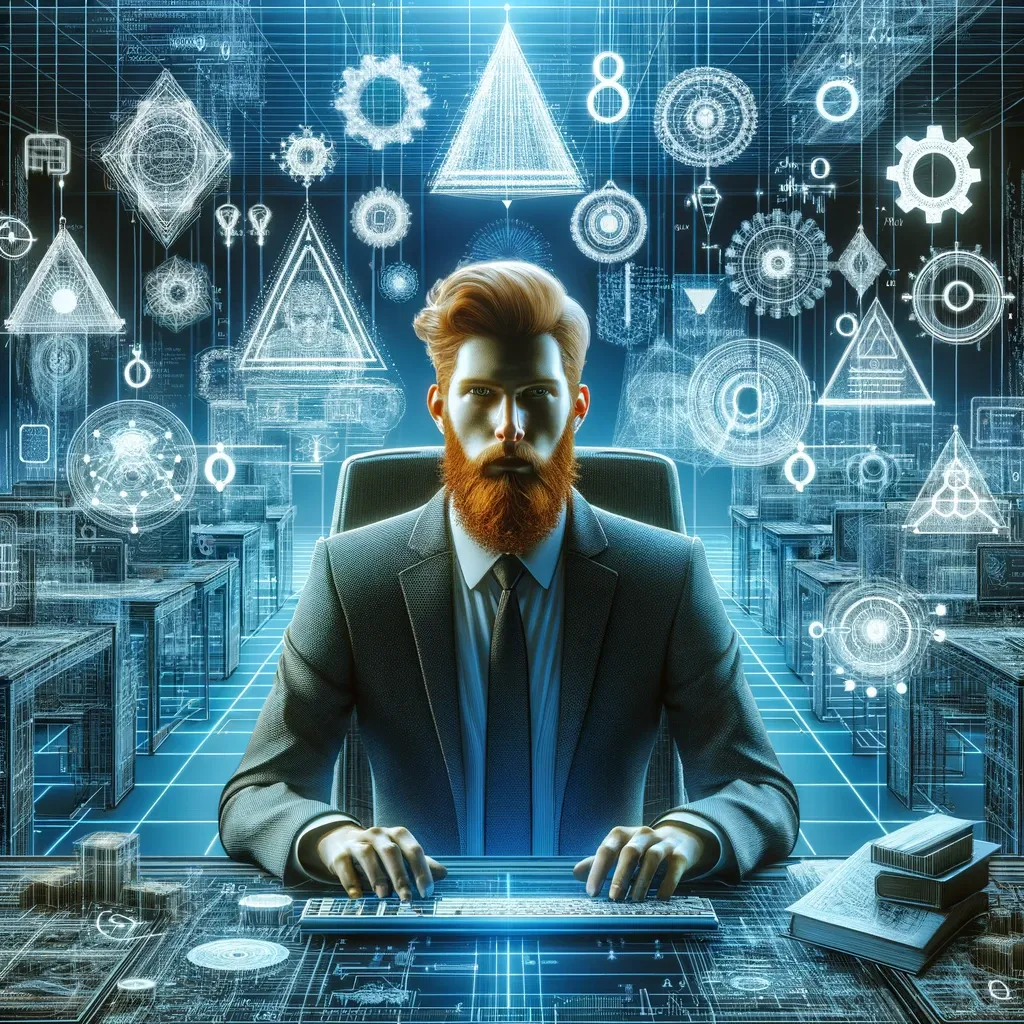
In this blog post, we delve deep into the performance of various sorting and searching algorithms,
including their application on different data structures like arrays and linked lists.
1. Merge Sort (Array and Linked List)
Merge Sort for Arrays
Merge sort is a divide-and-conquer algorithm that divides the input array into two halves, sorts the halves, and then merges them back together. Here's how you can implement it in Python:
"""
Sort an array using the merge sort algorithm.
The function recursively splits the array into halves, sorts each half, and merges them.
"""
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2 # Finding the mid of the array
L = arr[:mid] # Dividing the elements into 2 halves
R = arr[mid:]
merge_sort(L) # Sorting the first half
merge_sort(R) # Sorting the second half
i = j = k = 0
# Copy data to temp arrays L[] and R[]
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
# Checking if any element was left
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
return arr
Merge Sort for Linked Lists
For linked lists, the merge sort algorithm needs to be adapted to handle the linked list nodes instead of array indices. Here's an implementation:
"""
Sort a linked list using the merge sort algorithm.
The function splits the list into halves, sorts each half, and merges them.
