Build Your First Game in Ruby: A Number Guessing Game Tutorial
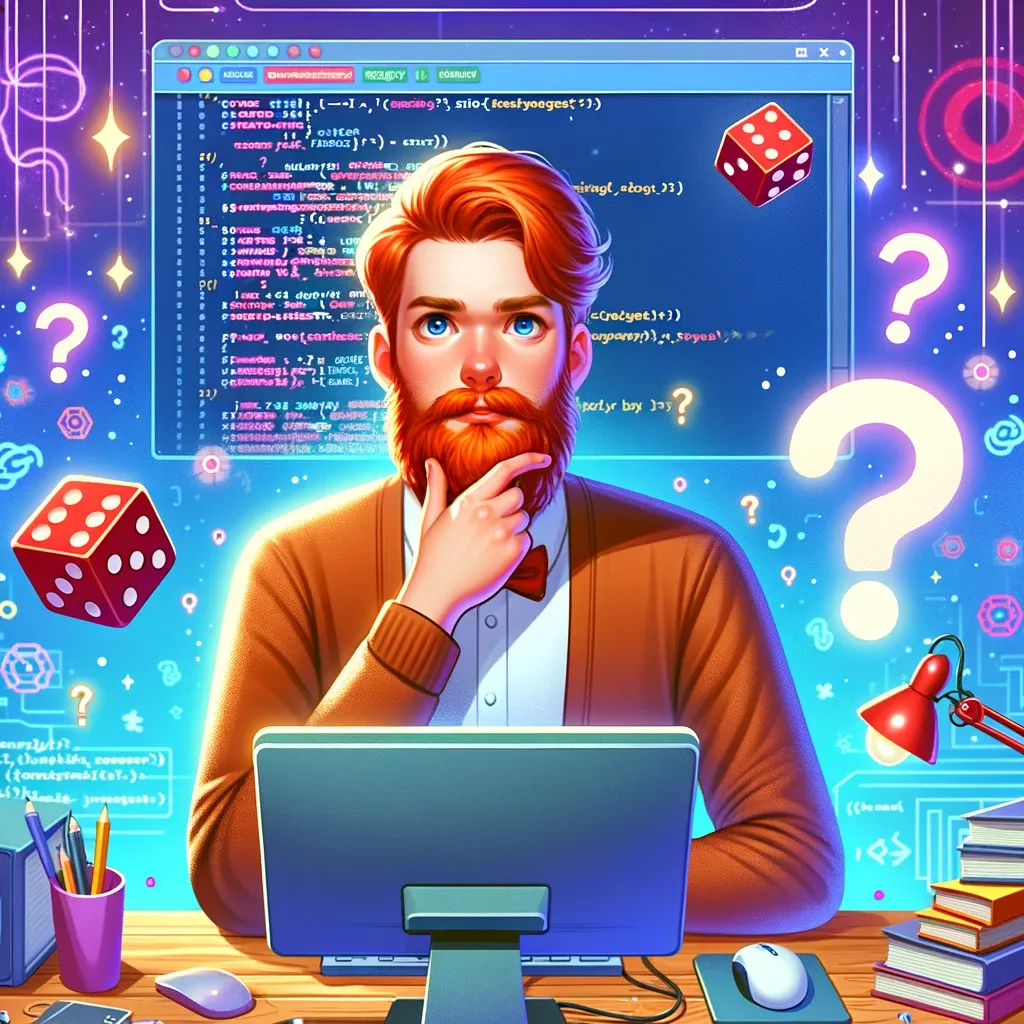
Introduction
In this tutorial, we'll dive into the fun world of coding by creating a simple text-based game using Ruby. If you're new to programming or looking to get started with Ruby, this is a perfect project for you. You'll learn the basics of user input, loops, conditionals, and random number generation—all through building a number guessing game!
Setting Up Your Ruby Environment
Before we start coding, ensure that you have Ruby installed on your computer. You can download and install Ruby from the official Ruby website.
Step 1: Planning the Game
Our game will generate a random number between 1 and 100, and the player will have a limited number of attempts to guess it correctly. After each guess, the game will inform the player whether the guess was too high or too low.
