Advanced jQuery Techniques for Enhanced Web Development
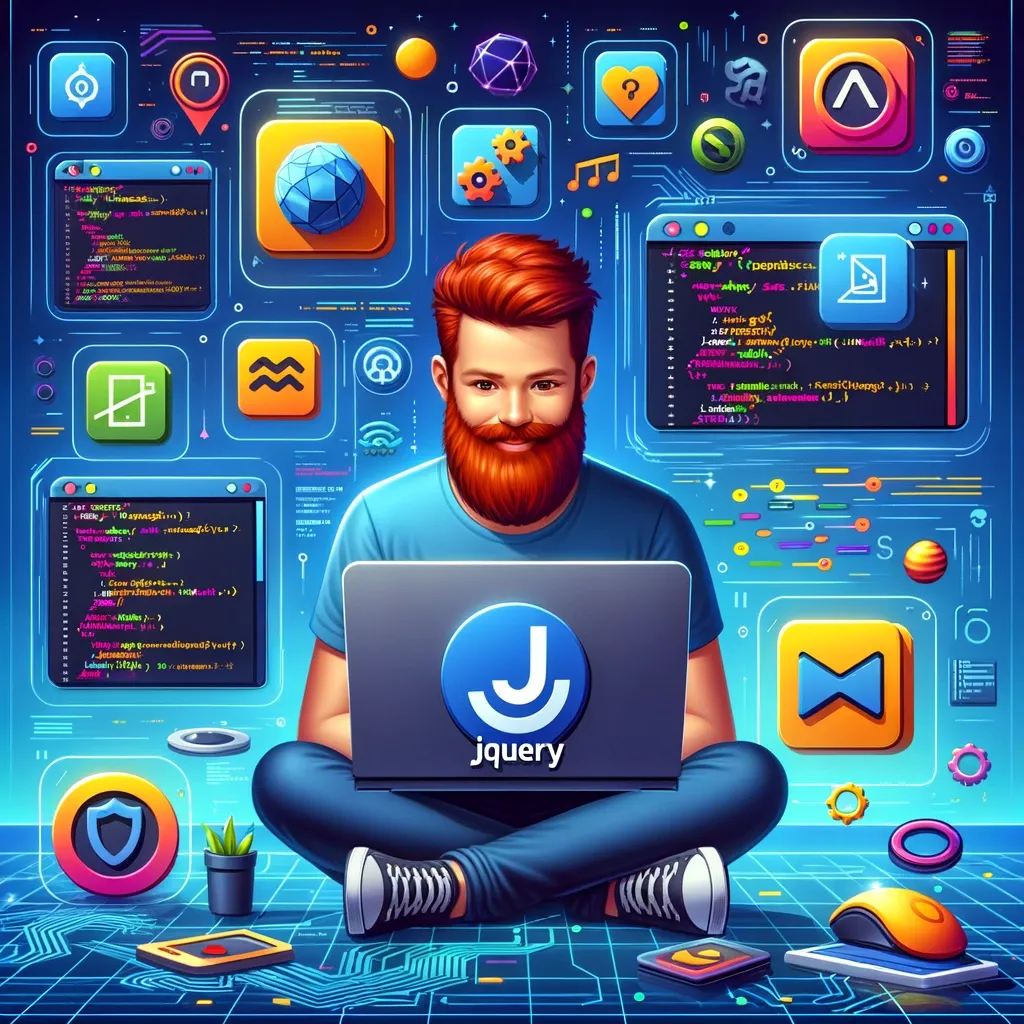
jQuery is packed with many powerful methods that can streamline your web development tasks. Beyond the basics, there are advanced techniques and methods that can significantly enhance your projects. In this post, we will delve into some of these advanced jQuery methods and practical use cases to showcase how they can be leveraged effectively.
DOM Manipulation
DOM (Document Object Model) manipulation is at the heart of jQuery. It allows developers to dynamically change the content and structure of a webpage without reloading it.
Selecting Elements
Selecting elements is the first step in manipulating the DOM. jQuery provides various methods to select HTML elements, making it easier to perform operations on them.
children()
: Selects direct children of the selected element. This method is useful when you need to select immediate child elements only.
$('#myList').children(); // Select all direct children of #myList
find()
: Finds descendants of the selected element. This method is useful for narrowing down the search to specific child elements.
$('#myDiv').find('p'); // Find all <p> elements within #myDiv
$()
: The primary function for selecting elements. This function accepts a CSS selector and returns a jQuery object containing the matched elements.
$('p'); // Select all <p> elements
$('#myId'); // Select element with id="myId"
$('.myClass'); // Select elements with class="myClass"
Modifying Elements
Modifying elements is essential for dynamically changing the content, attributes, or styles of elements on a webpage. jQuery provides several methods to facilitate this.
append()
and prepend()
: Insert content inside an element.
$('#myDiv').append('<p>Appended content</p>'); // Append content
$('#myDiv').prepend('<p>Prepended content</p>'); // Prepend content
addClass()
and removeClass()
: Add or remove classes.
$('p').addClass('newClass'); // Add class
$('p').removeClass('oldClass'); // Remove class
css()
: Get or set CSS properties of an element.
$('p').css('color', 'blue'); // Set the color to blue
attr()
: Get or set attributes of an element.
$('img').attr('src'); // Get the 'src' attribute of an <img>
$('img').attr('src', 'new-image.jpg'); // Set the 'src' attribute
text()
: Get or set the text content of an element.
$('#myDiv').text(); // Get text content
$('#myDiv').text('New text content'); // Set text content
html()
: Get or set the HTML content of an element.
$('#myDiv').html(); // Get HTML content
$('#myDiv').html('<p>New content</p>'); // Set HTML content
Event Handling
Handling events is crucial for creating interactive web applications. jQuery makes it easy to attach, remove, and manage event handlers.
hover()
: Attach handlers for mouseenter and mouseleave events. This method is useful for creating interactive hover effects.