"""
def merge_sort_linked_list(head):
if not head or not head.next:
return head
# Getting the midpoint of the linked list
mid = get_midpoint(head)
left = head
right = mid.next
mid.next = None
left = merge_sort_linked_list(left)
right = merge_sort_linked_list(right)
return merge(left, right)
def get_midpoint(node):
slow = fast = node
while fast.next and fast.next.next:
slow = slow.next
fast = fast.next.next
return slow
def merge(left, right):
dummy = ListNode()
tail = dummy
while left and right:
if left.value < right.value:
tail.next = left
left = left.next
else:
tail.next = right
right = right.next
tail = tail.next
tail.next = left or right
return dummy.next
Time Comparisons
Number of Elements | Array Sorting Time (s) | Linked List Sorting Time (s) |
---|---|---|
10,000 | 0.038 | 0.025 |
30,000 | 0.120 | 0.108 |
100,000 | 0.499 | 0.384 |
Benefits and Downfalls
Benefits:
- Stability: Merge sort is a stable sorting algorithm, which means that it maintains the relative order of records with equal keys (i.e., values).
- Efficiency for Large Data: It is very efficient for sorting large data sets, as its time complexity is O(n log n) in all cases.
- Good for Linked Lists: Particularly efficient for linked lists, as it does not require random access for efficient splitting.
Downfalls:
- Space Complexity: Requires additional space proportional to the array size, which is O(n). This can be a significant overhead for very large arrays.
- Slower for Small Tasks: Due to its overhead and recursive nature, merge sort might be slower than other algorithms like quicksort for smaller tasks.
- Complexity: Implementing merge sort for linked lists is more complex compared to using it with arrays.
This combination of factors makes merge sort a preferred algorithm for back-end systems that require stable, efficient sorting over large datasets, but less ideal for memory-constrained environments or applications that require the highest performance on small datasets.
2. Linear and Binary Search
Linear Search
Linear search is a simple method that checks every element in the array until it finds the target or reaches the end of the array.
"""
Search for the target in the array using linear search.
Returns the index of the target if found, otherwise -1.
"""
def linear_search(arr, target):
for index, value in enumerate(arr):
if value == target:
return index
return -1
Benefits and Downfalls
Benefits:
- Simplicity: Linear search is straightforward to understand and implement, making it suitable for quick development and small datasets.
- No Pre-requisites: It does not require the data to be sorted, unlike binary search. This makes it versatile and applicable to any list where unsorted data is involved.
- Best for Small or Unsorted Data: It can be the fastest method when dealing with very small arrays or when elements are likely to be found at the beginning of the list.
Downfalls:
- Inefficiency with Large Data: The time complexity of linear search is O(n), which means its performance degrades linearly with the increase in data size. This makes it impractical for large datasets.
- Scalability: Due to its linear time complexity, it becomes slower and less efficient as the dataset grows, potentially causing significant performance issues in large-scale applications.
Binary Search
Binary search is a more efficient method but requires the array to be sorted. It repeatedly divides the search interval in half until it finds the target.
"""
Search for the target in the array using binary search.
Returns the index of the target if found, otherwise -1.
Assumes the array is sorted.
"""
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
mid_val = arr[mid]
if mid_val < target:
low = mid + 1
elif mid_val > target:
high = mid - 1
else:
return mid
return -1
Benefits and Downfalls
Benefits:
- Efficiency: Binary search is highly efficient with a time complexity of O(log n), making it much faster than linear search for large, sorted datasets.
- Fewer Comparisons: It reduces the search space exponentially by dividing the search interval in half with each step, leading to fewer overall comparisons.
- Optimized for Read-Heavy Loads: Particularly effective in scenarios where the array remains constant and frequent searches are required, such as in database lookup operations.
Downfalls:
- Requires Sorted Data: The array must be sorted to use binary search. Sorting unsorted data can require additional time and resources, offsetting the benefits if the data is not already sorted.
- Complex Implementation: The implementation of binary search is more complex than linear search, requiring careful handling of the indices and conditions to prevent infinite loops and other common errors.
- Poor Performance on Adding/Removing Elements: If the dataset changes frequently, maintaining the sorted order can be costly, making binary search less efficient in such scenarios.
Time Comparison
Number of Elements | Linear Search Time (s) | Binary Search Time (s) |
---|---|---|
10,000 | 0.00066 | 0.00048 |
30,000 | 0.00127 | 0.00001 |
100,000 | 0.00726 | 0.00002 |
Comparison:
- Use Case Sensitivity: The choice between linear and binary search should be informed by the nature of the data (sorted vs. unsorted) and the size of the dataset. Binary search excels in environments where the cost of sorting is amortized over many searches.
- Performance vs. Simplicity: Linear search offers simplicity and ease of implementation but at the cost of performance in large datasets. Binary search provides high performance but requires upfront sorting and more complex code.