Step 2: Writing the Code
Here's the complete code for our number guessing game:
# number_guessing_game.rb
puts "Welcome to the Number Guessing Game!"
secret_number = rand(1..100)
max_guesses = 5
max_guesses.times do |guess|
print "Guess #{guess + 1}/#{max_guesses}: "
player_guess = gets.to_i
if player_guess == secret_number
puts "Congratulations! You guessed the correct number: #{secret_number}"
break
elsif player_guess > secret_number
puts "Too high!"
else
puts "Too low!"
end
if guess == max_guesses - 1
puts "Game over! The secret number was #{secret_number}. Better luck next time!"
end
end
Step 3: Running the Game
To run the game, save the code in a file named number_guessing_game.rb
. Open your terminal, navigate to the directory where the file is saved, and type:
ruby number_guessing_game.rb
You'll see the game prompts in the terminal, and you can start playing by entering your guesses.
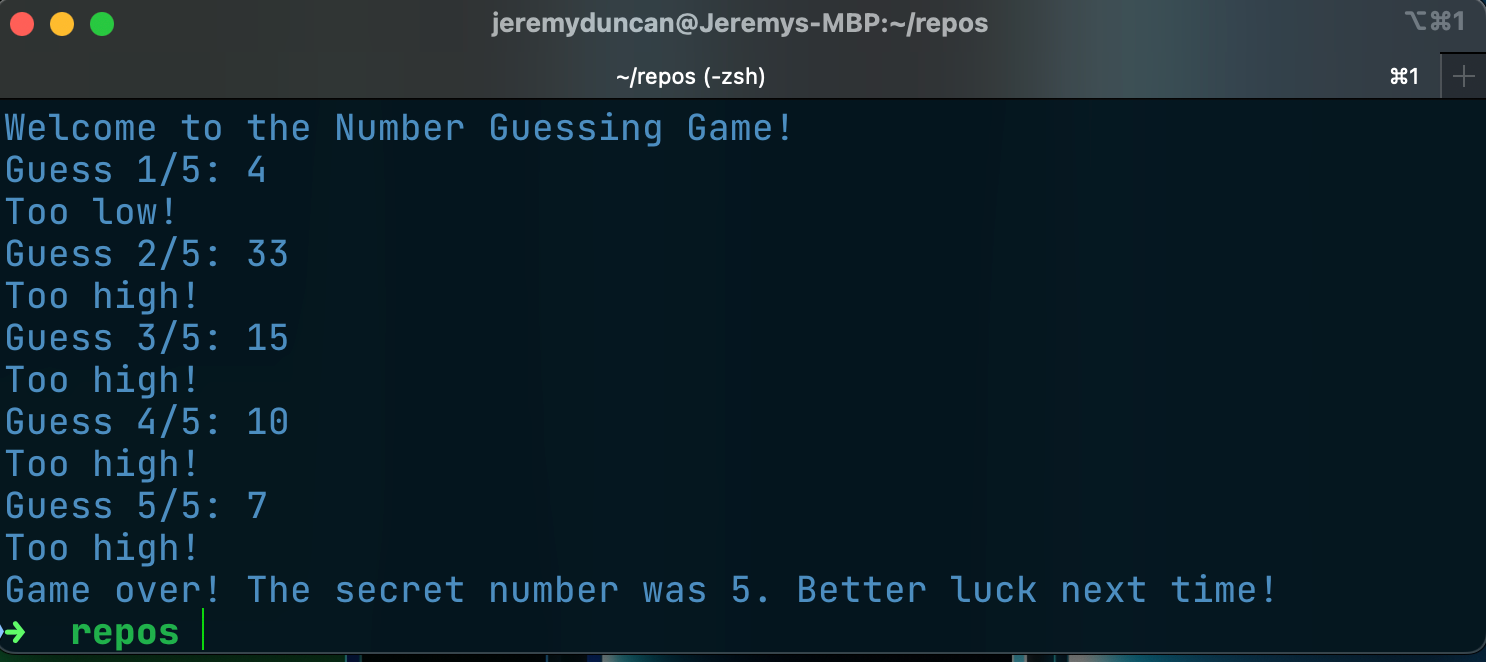
Conclusion
Congratulations! You've just created your first simple game in Ruby. This project introduces you to the basics of Ruby programming and demonstrates how you can use it to build fun, interactive applications.
Feel free to modify the code and experiment with adding new features, such as a scoring system or a hint mechanism.