Creating a CRUD API with Ruby on Rails and Making API Calls
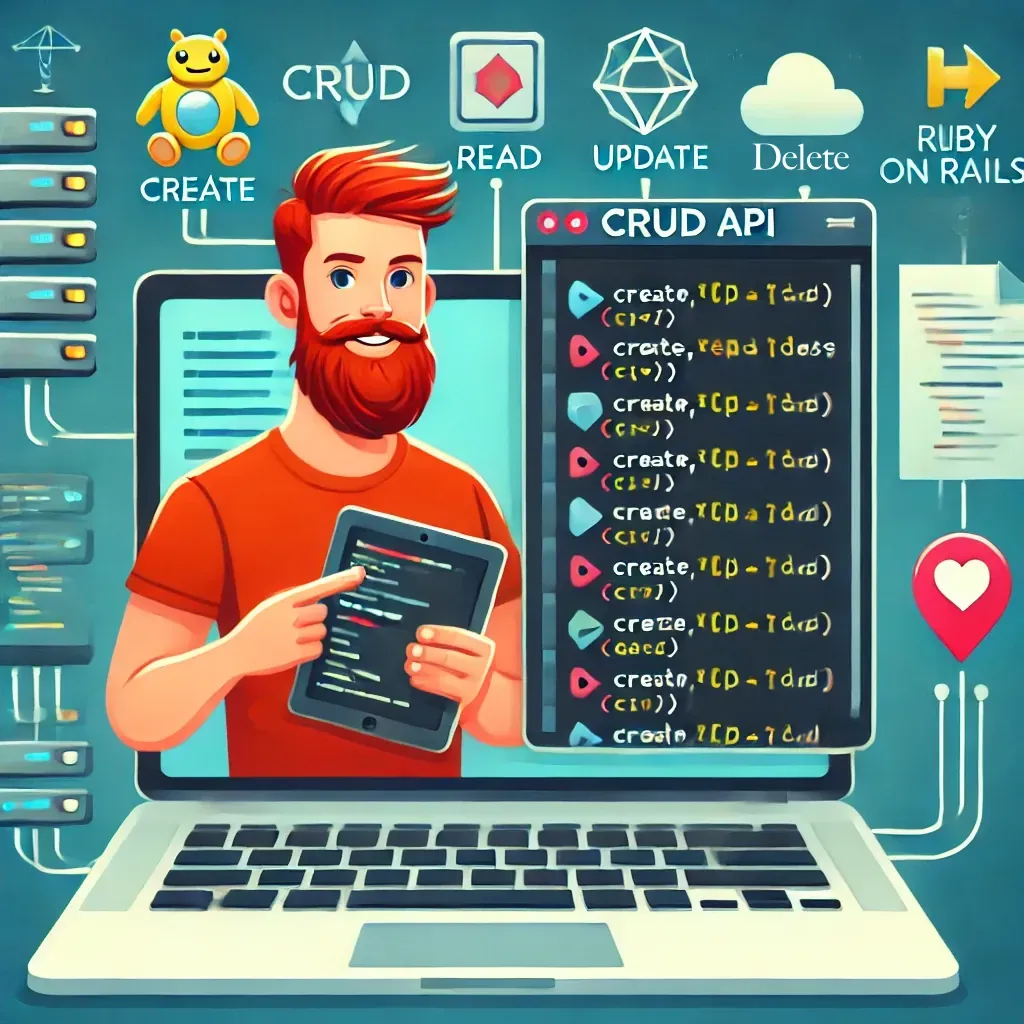
In this tutorial, we will create a simple API using Ruby on Rails that supports CRUD (Create, Read, Update, Delete) operations. We will also demonstrate how to make API calls to this Rails API and handle the data.
Prerequisites
Before we start, ensure you have the following installed:
- Ruby
- Rails
- Postman (for testing API calls)
Step 1: Setting Up the Rails Project
First, create a new Rails project:
rails new blog_api
cd blog_api
Generate a scaffold for a simple resource, Post
:
rails generate scaffold Post title:string content:text
Setting up database
- Ensure your database and credentials are configured in config/database.yml
- Create the database
rails db:create
- Run the migrations to create the database tables:
rails db:migrate
Step 2: Configuring Routes
In config/routes.rb
, ensure the routes are set up for the posts resource:
Rails.application.routes.draw do
resources :posts
end
Step 3: Modify the PostsController
Update your PostsController
to skip CSRF verification for JSON format requests.
app/controllers/posts_controller.rb
class PostsController < ApplicationController
# Add to controller
protect_from_forgery unless: -> { request.format.json? }
end
Step 4: Testing the API
Start the Rails server:
rails s
Creating a Post
To create a post, make a POST request to http://localhost:3000/posts
with the following JSON body:
{
"post": {
"title": "My First Post",
"content": "This is the content of my first post."
}
}
Reading Posts
To get a list of posts, make a GET request to http://localhost:3000/posts
.
Updating a Post
To update a post, make a PUT request to http://localhost:3000/posts/1
with the following JSON body:
{
"post": {
"title": "Updated Post",
"content": "This is the updated content."
}
}
Deleting a Post
To delete a post, make a DELETE request to http://localhost:3000/posts/1
.
Step 5: Making API Calls with Postman
Sending a GET Request
- Open Postman and create a new GET request.
- Enter the URL:
http://localhost:3000/posts
- Click
Send
and you should see a list of posts.
Sending a POST Request
- Create a new POST request in Postman.
- Enter the URL:
http://localhost:3000/posts
- Go to the
Body
tab and selectraw
andJSON
. - Click
Send
to create a new post.
Enter the JSON body:
{
"post": {
"title": "Another Post",
"content": "This is another post."
}
}
Sending a PUT Request
- Create a new PUT request in Postman.
- Enter the URL:
http://localhost:3000/posts/1
- Go to the
Body
tab and selectraw
andJSON
. - Click
Send
to update the post.
Enter the JSON body:
{
"post": {
"title": "Updated Post Title",
"content": "This is the updated content of the post."
}
}
Sending a DELETE Request
- Create a new DELETE request in Postman.
- Enter the URL:
http://localhost:3000/posts/1
- Click
Send
to delete the post.
Conclusion
In this tutorial, we created a simple CRUD API using Ruby on Rails and demonstrated how to make API calls using Postman. This setup provides a foundation for building more complex APIs and interacting with them programmatically.