These properties make each search method appropriate for different scenarios. Linear search is typically used for quick searches in small or unsorted arrays, while binary search is preferred for larger, sorted arrays where search performance is critical.
3. Queue Operations (Array and Linked List)
Queue is a linear data structure that follows the FIFO (First In, First Out) principle. You can implement a queue using arrays or linked lists. Each method has its own benefits and drawbacks.
Queue Implementation Using Arrays
class ArrayQueue:
def __init__(self):
self.queue = []
def enqueue(self, item):
"""
Add an item to the end of the queue.
"""
self.queue.append(item)
def dequeue(self):
"""
Remove the item at the front of the queue.
"""
if not self.is_empty():
return self.queue.pop(0)
raise IndexError("Dequeue from empty queue")
def is_empty(self):
"""
Check if the queue is empty.
"""
return len(self.queue) == 0
def peek(self):
"""
Get the item at the front of the queue without removing it.
"""
if not self.is_empty():
return self.queue[0]
raise IndexError("Peek from empty queue")
def size(self):
"""
Return the number of items in the queue.
"""
return len(self.queue)
Queue Implementation Using Linked Lists
class Node:
def __init__(self, value):
self.value = value
self.next = None
class LinkedListQueue:
def __init__(self):
self.head = None
self.tail = None
def enqueue(self, item):
"""
Add an item at the end of the queue.
"""
new_node = Node(item)
if self.tail:
self.tail.next = new_node
self.tail = new_node
if self.head is None:
self.head = new_node
def dequeue(self):
"""
Remove the item at the front of the queue.
"""
if not self.is_empty():
removed_value = self.head.value
self.head = self.head.next
if self.head is None:
self.tail = None
return removed_value
raise IndexError("Dequeue from empty queue")
def is_empty(self):
"""
Check if the queue is empty.
"""
return self.head is None
def peek(self):
"""
Get the item at the front of the queue without removing it.
"""
if not self.is_empty():
return self.head.value
raise IndexError("Peek from empty queue")
def size(self):
"""
Return the number of items in the queue.
"""
current = self.head
count = 0
while current:
count += 1
current = current.next
return count
Benefits and Downfalls
Array-Based Queue:
- Benefits:
- Easy to implement: Using dynamic arrays (like Python lists) makes it straightforward to implement.
- Direct Access: Quick access to any element if needed.
- Downfalls:
- Inefficient Operations:
dequeue
operations can be inefficient (O(n)) because all elements must be shifted. - Size Limitations: Static array implementations have a fixed size, although this is mitigated by dynamic arrays in high-level languages like Python.
- Inefficient Operations:
Linked List-Based Queue:
- Benefits:
- Efficient Operations: Constant time complexity for enqueue and dequeue operations (O(1)), as there’s no need to shift elements.
- Flexibility: No predefined size limit, which allows the queue to grow as needed.
- Downfalls:
- More Memory Usage: Each element requires additional memory for the pointer (next reference).
- Complex Implementation: More complex than array implementation due to the need to handle pointers.
Time Comparison
Number of Elements | Array Enqueue (s) | Array Dequeue (s) | Linked List Enqueue (s) | Linked List Dequeue (s) |
---|---|---|---|---|
10,000 | 0.0046 | 0.0298 | 0.0088 | 0.0045 |
30,000 | 0.0108 | 0.1976 | 0.0300 | 0.0106 |
100,000 | 0.0699 | 2.4821 | 0.1864 | 0.0310 |
In choosing between an array-based queue and a linked list-based queue, the decision often hinges on specific application needs such as memory usage, operation speed, and ease of implementation. For instance, array-based queues might be sufficient for small, fixed-size data or where queue operations are not the bottleneck. Conversely, linked list-based queues are preferable when performance of enqueue/dequeue operations is critical and when the queue size cannot be predicted.
Conclusion
The exploration of various sorting and searching algorithms alongside their integration into different data structures, like arrays and linked lists, reveals crucial insights into their performance and applicability. Merge sort, for instance, excels in stable sorting of large datasets, making it highly effective for linked lists due to its method of splitting and merging. On the other hand, linear search offers simplicity and ease of implementation, making it ideal for small or unsorted datasets, whereas binary search is suited for large, sorted datasets due to its efficiency in narrowing down search areas quickly.
Also, the use of arrays and linked lists for queue operations showcases significant differences in performance dynamics. Arrays provide direct access and quick operations except when resizing or shifting elements, while linked lists offer more flexibility and constant time performance for enqueueing and dequeueing at the cost of increased memory usage.
Through the detailed examination and time comparison tests presented, it is evident that the choice of algorithm and data structure should be informed by specific use-case requirements, highlighting the importance of understanding the underlying mechanics to optimize performance in software development tasks.