$('#myElement').hover(
function() { $(this).addClass('hover'); },
function() { $(this).removeClass('hover'); }
);
click()
: Attach a click event handler. This is a shorthand method for on('click', handler)
.
$('#myButton').click(function() {
alert('Button clicked!');
});
off()
: Remove an event handler. This method is useful for detaching event handlers when they are no longer needed.
$('#myButton').off('click'); // Remove click event handler
on()
: Attach an event handler. This method is versatile and can be used for various events like click, hover, keypress, etc.
$('#myButton').on('click', function() {
alert('Button clicked!');
});
Effects and Animations
jQuery provides a range of methods for creating effects and animations, making it easy to enhance the visual experience of your web applications.
animate()
: Perform custom animations. This method allows for complex animations by changing multiple CSS properties.
$('#myElement').animate({ left: '250px' }, 'slow');
slideDown()
and slideUp()
: Slide elements up or down.
$('#myElement').slideDown('fast'); // Slide down element
$('#myElement').slideUp('fast'); // Slide up element
fadeIn()
and fadeOut()
: Fade in or out elements.
$('#myElement').fadeIn('slow'); // Fade in element
$('#myElement').fadeOut('slow'); // Fade out element
hide()
and show()
: Hide or show elements.
$('#myElement').hide(); // Hide element
$('#myElement').show(); // Show element
AJAX
AJAX (Asynchronous JavaScript and XML) allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. This means that parts of a web page can be updated without reloading the whole page.
$.get()
and $.post()
: Simplified AJAX GET and POST requests. These methods provide an easier way to make GET and POST requests.
$.get('https://api.example.com/data', function(data) {
console.log(data);
});
$.post('https://api.example.com/data', { key: 'value' }, function(data) {
console.log(data);
});
$.ajax()
: Perform an asynchronous HTTP request. This method is highly configurable and can handle various types of requests.
$.ajax({
url: 'https://api.example.com/data',
method: 'GET',
success: function(data) {
console.log(data);
},
error: function(error) {
console.log('Error:', error);
}
});
Utilities
jQuery offers several utility methods that can help simplify your code and improve its efficiency.
$.extend()
: Merge the contents of two or more objects. This method is useful for combining settings or data objects.
var object1 = { a: 1 };
var object2 = { b: 2 };
var result = $.extend({}, object1, object2);
console.log(result); // Output: { a: 1, b: 2 }
$.each()
: Iterate over a jQuery object or an array. This method is useful for looping through elements or data structures.
$.each([1, 2, 3], function(index, value) {
console.log(index + ': ' + value);
});
Additional Useful jQuery Methods
toggle()
The toggle()
method is used to switch between hiding and showing an element. This is particularly useful for creating interactive elements like dropdowns or accordions.
$('#myButton').click(function() {
$('#myElement').toggle();
});
serialize()
The serialize()
method serializes a form's data into a query string. This is useful for sending form data via AJAX without manually constructing the data string.
$('#myForm').submit(function(event) {
event.preventDefault();
var formData = $(this).serialize();
$.post('/submit', formData, function(response) {
console.log('Form submitted successfully');
});
});
data()
The data()
method is used to store and retrieve data associated with DOM elements. This is helpful for managing element-specific data without cluttering the DOM with custom attributes.
$('#myElement').data('key', 'value'); // Store data
var value = $('#myElement').data('key'); // Retrieve data
console.log(value); // Output: value
$.proxy()
The $.proxy()
method allows you to change the context (this
value) of a function. This is useful when you need to ensure that a function runs in a specific context.
function showMessage() {
console.log(this.message);
}
var context = { message: 'Hello, world!' };
$('#myButton').click($.proxy(showMessage, context));
Practical Use Cases
Dynamic Content Loading
jQuery can be used to load content dynamically into a page without requiring a full page reload. This improves user experience by providing faster and more seamless interactions.
$('#loadContentButton').click(function() {
$('#content').load('/path/to/content.html', function(response, status, xhr) {
if (status == 'error') {
$('#content').html('An error occurred while loading the content.');
}
});
});
Form Validation
With jQuery, you can easily add client-side validation to forms, providing instant feedback to users and reducing server load.
$('#myForm').submit(function(event) {
var isValid = true;
if ($('#name').val().trim() === '') {
isValid = false;
$('#nameError').text('Name is required.');
} else {
$('#nameError').text('');
}
if (!isValid) {
event.preventDefault();
}
});
Smooth Scrolling
jQuery can create smooth scrolling effects for anchor links, enhancing the user experience on single-page applications or sections within a page.
$('a[href^="#"]').click(function(event) {
event.preventDefault();
var target = $(this.hash);
$('html, body').animate({
scrollTop: target.offset().top
}, 1000);
});
Toggle Class
The toggleClass()
method is useful for adding or removing a class from an element based on its current state. This is particularly handy for implementing interactive UI components.
$('#myButton').click(function() {
$('#myElement').toggleClass('active');
});
Debouncing
Debouncing ensures that a function is not called too frequently. This is particularly useful for handling events like window resizing or scrolling.
function resizeHandler() {
console.log('Window resized');
}
var debouncedResizeHandler = $.debounce(250, resizeHandler);
$(window).resize(debouncedResizeHandler);
Conclusion
jQuery offers a plethora of methods that extend far beyond basic DOM manipulation and event handling. By leveraging these advanced techniques and understanding practical use cases, you can create more dynamic, efficient, and user-friendly web applications. Whether you're dynamically loading content, validating forms, or enhancing user interactions with animations and smooth scrolling, jQuery remains a powerful tool in the modern web developer's toolkit.