An Overview of the Slim Templating Language for Ruby on Rails
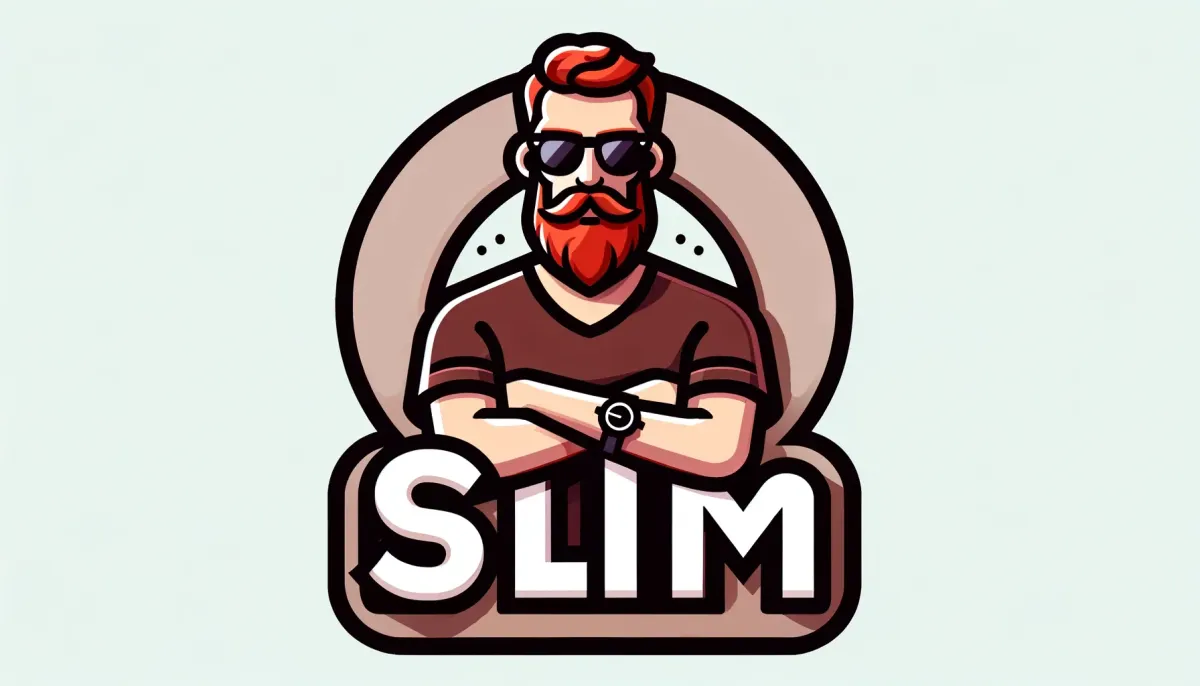
Slim is a lightweight templating language used with Ruby on Rails. It offers a minimalist syntax that reduces the verbosity of traditional HTML templates, providing an alternative to ERB or Haml. Slim’s primary goal is to simplify the writing of HTML, making your templates cleaner and easier to read. In this blog post, we'll delve into Slim's syntax, compare it with other templating languages, and share some examples to demonstrate its simplicity and power.
Slim Syntax Basics
Slim templates use indentation to indicate HTML structure, reducing the need for closing tags and extra markup. The basic structure of a Slim template resembles YAML, with indentation defining the nested relationships between elements. Here's a simple example to illustrate Slim's basic syntax:
doctype html
html
head
title Welcome to Slim
body
h1 Hello, Slim!
p This is a basic Slim template.
This snippet shows a basic HTML structure with a document type, HTML tags, a head section, and a body section. The indentation is used to indicate parent-child relationships, with no need for closing tags like in traditional HTML.
Embedding Ruby Code in Slim
Slim makes it easy to embed Ruby code within your templates. You can insert Ruby code using the equal sign =
to evaluate expressions, while the hyphen -
is used to execute code without output. Here's an example that uses embedded Ruby to loop through an array of items and display them in an unordered list:
ul
- items = ['Apple', 'Banana', 'Cherry']
- items.each do |item|
li = item
This code creates an unordered list with three list items. The Ruby loop iterates through the items
array, and each item is displayed within an li
tag.
Slim Control Structures
Slim also supports control structures such as conditionals and loops. For conditionals, Slim uses the pipe |
to separate the condition from the content to be displayed. Here's an example of a Slim template with a conditional that checks whether a user is logged in:
- user_logged_in = true
- if user_logged_in
p Welcome back!
- else
p Please log in.
This code snippet shows how to use a conditional to determine which content to display based on the value of user_logged_in
.
Benefits of Using Slim
Slim offers several benefits for Ruby on Rails developers:
- Concise Syntax: Slim's minimal syntax reduces boilerplate code, making templates easier to read and maintain.
- Faster Development: The concise syntax allows developers to create templates more quickly, leading to improved productivity.
- Compatibility: Slim is compatible with Rails and can be used with other templating engines like Haml and ERB.
Additional Resources
To learn more about Slim, here are some valuable resources to help you get started:
- Slim Documentation: The official Slim website provides comprehensive documentation on Slim's syntax, features, and best practices.
- Slim GitHub Repository: The GitHub repository for Slim, where you can find the source code and contribute to the project.
- HTML & ERB to Slim Converter: This is a handy tool to do quick conversions from HTML and ERB to Slim. Great for learning as you can see the differences in the code side by side.
- Ruby on Rails Guides: The official Ruby on Rails guides offer additional information on integrating Slim into Rails projects.
With its concise syntax and flexibility, Slim is a great choice for developers looking to streamline their template creation process.